Introduction to Network Programming in Python
This tutorial introduces Python sockets and demonstrates building HTTP servers and clients using the socket
module. It also explores Tornado, a Python networking library ideal for long-polling, WebSockets, and applications needing persistent user connections.
Understanding Sockets
A socket acts as a communication channel between two applications, whether on the same machine or across a network. Essentially, it's a connection link between a server and a client; the server provides information requested by the client. For example, your browser uses a socket to connect to a web server when you visit a webpage.
The socket
Module
Socket creation uses the socket.socket()
function:
import socket s = socket.socket(socket_family, socket_type, protocol=0)
Arguments:
-
socket_family
: Address family (e.g.,socket.AF_INET
for IPv4,socket.AF_INET6
for IPv6). -
socket_type
: Socket type (e.g.,socket.SOCK_STREAM
for TCP,socket.SOCK_DGRAM
for UDP). -
protocol
: Usually defaults to 0.
Once you have a socket object, you can build a server or client using its methods.
Creating a Simple Client
Key client methods:
s = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
-
s.connect()
: Establishes a TCP connection.
Example:
import socket stream_socket = socket.socket(socket.AF_INET, socket.SOCK_STREAM) server = "localhost" port = 8080 server_address = (server, port) stream_socket.connect(server_address) message = 'message' stream_socket.sendall(message.encode()) data = stream_socket.recv(10) print(data) stream_socket.close()
Building a Simple Server
Key server methods:
s = socket.socket(socket.AF_INET, socket.SOCK_STREAM)
-
s.bind()
: Assigns an address (hostname, port) to the socket. -
s.listen()
: Starts listening for TCP connections. -
s.accept()
: Accepts a TCP client connection.
Example:
import socket sock = socket.socket(socket.AF_INET, socket.SOCK_STREAM) host = 'localhost' port = 8080 sock.bind((host, port)) sock.listen(1) print('Waiting for a connection') connection, client = sock.accept() print(client, 'connected') data = connection.recv(16) print('Received "%s"' % data) if data: connection.sendall(data) else: print('No data from', client) connection.close()
Run the client and server in separate terminals for communication. Use netstat -ntlp
(or a similar command for your OS) to check port usage.
The Tornado Framework
Tornado is a Python web framework and asynchronous networking library. Its non-blocking I/O handles many concurrent connections, making it suitable for long-polling, WebSockets, and applications requiring persistent connections.
A simple Tornado WebSocket example:
import tornado.ioloop import tornado.web class ApplicationHandler(tornado.web.RequestHandler): def get(self): self.write("""<title>Tornado Framework</title><h2 id="Welcome-to-the-Tornado-framework">Welcome to the Tornado framework</h2>""") if __name__ == "__main__": application = tornado.web.Application([ (r"/", ApplicationHandler), ]) application.listen(5001) tornado.ioloop.IOLoop.instance().start()
Tornado also integrates with asyncio
, enabling the use of both libraries within the same event loop.
Synchronous vs. Asynchronous Programming
Synchronous programming executes tasks sequentially, while asynchronous programming allows concurrent task execution without waiting for others to finish. Asynchronous programming is advantageous when dealing with I/O-bound operations like API calls, preventing delays and improving application responsiveness. Tornado's asynchronous capabilities are particularly useful for handling multiple API requests concurrently.
Conclusion
This tutorial provided a foundation for socket programming in Python and demonstrated simple server/client creation. Further exploration of the socket
module and Tornado will enhance your networking capabilities. Remember to consult the official Python documentation for more detailed information.
(Image of Tornado webserver output - replace with actual image URL if available)
The above is the detailed content of Introduction to Network Programming in Python. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


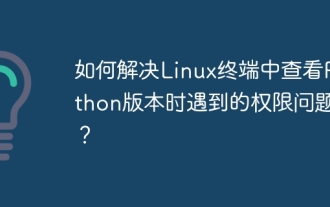
Solution to permission issues when viewing Python version in Linux terminal When you try to view Python version in Linux terminal, enter python...
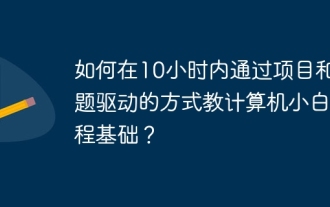
How to teach computer novice programming basics within 10 hours? If you only have 10 hours to teach computer novice some programming knowledge, what would you choose to teach...
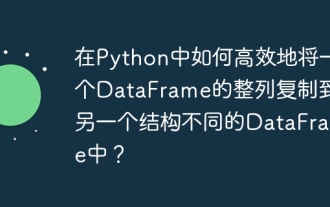
When using Python's pandas library, how to copy whole columns between two DataFrames with different structures is a common problem. Suppose we have two Dats...
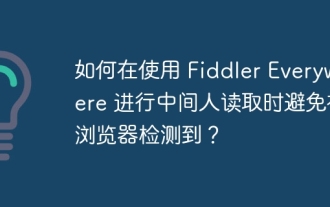
How to avoid being detected when using FiddlerEverywhere for man-in-the-middle readings When you use FiddlerEverywhere...
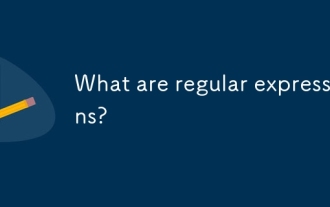
Regular expressions are powerful tools for pattern matching and text manipulation in programming, enhancing efficiency in text processing across various applications.
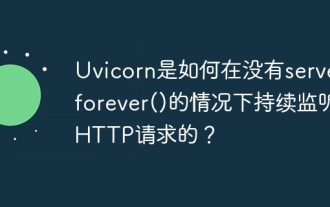
How does Uvicorn continuously listen for HTTP requests? Uvicorn is a lightweight web server based on ASGI. One of its core functions is to listen for HTTP requests and proceed...
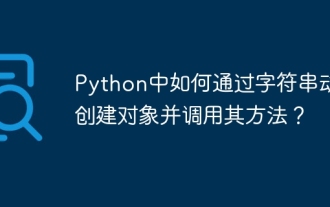
In Python, how to dynamically create an object through a string and call its methods? This is a common programming requirement, especially if it needs to be configured or run...
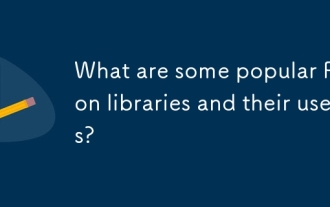
The article discusses popular Python libraries like NumPy, Pandas, Matplotlib, Scikit-learn, TensorFlow, Django, Flask, and Requests, detailing their uses in scientific computing, data analysis, visualization, machine learning, web development, and H
