How to handle foreign key constraints in SQL delete rows
Deleting Rows with Foreign Key Constraints
This is a common problem in relational database management. Foreign key constraints are designed to maintain data integrity by ensuring referential consistency between tables. When you try to delete a row in a parent table that has corresponding rows in a child table referencing it via a foreign key, the database will usually prevent the deletion and throw an error. This is because deleting the parent row would leave the child rows with dangling references, violating the constraint and potentially leading to data corruption or inconsistencies. The simplest and most recommended approach is to first delete the related rows in the child table(s) before deleting the row in the parent table.
For example, consider a Customers
table (parent) and an Orders
table (child) with a foreign key relationship linking Orders.CustomerID
to Customers.CustomerID
. If you try to delete a customer who has placed orders, the database will prevent the deletion. The correct approach is to first delete all orders associated with that customer from the Orders
table, and only then delete the customer from the Customers
table. This can be achieved using the following SQL statements (assuming your database system supports cascading deletes - see below for alternatives):
DELETE FROM Orders WHERE CustomerID = <customer_id>; DELETE FROM Customers WHERE CustomerID = <customer_id>;
Replacing <customer_id>
with the actual customer ID.
Bypassing Foreign Key Constraints
While generally discouraged, there are ways to bypass foreign key constraints, but it should only be done under very controlled circumstances and with a complete understanding of the potential risks. Directly bypassing these constraints can lead to data inconsistencies and corruption. The methods for bypassing depend on your specific database system.
- Temporary disabling constraints: Some database systems allow you to temporarily disable foreign key constraints before deleting the rows and then re-enabling them afterward. This is generally risky and should be avoided unless absolutely necessary. It requires careful planning and execution to ensure data integrity is restored after the operation. The syntax varies across different database systems (e.g.,
ALTER TABLE ... DISABLE CONSTRAINT
in some systems). - Using transactions: Enclosing the delete operations within a transaction allows for rollback in case of errors. This minimizes the risk of leaving the database in an inconsistent state. However, this does not actually bypass the constraint, it simply allows for a controlled failure mechanism.
- Using
ON DELETE CASCADE
(Recommended Approach when applicable): This is the best way to manage the relationship if it is appropriate for your data model. This clause allows you to define the behavior when a parent row is deleted.ON DELETE CASCADE
will automatically delete all corresponding child rows when a parent row is deleted. This is safer than bypassing the constraint because it maintains data integrity in a controlled manner. This should be part of the table's initial design rather than a solution applied retroactively.
DELETE FROM Orders WHERE CustomerID = <customer_id>; DELETE FROM Customers WHERE CustomerID = <customer_id>;
Handling Foreign Key Constraint Violations
There are several ways to handle foreign key constraint violations during data deletion:
-
Cascading Deletes (
ON DELETE CASCADE
): As explained above, this is the preferred approach if appropriate for your data model. It automatically deletes related child rows. -
Restricting Deletes (
ON DELETE RESTRICT
): This is the default behavior in most database systems. It prevents deletion of a parent row if there are related child rows. This enforces referential integrity but requires manual cleanup of related rows in child tables before deleting parent rows. -
Setting Nulls (
ON DELETE SET NULL
): This sets the foreign key column in the child table toNULL
when the corresponding parent row is deleted. This is only suitable ifNULL
is a valid value for the foreign key column. -
No Action (
ON DELETE NO ACTION
): Similar toON DELETE RESTRICT
, this prevents the deletion if there are related child rows. However, it may differ slightly depending on the database system in terms of the specific timing of constraint checks. - Error Handling: Implementing proper error handling in your application code is crucial. Your application should gracefully handle the constraint violation error and provide informative messages to the user. This might involve prompting the user to delete related child rows first or providing alternative actions.
Best Practices for Deleting Rows with Foreign Key Constraints
- Always plan your deletions carefully: Understand the relationships between your tables and the impact of deleting data.
