


How to jump to the order details page when the WeChat mini program gesture returns?
WeChat Mini Program Gesture Back to Order Details Page
This question addresses how to navigate to an order details page using a gesture back action within a WeChat Mini Program. Unfortunately, directly using a gesture back action to initiate navigation to a specific page isn't inherently supported by the WeChat Mini Program framework. The gesture back action is designed to navigate back through the navigation stack, returning to the previously viewed page. To achieve the desired behavior, you need to design your application flow to leverage this functionality. This means that the order details page must be reachable through a standard navigation process (e.g., using wx.navigateTo
). If you want a user to reach the order details page using a gesture back, you'll need to ensure the order details page was the previous page in the navigation stack.
Preventing Gesture Back from Navigating Away from the Order Details Page
To prevent the gesture back from navigating away from the order details page, you need to intercept the back gesture event and handle it accordingly. This can be achieved using the onBackPress
lifecycle method within your order details page's JavaScript file. This method is called when a back gesture is detected. Inside this method, you can choose to either prevent the default back navigation behavior or perform some custom action.
Here's how you can implement it:
Page({ onBackPress() { // Perform some action before allowing the back navigation (e.g., show a confirmation dialog) wx.showModal({ title: 'Confirm', content: 'Are you sure you want to leave this page?', success: (res) => { if (res.confirm) { // User confirmed, allow back navigation return true; // Allow default back navigation } else { // User canceled, prevent back navigation return false; // Prevent default back navigation } } }); } });
This code presents a confirmation dialog to the user. If the user confirms, the default back navigation is allowed; otherwise, it's prevented, keeping the user on the order details page. You could replace the wx.showModal
with other logic, such as saving unsaved changes or performing other necessary actions before allowing the navigation.
Best Practice for Handling Gesture Back Navigation to the Order Details Page
The best practice is to avoid relying solely on gesture back navigation to reach the order details page. Instead, structure your application's navigation flow logically using standard navigation methods (wx.navigateTo
, wx.redirectTo
, wx.navigateBack
). Ensure that the order details page is consistently reached through explicit navigation actions initiated by the user (e.g., tapping on an order in a list). Using the onBackPress
method, as described above, provides a mechanism to handle unexpected back gestures and prevent unintended navigation. This approach improves user experience and maintainability.
Using a Custom Navigation Method Instead of Default Gesture Back
While you can't directly replace the default gesture back behavior with a completely custom animation, you can achieve a similar effect by using custom transitions within your navigation methods. Instead of relying on the default transition provided by wx.navigateTo
, you can create your own custom transitions using animation libraries or techniques provided by the WeChat Mini Program framework. However, this won't replace the gesture back functionality; it will only modify the visual transition when navigating to the order details page, not the back navigation itself. The onBackPress
method will still control the back gesture. This custom transition would be applied when initially navigating to the order details page, not when using the back gesture. Remember to handle the back gesture using onBackPress
for a consistent user experience.
The above is the detailed content of How to jump to the order details page when the WeChat mini program gesture returns?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


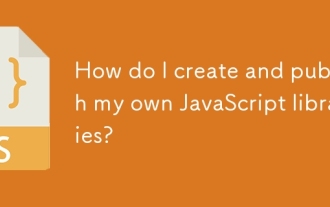
Article discusses creating, publishing, and maintaining JavaScript libraries, focusing on planning, development, testing, documentation, and promotion strategies.
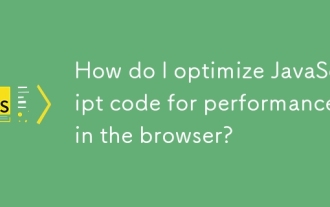
The article discusses strategies for optimizing JavaScript performance in browsers, focusing on reducing execution time and minimizing impact on page load speed.
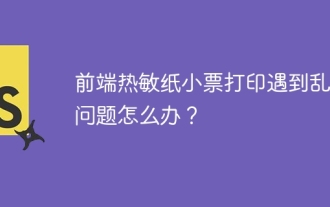
Frequently Asked Questions and Solutions for Front-end Thermal Paper Ticket Printing In Front-end Development, Ticket Printing is a common requirement. However, many developers are implementing...
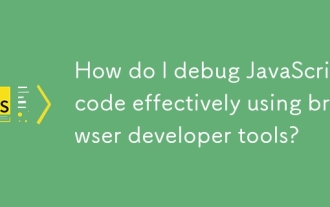
The article discusses effective JavaScript debugging using browser developer tools, focusing on setting breakpoints, using the console, and analyzing performance.
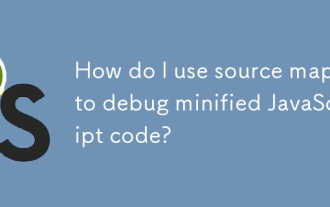
The article explains how to use source maps to debug minified JavaScript by mapping it back to the original code. It discusses enabling source maps, setting breakpoints, and using tools like Chrome DevTools and Webpack.
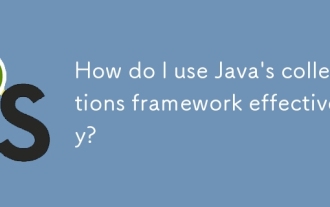
This article explores effective use of Java's Collections Framework. It emphasizes choosing appropriate collections (List, Set, Map, Queue) based on data structure, performance needs, and thread safety. Optimizing collection usage through efficient
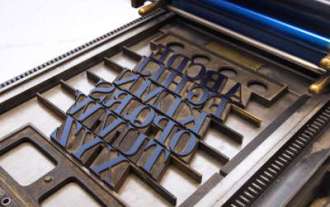
Once you have mastered the entry-level TypeScript tutorial, you should be able to write your own code in an IDE that supports TypeScript and compile it into JavaScript. This tutorial will dive into various data types in TypeScript. JavaScript has seven data types: Null, Undefined, Boolean, Number, String, Symbol (introduced by ES6) and Object. TypeScript defines more types on this basis, and this tutorial will cover all of them in detail. Null data type Like JavaScript, null in TypeScript

This tutorial will explain how to create pie, ring, and bubble charts using Chart.js. Previously, we have learned four chart types of Chart.js: line chart and bar chart (tutorial 2), as well as radar chart and polar region chart (tutorial 3). Create pie and ring charts Pie charts and ring charts are ideal for showing the proportions of a whole that is divided into different parts. For example, a pie chart can be used to show the percentage of male lions, female lions and young lions in a safari, or the percentage of votes that different candidates receive in the election. Pie charts are only suitable for comparing single parameters or datasets. It should be noted that the pie chart cannot draw entities with zero value because the angle of the fan in the pie chart depends on the numerical size of the data point. This means any entity with zero proportion
