Python Round Up Function
The upward rounding function is a valuable mathematical tool used by professionals in finance, analytics, and programming. This function ensures numbers are rounded up to a specified level, preventing underestimation. Businesses benefit significantly from this in budgeting, pricing, and statistical analysis. This article explores Python's upward rounding capabilities and its real-world applications.
Learning Objectives
- Define the upward rounding function and its purpose.
- Understand the syntax and parameters of upward rounding functions.
- Apply upward rounding in various contexts (spreadsheets, programming).
- Identify practical applications of upward rounding in real-world scenarios.
Table of contents
- What is the Upward Rounding Function?
- Methods for Upward Rounding in Python
- Real-World Applications
- Method Summary
- Practical Use Cases
- Conclusion
- Frequently Asked Questions
What is the Upward Rounding Function?
The upward rounding function rounds numbers to precise decimal places or multiples of specific values. Unlike traditional rounding, it always results in a value equal to or greater than the input, eliminating underestimation.
Key Characteristics
- Guaranteed Upward Rounding: Always rounds up to the next integer or specified decimal place.
- Underestimation Prevention: Crucial for financial applications where underestimation can lead to budget shortfalls.
Syntax and Parameters
The syntax varies depending on the platform (Excel, Python). A general structure is:
-
Excel:
ROUNDUP(number, num_digits)
-
number
: The value to round up. -
num_digits
: The number of decimal places (positive), or the number of places to the left of the decimal point (negative). 0 rounds to the nearest whole number.
-
-
Python:
math.ceil(x)
- Rounds the floating-point number
x
up to the nearest integer.
- Rounds the floating-point number
Methods to Round Up a Number in Python
Python offers several ways to round up, each with its strengths:
Using the math.ceil()
Function
The math.ceil()
function (from the math
module) is the simplest method for rounding up to the nearest integer.
Example:
import math number = 5.3 rounded_number = math.ceil(number) print(rounded_number) # Output: 6
Custom Upward Rounding Function
For more control, create a custom function:
Example:
import math def round_up(n, decimals=0): multiplier = 10 ** decimals return math.ceil(n * multiplier) / multiplier # Usage result = round_up(3.14159, 2) print(result) # Output: 3.15
This function handles rounding to a specified number of decimal places.
Using NumPy's ceil()
Function
NumPy offers efficient upward rounding for arrays:
Example:
import math number = 5.3 rounded_number = math.ceil(number) print(rounded_number) # Output: 6
Using the decimal
Module
For high-precision applications (e.g., finance), the decimal
module provides accurate rounding:
Example:
import math def round_up(n, decimals=0): multiplier = 10 ** decimals return math.ceil(n * multiplier) / multiplier # Usage result = round_up(3.14159, 2) print(result) # Output: 3.15
Rounding Up with the Built-in round()
Function (Approximation)
While round()
doesn't directly round up, a workaround is possible:
import numpy as np array = np.array([1.1, 2.5, 3.7]) rounded_array = np.ceil(array) print(rounded_array) # Output: [2. 3. 4.]
Real-World Applications
Here are some practical examples:
Retail Pricing
Rounding up prices simplifies transactions and ensures whole-number charges.
Expense Calculation
Rounding up project expenses ensures the budget covers all potential costs.
Project Management Time Estimation
Rounding up time estimates ensures sufficient resource allocation.
Inventory Management
Rounding up inventory levels ensures sufficient stock to meet demand.
Travel Planning
Rounding up distances improves fuel cost and travel time estimations.
Summary of Methods
Method | Description | Example Code | ||||||||||||||||||
---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|---|
math.ceil() |
Rounds up to the nearest integer | math.ceil(5.3) → 6 |
||||||||||||||||||
Custom Function | Rounds up to specified decimal places | round_up(3.14159, 2) → 3.15 |
||||||||||||||||||
NumPy's
|
Rounds up elements in an array | np.ceil([1.1, 2.5]) → [2., 3.] |
||||||||||||||||||
Module | High-precision rounding | Decimal('2.675').quantize(...) → 2.68 |
||||||||||||||||||
Built-in (workaround) | Approximation of upward rounding using built-in round() | round_up_builtin(4.2) → 5 |
Practical Applications
- Finance: Accurate budgeting and financial forecasting.
