How to add, delete, modify and check mongodb database
MongoDB CRUD Operations: Inserting, Updating, Deleting, and Querying Data
MongoDB offers a flexible and efficient way to perform Create, Read, Update, and Delete (CRUD) operations. Let's explore how to perform each of these actions.
Inserting Data:
Inserting documents into a MongoDB collection is straightforward. You can use the insertOne()
method to insert a single document or insertMany()
to insert multiple documents. Here's an example using the MongoDB shell:
// Insert a single document db.myCollection.insertOne( { name: "John Doe", age: 30, city: "New York" } ); // Insert multiple documents db.myCollection.insertMany( [ { name: "Jane Doe", age: 25, city: "London" }, { name: "Peter Jones", age: 40, city: "Paris" } ] );
Drivers like Node.js or Python offer similar methods, often with added features for error handling and asynchronous operations. For example, in Node.js using the MongoDB driver:
const { MongoClient } = require('mongodb'); const uri = "mongodb://localhost:27017"; // Replace with your connection string const client = new MongoClient(uri); async function run() { try { await client.connect(); const database = client.db('myDatabase'); const collection = database.collection('myCollection'); const doc = { name: "Alice", age: 28, city: "Tokyo" }; const result = await collection.insertOne(doc); console.log(`A document was inserted with the _id: ${result.insertedId}`); } finally { await client.close(); } } run().catch(console.dir);
Updating Data:
MongoDB provides several ways to update documents. updateOne()
updates a single document matching a query, while updateMany()
updates multiple documents. You use the $set
operator to modify fields within a document. Here's an example using the MongoDB shell:
// Update a single document db.myCollection.updateOne( { name: "John Doe" }, { $set: { age: 31 } } ); // Update multiple documents db.myCollection.updateMany( { age: { $lt: 30 } }, { $set: { city: "Unknown" } } );
Similar updateOne()
and updateMany()
methods exist in various drivers.
Deleting Data:
Deleting documents involves using deleteOne()
to remove a single matching document and deleteMany()
to remove multiple matching documents.
// Delete a single document db.myCollection.deleteOne( { name: "Jane Doe" } ); // Delete multiple documents db.myCollection.deleteMany( { city: "Unknown" } );
Again, driver libraries provide equivalent functions.
Querying Data:
Retrieving data from MongoDB is done using the find()
method. This method allows for powerful querying using various operators and conditions.
// Find all documents db.myCollection.find(); // Find documents where age is greater than 30 db.myCollection.find( { age: { $gt: 30 } } ); // Find documents and project specific fields db.myCollection.find( { age: { $gt: 30 } }, { name: 1, age: 1, _id: 0 } ); // _id: 0 excludes the _id field
The find()
method returns a cursor, which you can iterate through to access the individual documents. Drivers provide methods to handle cursors efficiently.
Efficiently Querying Large Datasets in MongoDB
Efficiently querying large datasets in MongoDB requires understanding indexing and query optimization techniques. Indexes are crucial for speeding up queries. Create indexes on frequently queried fields. Use appropriate query operators and avoid using $where
clauses (which are slow). Analyze query execution plans using explain()
to identify bottlenecks and optimize your queries. Consider using aggregation pipelines for complex queries involving multiple stages of processing. Sharding can distribute data across multiple servers for improved scalability and query performance on extremely large datasets.
Best Practices for Ensuring Data Integrity When Performing CRUD Operations in MongoDB
Maintaining data integrity in MongoDB involves several key practices:
- Data Validation: Use schema validation to enforce data types and constraints on your documents. This prevents invalid data from being inserted into your collection.
- Transactions (for MongoDB 4.0 and later): Use multi-document transactions to ensure atomicity when performing multiple CRUD operations within a single logical unit of work. This prevents partial updates or inconsistencies.
- Error Handling: Implement robust error handling in your application code to gracefully manage potential issues during CRUD operations (e.g., network errors, duplicate key errors).
- Auditing: Track changes to your data by logging CRUD operations, including timestamps and user information. This helps with debugging, security auditing, and data recovery.
- Regular Backups: Regularly back up your MongoDB data to protect against data loss due to hardware failure or other unforeseen events.
Differences Between MongoDB's Shell and a Driver for CRUD Operations
The MongoDB shell provides a convenient interactive environment for performing CRUD operations directly against the database. It's great for quick testing and ad-hoc queries. However, for production applications, using a driver (like Node.js, Python, Java, etc.) is essential. Drivers offer:
- Error Handling and Exception Management: Drivers provide built-in mechanisms for handling errors and exceptions, which are crucial for building robust applications. The shell provides less robust error handling.
- Asynchronous Operations: Drivers support asynchronous operations, enabling your application to remain responsive while performing potentially time-consuming database operations. The shell is synchronous.
- Connection Pooling: Drivers manage database connections efficiently through connection pooling, improving performance and resource utilization.
- Integration with Application Frameworks: Drivers integrate seamlessly with various application frameworks and programming languages, simplifying development.
- Security: Drivers often offer enhanced security features like connection encryption and authentication.
While the shell is valuable for learning and experimentation, drivers are necessary for building production-ready applications that require robust error handling, asynchronous operations, and efficient resource management.
The above is the detailed content of How to add, delete, modify and check mongodb database. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










