Can export default in Vue export strings?
Can Vue's export default
Export a String?
Yes, Vue's export default
can export a string. While it's not the typical usage, export default
is designed to export a single value from a module, and that value can be of any data type, including a string. For example:
// myString.js export default 'Hello from myString.js';
This code creates a module that exports the string "Hello from myString.js". You can then import and use this string in other modules:
// MyComponent.vue import myString from './myString.js'; export default { data() { return { message: myString }; }, template: ` <div> <p>{{ message }}</p> </div> ` };
In this example, the MyComponent
uses the imported string myString
to display "Hello from myString.js" in its template.
Can I Export a String Literal Using export default
in a Vue Component?
Yes, you absolutely can export a string literal using export default
in a Vue component. However, it's crucial to understand that this string will replace the entire component definition. The component itself will not be rendered. Instead, the string will be the exported value.
Consider this example:
// MyComponent.vue export default 'This is my string literal';
If you try to use this in another component like this:
// AnotherComponent.vue import MyComponent from './MyComponent.vue'; export default { data() { return { message: MyComponent }; }, template: ` <div> <p>{{ message }}</p> </div> ` };
AnotherComponent
will display "This is my string literal", not a rendered component. This is because export default
has been overridden with the string.
What are the Implications of Exporting a String Using export default
in a Vue.js Project?
Exporting a string using export default
in a Vue.js project has significant implications, primarily related to the intended use of components. The main implication is that you're losing the core functionality of a Vue component: rendering a UI element. It effectively transforms your .vue
file into a simple JavaScript module exporting a string.
This can lead to confusion and maintenance issues. If you intend to use the .vue
file as a component, exporting a string using export default
will prevent that. Furthermore, code relying on the expected component structure will break. It's generally considered an anti-pattern unless you have a very specific reason to replace the component's standard export with a string.
Is There a Preferred Method for Exporting Simple Data Types Like Strings in Vue Components Besides export default
?
Yes, there are better ways to export simple data types like strings in Vue components than using export default
. Instead of replacing the component's export, you should export the string as a named export within the component's setup
or options
API:
Using the Composition API (setup
):
// MyComponent.vue export const myString = 'This is my string'; export default { setup() { return { myString }; }, // ... rest of your component };
Using the Options API:
// myString.js export default 'Hello from myString.js';
This approach keeps the string separate from the component definition, allowing you to use both the component and the string independently. This is cleaner, more organized, and avoids the potential pitfalls of using export default
for simple data types. This is the preferred method for exporting simple data types alongside your Vue components.
The above is the detailed content of Can export default in Vue export strings?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
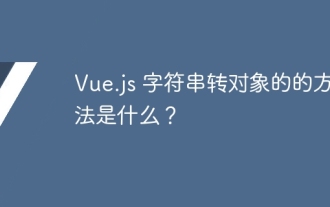
Using JSON.parse() string to object is the safest and most efficient: make sure that strings comply with JSON specifications and avoid common errors. Use try...catch to handle exceptions to improve code robustness. Avoid using the eval() method, which has security risks. For huge JSON strings, chunked parsing or asynchronous parsing can be considered for optimizing performance.

Vue.js is suitable for small and medium-sized projects and fast iterations, while React is suitable for large and complex applications. 1) Vue.js is easy to use and is suitable for situations where the team is insufficient or the project scale is small. 2) React has a richer ecosystem and is suitable for projects with high performance and complex functional needs.
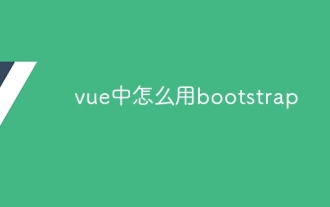
Using Bootstrap in Vue.js is divided into five steps: Install Bootstrap. Import Bootstrap in main.js. Use the Bootstrap component directly in the template. Optional: Custom style. Optional: Use plug-ins.

Vue.js is not difficult to learn, especially for developers with a JavaScript foundation. 1) Its progressive design and responsive system simplify the development process. 2) Component-based development makes code management more efficient. 3) The usage examples show basic and advanced usage. 4) Common errors can be debugged through VueDevtools. 5) Performance optimization and best practices, such as using v-if/v-show and key attributes, can improve application efficiency.
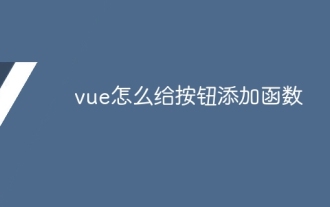
You can add a function to the Vue button by binding the button in the HTML template to a method. Define the method and write function logic in the Vue instance.

Vue.js is mainly used for front-end development. 1) It is a lightweight and flexible JavaScript framework focused on building user interfaces and single-page applications. 2) The core of Vue.js is its responsive data system, and the view is automatically updated when the data changes. 3) It supports component development, and the UI can be split into independent and reusable components.
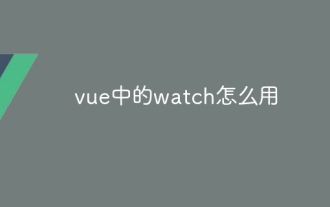
The watch option in Vue.js allows developers to listen for changes in specific data. When the data changes, watch triggers a callback function to perform update views or other tasks. Its configuration options include immediate, which specifies whether to execute a callback immediately, and deep, which specifies whether to recursively listen to changes to objects or arrays.
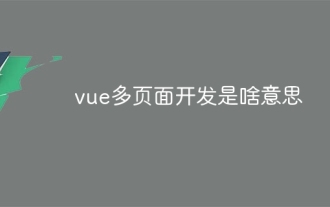
Vue multi-page development is a way to build applications using the Vue.js framework, where the application is divided into separate pages: Code Maintenance: Splitting the application into multiple pages can make the code easier to manage and maintain. Modularity: Each page can be used as a separate module for easy reuse and replacement. Simple routing: Navigation between pages can be managed through simple routing configuration. SEO Optimization: Each page has its own URL, which helps SEO.
