Codestral API Tutorial: Getting Started With Mistral's API
Codestral: A Comprehensive Guide to the Code Generation API
Codestral, a cutting-edge generative model, excels at code generation tasks like fill-in-the-middle (FIM) and code completion. Trained on over 80 programming languages, it's a versatile tool for developers working with both common and less-used languages. This tutorial details how to effectively utilize the Codestral API. For a broader overview of Codestral, see my article on "What is Mistral's Codestral".
API Endpoints
Codestral offers two primary API endpoints:
-
codestral.mistral.ai
: Ideal for individual users and small-scale projects. Currently free (until August 1, 2024), it will transition to a subscription model. -
api.mistral.ai
: Designed for business needs and high-volume usage, offering increased rate limits and robust support.
Mistral recommends codestral.mistral.ai
for IDE plugins or user-facing tools, allowing users to manage their own API keys. api.mistral.ai
is preferred for other applications due to its higher rate limits and scalability. This tutorial focuses on codestral.mistral.ai
.
Getting Started
Obtaining an API Key:
- Sign up: Create a Mistral AI account.
-
Get your API key: For
api.mistral.ai
, navigate to the API Keys tab and generate a new key. Forcodestral.mistral.ai
, go to the Codestral tab (often marked "New"), complete the signup (note: a phone number is usually required), and access your key once approved.
Authentication (Python):
We'll use the requests
library to create authentication functions for both endpoints:
import requests import json api_key = 'INSERT YOUR API KEY HERE' def call_chat_endpoint(data, api_key=api_key): url = "https://codestral.mistral.ai/v1/chat/completions" #Corrected URL headers = { "Authorization": f"Bearer {api_key}", "Content-Type": "application/json", "Accept": "application/json" } response = requests.post(url, headers=headers, data=json.dumps(data)) return response.json() if response.status_code == 200 else f"Error: {response.status_code}, {response.text}" def call_fim_endpoint(data, api_key=api_key): url = "https://codestral.mistral.ai/v1/fim/completions" #Corrected URL headers = { "Authorization": f"Bearer {api_key}", "Content-Type": "application/json", "Accept": "application/json" } response = requests.post(url, headers=headers, data=json.dumps(data)) return response.json() if response.status_code == 200 else f"Error: {response.status_code}, {response.text}"
Understanding the Endpoints
Fill-in-the-Middle (FIM) Endpoint:
Generates code to fill the gap between a prompt
and an optional suffix
.
-
URL:
https://codestral.mistral.ai/v1/fim/completions
-
Parameters:
prompt
,suffix
(optional),stop
(optional)
Example:
prompt = "def fibonacci(n: int):" suffix = "n = int(input('Enter a number: '))\nprint(fibonacci(n))" data = {"model": "codestral-latest", "prompt": prompt, "suffix": suffix, "temperature": 0} response = call_fim_endpoint(data)
Instruct Endpoint:
Uses instructions to guide code generation.
-
URL:
https://codestral.mistral.ai/v1/chat/completions
-
Parameters:
prompt
,temperature
(optional),max_tokens
(optional)
Example:
import requests import json api_key = 'INSERT YOUR API KEY HERE' def call_chat_endpoint(data, api_key=api_key): url = "https://codestral.mistral.ai/v1/chat/completions" #Corrected URL headers = { "Authorization": f"Bearer {api_key}", "Content-Type": "application/json", "Accept": "application/json" } response = requests.post(url, headers=headers, data=json.dumps(data)) return response.json() if response.status_code == 200 else f"Error: {response.status_code}, {response.text}" def call_fim_endpoint(data, api_key=api_key): url = "https://codestral.mistral.ai/v1/fim/completions" #Corrected URL headers = { "Authorization": f"Bearer {api_key}", "Content-Type": "application/json", "Accept": "application/json" } response = requests.post(url, headers=headers, data=json.dumps(data)) return response.json() if response.status_code == 200 else f"Error: {response.status_code}, {response.text}"
Advanced Usage
-
Rate Limits:
codestral.mistral.ai
has limits of 30 requests per minute and 2000 per day;api.mistral.ai
has 200 requests per second per workspace. Implement retry logic using Python'stime
library to handle rate limits. - Error Handling: Handle common errors (401, 429, 500) using appropriate error codes. Retry logic is beneficial for transient errors.