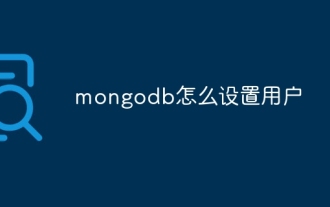
To set up a MongoDB user, follow these steps: 1. Connect to the server and create an administrator user. 2. Create a database to grant users access. 3. Use the createUser command to create a user and specify their role and database access rights. 4. Use the getUsers command to check the created user. 5. Optionally set other permissions or grant users permissions to a specific collection.

The main tools for connecting to MongoDB are: 1. MongoDB Shell, suitable for quickly viewing data and performing simple operations; 2. Programming language drivers (such as PyMongo, MongoDB Java Driver, MongoDB Node.js Driver), suitable for application development, but you need to master the usage methods; 3. GUI tools (such as Robo 3T, Compass) provide a graphical interface for beginners and quick data viewing. When selecting tools, you need to consider application scenarios and technology stacks, and pay attention to connection string configuration, permission management and performance optimization, such as using connection pools and indexes.
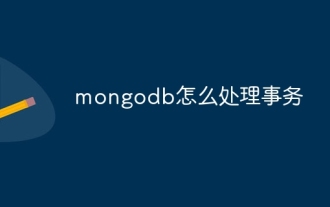
Transaction processing in MongoDB provides solutions such as multi-document transactions, snapshot isolation, and external transaction managers to achieve transaction behavior, ensure multiple operations are executed as one atomic unit, ensuring atomicity and isolation. Suitable for applications that need to ensure data integrity, prevent concurrent operational data corruption, or implement atomic updates in distributed systems. However, its transaction processing capabilities are limited and are only suitable for a single database instance. Multi-document transactions only support read and write operations. Snapshot isolation does not provide atomic guarantees. Integrating external transaction managers may also require additional development work.
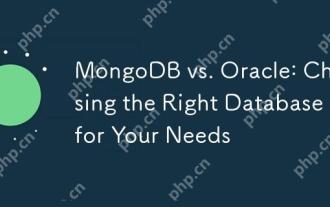
MongoDB is suitable for unstructured data and high scalability requirements, while Oracle is suitable for scenarios that require strict data consistency. 1.MongoDB flexibly stores data in different structures, suitable for social media and the Internet of Things. 2. Oracle structured data model ensures data integrity and is suitable for financial transactions. 3.MongoDB scales horizontally through shards, and Oracle scales vertically through RAC. 4.MongoDB has low maintenance costs, while Oracle has high maintenance costs but is fully supported.
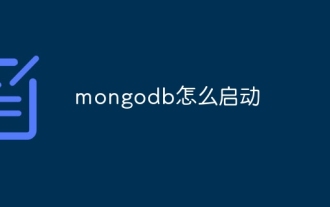
To start the MongoDB server: On a Unix system, run the mongod command. On Windows, run the mongod.exe command. Optional: Set the configuration using the --dbpath, --port, --auth, or --replSet options. Use the mongo command to verify that the connection is successful.

MongoDB is more suitable for processing unstructured data and rapid iteration, while Oracle is more suitable for scenarios that require strict data consistency and complex queries. 1.MongoDB's document model is flexible and suitable for handling complex data structures. 2. Oracle's relationship model is strict to ensure data consistency and complex query performance.

Choose MongoDB or Redis according to application requirements: MongoDB is suitable for storing complex data, and Redis is suitable for fast access to key-value pairs and caches. MongoDB uses document data models, provides persistent storage, and horizontal scalability; while Redis uses key values to perform well and cost-effectively. The final choice depends on the specific needs of the application, such as data type, performance requirements, scalability, and reliability.
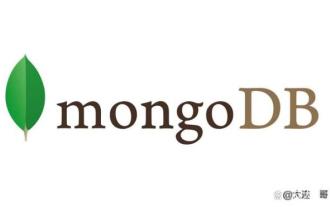
This article explains the advanced MongoDB query skills, the core of which lies in mastering query operators. 1. Use $and, $or, and $not combination conditions; 2. Use $gt, $lt, $gte, and $lte for numerical comparison; 3. $regex is used for regular expression matching; 4. $in and $nin match array elements; 5. $exists determine whether the field exists; 6. $elemMatch query nested documents; 7. Aggregation Pipeline is used for more powerful data processing. Only by proficiently using these operators and techniques and paying attention to index design and performance optimization can you conduct MongoDB data queries efficiently.
