


Managing Multi-Device Sessions with Laravel's Device Logout Feature
Laravel offers a robust security feature through Auth::logoutOtherDevices() that enables users to terminate their sessions across all devices except the current one. This capability is particularly valuable for maintaining account security in applications handling sensitive data.
You can implement this feature for proactive security measures, like responding to suspicious activities:
<!-- Syntax highlighted by torchlight.dev -->public function secureSessions(Request $request) { Auth::logoutOtherDevices($request->password); return back()->with('status', 'All other device sessions terminated'); }
The implementation requires the auth.session middleware for proper session management:
<!-- Syntax highlighted by torchlight.dev -->Route::middleware(['auth', 'auth.session'])->group(function () { // Protected routes });
Here's a practical implementation for password updates with multi-device logout:
<!-- Syntax highlighted by torchlight.dev -->class SecurityController extends Controller { public function updatePassword(Request $request) { $validated = $request->validate([ 'current_password' => 'required', 'new_password' => 'required|min:8|confirmed' ]); if (!Hash::check($request->current_password, Auth::user()->password)) { return back()->withErrors([ 'current_password' => 'Invalid password provided' ]); } Auth::logoutOtherDevices($request->current_password); Auth::user()->update([ 'password' => Hash::make($request->new_password) ]); return redirect('/dashboard') ->with('status', 'Password updated and other devices logged out'); } }
This approach provides users with greater control over their account security while helping prevent unauthorised access through forgotten active sessions.
The above is the detailed content of Managing Multi-Device Sessions with Laravel's Device Logout Feature. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
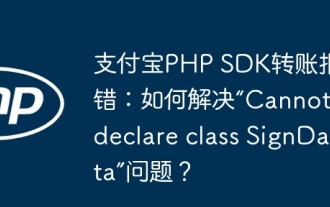
Alipay PHP...
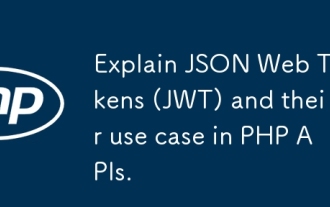
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
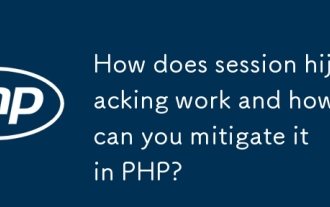
Session hijacking can be achieved through the following steps: 1. Obtain the session ID, 2. Use the session ID, 3. Keep the session active. The methods to prevent session hijacking in PHP include: 1. Use the session_regenerate_id() function to regenerate the session ID, 2. Store session data through the database, 3. Ensure that all session data is transmitted through HTTPS.
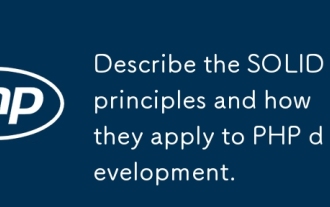
The application of SOLID principle in PHP development includes: 1. Single responsibility principle (SRP): Each class is responsible for only one function. 2. Open and close principle (OCP): Changes are achieved through extension rather than modification. 3. Lisch's Substitution Principle (LSP): Subclasses can replace base classes without affecting program accuracy. 4. Interface isolation principle (ISP): Use fine-grained interfaces to avoid dependencies and unused methods. 5. Dependency inversion principle (DIP): High and low-level modules rely on abstraction and are implemented through dependency injection.
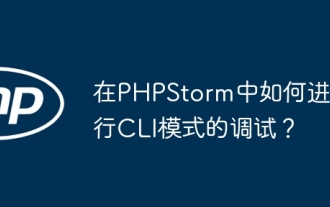
How to debug CLI mode in PHPStorm? When developing with PHPStorm, sometimes we need to debug PHP in command line interface (CLI) mode...
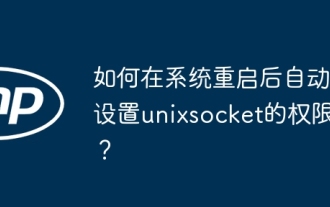
How to automatically set the permissions of unixsocket after the system restarts. Every time the system restarts, we need to execute the following command to modify the permissions of unixsocket: sudo...
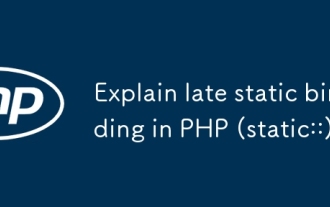
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.
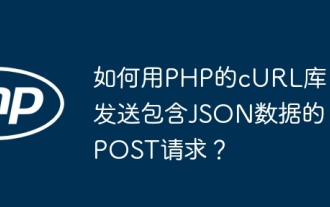
Sending JSON data using PHP's cURL library In PHP development, it is often necessary to interact with external APIs. One of the common ways is to use cURL library to send POST�...