-
Customizing Output: Adjust parameters like
prompt
andtemperature
to fine-tune the generated code.
Integration
Codestral integrates with IDEs (VS Code, JetBrains) via plugins like Continue.dev. You can also create custom scripts. Here's an example for generating test functions:
prompt = "def fibonacci(n: int):" suffix = "n = int(input('Enter a number: '))\nprint(fibonacci(n))" data = {"model": "codestral-latest", "prompt": prompt, "suffix": suffix, "temperature": 0} response = call_fim_endpoint(data)
Best Practices
- Clear Prompts: Use precise and unambiguous prompts for optimal results.
- Iterative Refinement: Experiment and refine your prompts for better code generation.
- Responsible Use: Use the API ethically and legally, avoiding malicious code generation.
Conclusion
This guide provides a practical introduction to the Codestral API. Experiment and integrate it into your workflow to enhance your development process. For more on Mistral, explore the Mistral 7B tutorial and the guide to working with the Mistral large model.
The above is the detailed content of Codestral API Tutorial: Getting Started With Mistral's API. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
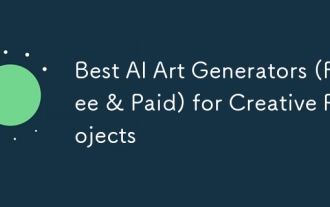
The article reviews top AI art generators, discussing their features, suitability for creative projects, and value. It highlights Midjourney as the best value for professionals and recommends DALL-E 2 for high-quality, customizable art.
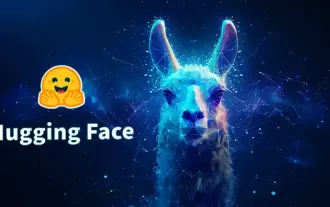
Meta's Llama 3.2: A Leap Forward in Multimodal and Mobile AI Meta recently unveiled Llama 3.2, a significant advancement in AI featuring powerful vision capabilities and lightweight text models optimized for mobile devices. Building on the success o

The article compares top AI chatbots like ChatGPT, Gemini, and Claude, focusing on their unique features, customization options, and performance in natural language processing and reliability.
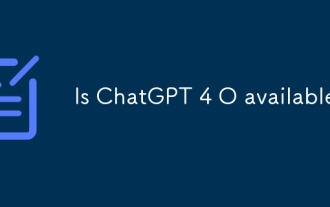
ChatGPT 4 is currently available and widely used, demonstrating significant improvements in understanding context and generating coherent responses compared to its predecessors like ChatGPT 3.5. Future developments may include more personalized interactions and real-time data processing capabilities, further enhancing its potential for various applications.
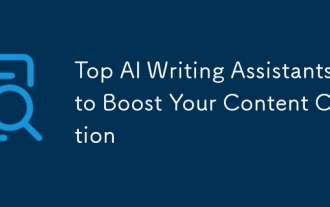
The article discusses top AI writing assistants like Grammarly, Jasper, Copy.ai, Writesonic, and Rytr, focusing on their unique features for content creation. It argues that Jasper excels in SEO optimization, while AI tools help maintain tone consist

The article reviews top AI voice generators like Google Cloud, Amazon Polly, Microsoft Azure, IBM Watson, and Descript, focusing on their features, voice quality, and suitability for different needs.
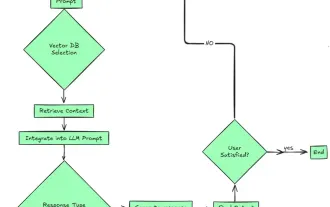
2024 witnessed a shift from simply using LLMs for content generation to understanding their inner workings. This exploration led to the discovery of AI Agents – autonomous systems handling tasks and decisions with minimal human intervention. Buildin
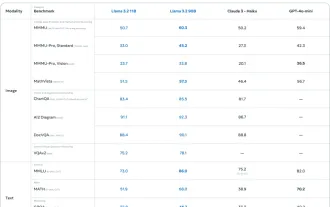
This week's AI landscape: A whirlwind of advancements, ethical considerations, and regulatory debates. Major players like OpenAI, Google, Meta, and Microsoft have unleashed a torrent of updates, from groundbreaking new models to crucial shifts in le