- Inventory Management: Preventing stockouts.
- Statistical Analysis: Ensuring sufficient sample sizes.
Conclusion
The upward rounding function is a crucial tool for precise calculations across various fields. Understanding its application improves numerical accuracy and decision-making.
Key Takeaways
- Upward rounding always rounds numbers upwards.
- It's applicable in various platforms (Excel, Python).
- Understanding its syntax is essential for correct usage.
- It has diverse applications in finance, inventory, and statistics.
- Mastering this function leads to better budgeting and forecasting.
Frequently Asked Questions
Q1: When to use upward rounding instead of regular rounding? Use upward rounding when underestimation is unacceptable (e.g., budgeting).
Q2: Can negative numbers be rounded up? Yes, they move closer to zero.
Q3: Upward rounding in Google Sheets? Use the ROUNDUP
function.
Q4: num_digits
as a negative value? Rounds to the left of the decimal point.
Q5: Upward rounding for currency? Yes, for accurate financial calculations.
The above is the detailed content of Python Round Up Function. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
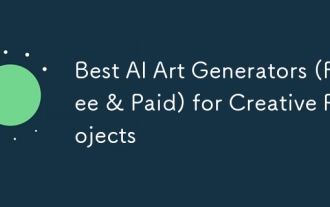
The article reviews top AI art generators, discussing their features, suitability for creative projects, and value. It highlights Midjourney as the best value for professionals and recommends DALL-E 2 for high-quality, customizable art.
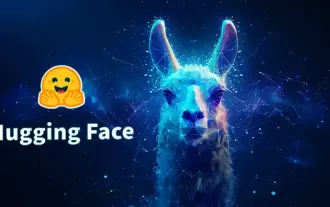
Meta's Llama 3.2: A Leap Forward in Multimodal and Mobile AI Meta recently unveiled Llama 3.2, a significant advancement in AI featuring powerful vision capabilities and lightweight text models optimized for mobile devices. Building on the success o

The article compares top AI chatbots like ChatGPT, Gemini, and Claude, focusing on their unique features, customization options, and performance in natural language processing and reliability.
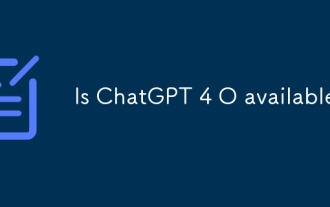
ChatGPT 4 is currently available and widely used, demonstrating significant improvements in understanding context and generating coherent responses compared to its predecessors like ChatGPT 3.5. Future developments may include more personalized interactions and real-time data processing capabilities, further enhancing its potential for various applications.
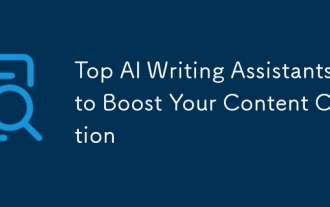
The article discusses top AI writing assistants like Grammarly, Jasper, Copy.ai, Writesonic, and Rytr, focusing on their unique features for content creation. It argues that Jasper excels in SEO optimization, while AI tools help maintain tone consist
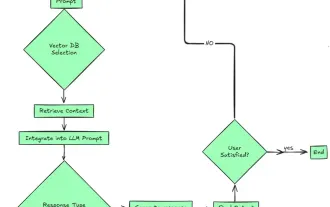
2024 witnessed a shift from simply using LLMs for content generation to understanding their inner workings. This exploration led to the discovery of AI Agents – autonomous systems handling tasks and decisions with minimal human intervention. Buildin

The article reviews top AI voice generators like Google Cloud, Amazon Polly, Microsoft Azure, IBM Watson, and Descript, focusing on their features, voice quality, and suitability for different needs.
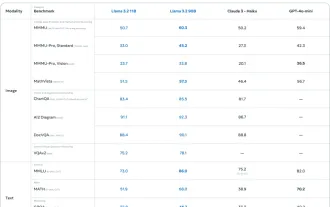
This week's AI landscape: A whirlwind of advancements, ethical considerations, and regulatory debates. Major players like OpenAI, Google, Meta, and Microsoft have unleashed a torrent of updates, from groundbreaking new models to crucial shifts in le