-
Use
ON DELETE CASCADE
where appropriate: This simplifies the process and ensures data consistency. However, carefully consider the implications before using this approach. It's best used when the logical relationship dictates that deleting the parent should automatically delete the children. -
Delete child rows first: If
ON DELETE CASCADE
isn't in place, always delete related child rows before deleting parent rows. - Use transactions: Enclose your delete operations within a transaction to ensure atomicity. This ensures that either all deletions succeed or none do, preventing inconsistencies.
- Implement error handling: Handle potential constraint violations gracefully in your application code.
- Regularly review your database schema: Ensure your foreign key constraints accurately reflect the relationships in your data.
By following these best practices, you can effectively manage foreign key constraints and prevent data inconsistencies when deleting rows in your SQL database. Always prioritize data integrity and avoid bypassing constraints unless absolutely necessary and under strict control.
The above is the detailed content of How to handle foreign key constraints in SQL delete rows. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


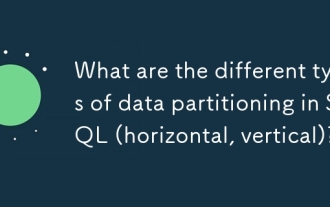
The article discusses horizontal and vertical data partitioning in SQL, focusing on their impact on performance and scalability. It compares benefits and considerations for choosing between them.
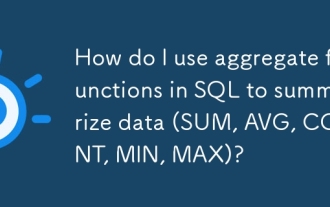
The article explains how to use SQL aggregate functions (SUM, AVG, COUNT, MIN, MAX) to summarize data, detailing their uses and differences, and how to combine them in queries.Character count: 159
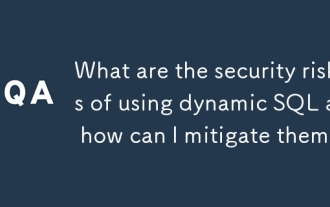
The article discusses security risks of dynamic SQL, focusing on SQL injection, and provides mitigation strategies like using parameterized queries and input validation.
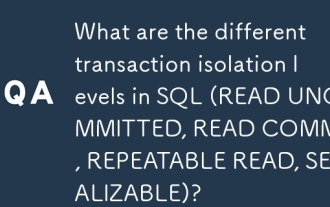
The article discusses SQL transaction isolation levels: READ UNCOMMITTED, READ COMMITTED, REPEATABLE READ, and SERIALIZABLE. It examines their impact on data consistency and performance, noting that higher isolation ensures greater consistency but ma
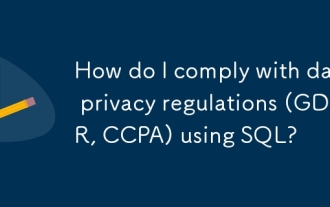
Article discusses using SQL for GDPR and CCPA compliance, focusing on data anonymization, access requests, and automatic deletion of outdated data.(159 characters)
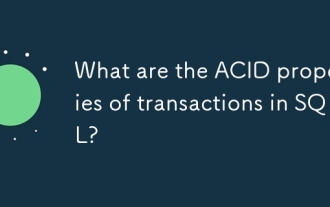
The article discusses the ACID properties (Atomicity, Consistency, Isolation, Durability) in SQL transactions, crucial for maintaining data integrity and reliability.
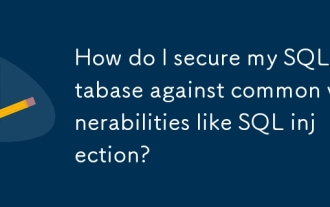
The article discusses securing SQL databases against vulnerabilities like SQL injection, emphasizing prepared statements, input validation, and regular updates.
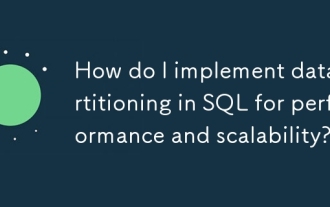
Article discusses implementing data partitioning in SQL for better performance and scalability, detailing methods, best practices, and monitoring tools.
