How to configure the lifecycle hooks of the component in Vue
Understanding export default
and Lifecycle Hooks in Vue.js
This article addresses common questions regarding the use of export default
and lifecycle hooks in Vue.js components.
Vue中export default如何配置组件的lifecycle hooks
export default
in Vue.js is a syntax used to export a single component from a .vue
file or a JavaScript module. It doesn't directly configure lifecycle hooks; rather, it's the way you export the component containing the lifecycle hooks. Lifecycle hooks are defined as methods within the component's options object. The export default
statement simply makes this component available for import in other parts of your application.
For example:
<script> export default { name: 'MyComponent', data() { return { message: 'Hello!' }; }, created() { console.log('Component created!'); }, mounted() { console.log('Component mounted!'); }, beforeUpdate() { console.log('Component before update!'); }, updated() { console.log('Component updated!'); }, beforeDestroy() { console.log('Component before destroy!'); }, destroyed() { console.log('Component destroyed!'); } }; </script>
In this example, the lifecycle hooks (created
, mounted
, etc.) are defined as methods within the object exported using export default
. The export default
statement itself doesn't change how these hooks are defined or function.
How can I access and utilize lifecycle hooks within a Vue component exported using export default
?
Accessing and utilizing lifecycle hooks within a component exported using export default
is straightforward. You define them as methods within the options object of your component, as shown in the previous example. Each hook is called at a specific point in the component's lifecycle, allowing you to perform actions like fetching data (created
), manipulating the DOM (mounted
), or cleaning up resources (beforeDestroy
).
Remember that the lifecycle hook names are specific and must be used correctly. Misspelling a hook name will prevent it from being called. Also, the context (this
) within a lifecycle hook refers to the component instance, allowing you to access data, methods, and computed properties.
What are the best practices for organizing lifecycle hook methods when using export default
in Vue components?
Organizing lifecycle hook methods effectively enhances readability and maintainability. Here are some best practices:
- Keep it concise: Avoid putting too much logic within a single hook. Break down complex tasks into smaller, more manageable methods called from within the hook.
- Group related logic: If you have multiple hooks performing similar tasks (e.g., data fetching and processing), consider structuring your code to group related actions.
- Use comments: Add clear and concise comments to explain the purpose of each hook and the actions performed within it.
- Follow a consistent naming convention: Maintain consistency in your naming style for methods and variables.
- Consider using mixins: For reusable lifecycle hook logic across multiple components, consider using Vue mixins. This promotes code reusability and reduces redundancy.
Does using export default
affect the way lifecycle hooks are defined and called in a Vue component?
No, using export default
does not affect how lifecycle hooks are defined and called. export default
is simply a mechanism for exporting the component; the lifecycle hooks are still defined and invoked according to their standard behavior within the Vue.js lifecycle. The component's structure and how its lifecycle hooks operate remain unchanged regardless of whether you use export default
or named exports. The only difference is how you import and use the component in other parts of your application.
The above is the detailed content of How to configure the lifecycle hooks of the component in Vue. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


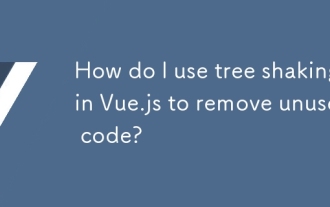
The article discusses using tree shaking in Vue.js to remove unused code, detailing setup with ES6 modules, Webpack configuration, and best practices for effective implementation.Character count: 159
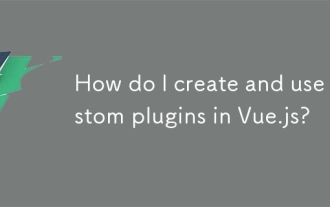
Article discusses creating and using custom Vue.js plugins, including development, integration, and maintenance best practices.

Vue.js is mainly used for front-end development. 1) It is a lightweight and flexible JavaScript framework focused on building user interfaces and single-page applications. 2) The core of Vue.js is its responsive data system, and the view is automatically updated when the data changes. 3) It supports component development, and the UI can be split into independent and reusable components.
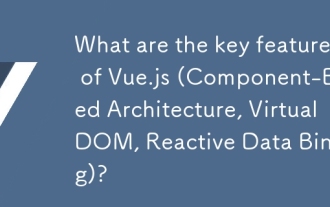
Vue.js enhances web development with its Component-Based Architecture, Virtual DOM for performance, and Reactive Data Binding for real-time UI updates.
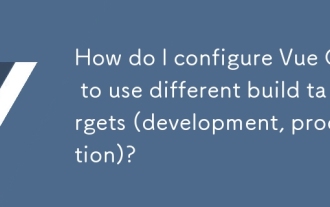
The article explains how to configure Vue CLI for different build targets, switch environments, optimize production builds, and ensure source maps in development for debugging.

The article discusses using Vue with Docker for deployment, focusing on setup, optimization, management, and performance monitoring of Vue applications in containers.
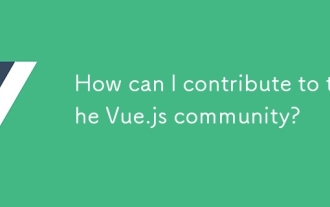
The article discusses various ways to contribute to the Vue.js community, including improving documentation, answering questions, coding, creating content, organizing events, and financial support. It also covers getting involved in open-source proje

Vue.js is not difficult to learn, especially for developers with a JavaScript foundation. 1) Its progressive design and responsive system simplify the development process. 2) Component-based development makes code management more efficient. 3) The usage examples show basic and advanced usage. 4) Common errors can be debugged through VueDevtools. 5) Performance optimization and best practices, such as using v-if/v-show and key attributes, can improve application efficiency.
