How to bind a workerman user workerman user binding tutorial
Binding Users to Workerman Connections
Workerman itself doesn't inherently handle user authentication or binding users to connections. It's a highly efficient asynchronous event-driven framework, focusing on handling connections and managing I/O. User authentication and session management are responsibilities you need to implement on top of Workerman. This typically involves using a separate authentication system (like a database or external service) and integrating it with your Workerman application. You'll need to design a protocol for your application to communicate with the authentication system, often involving exchanging credentials (username/password, tokens, etc.) during the connection handshake or subsequent requests. The server then uses the authentication results to associate a user ID or other identifying information with the connection. This can be done by storing the user ID in a connection-specific property within Workerman's connection object.
Implementing User Authentication with Workerman
Implementing user authentication with Workerman generally follows these steps:
-
Choose an Authentication Method: Select a suitable authentication method, such as:
- Username/Password: Simple but requires secure storage and hashing of passwords.
- Token-based Authentication: More secure, involving generating and validating access tokens. JWT (JSON Web Tokens) are a popular choice.
- OAuth 2.0: A widely used authorization framework, ideal for integrating with external services.
- Create an Authentication Service: Build a service (often a separate process or a set of functions) responsible for verifying user credentials. This service will interact with your authentication store (database, LDAP, etc.).
- Integrate with Workerman: When a client connects to your Workerman server, it must provide its credentials. Your Workerman application should receive these credentials, forward them to your authentication service, and receive a verification response.
- Manage Sessions: Upon successful authentication, generate a session ID (or use the access token directly) and store it (either in memory or persistently) alongside the connection. This allows you to identify the user for subsequent requests.
- Handle Subsequent Requests: For each subsequent request from a client, verify the session ID or access token to ensure the client is still authenticated.
Example (Conceptual using username/password and in-memory session):
// ... Workerman connection handling ... $connection->onMessage = function($connection, $data) { // ... Receive username and password from client ... // Authenticate the user $user = authenticateUser($username, $password); // Calls your authentication service if ($user) { // Generate session ID $sessionId = generateSessionId(); $connection->sessionId = $sessionId; // Store session ID in the connection object $connection->send("Authentication successful!"); // ... handle further requests using $connection->sessionId ... } else { $connection->close(); // Close connection on failed authentication } }; function authenticateUser($username, $password) { // ... Your authentication logic here, interacting with a database or other service ... }
Best Practices for Managing User Sessions in a Workerman Application
- Session Expiration: Implement session timeouts to automatically log out users after a period of inactivity.
- Secure Session Storage: If using persistent session storage (e.g., a database), ensure you use secure methods to prevent unauthorized access or modification.
- Session Invalidation: Provide a mechanism to manually invalidate sessions (e.g., upon logout).
- HTTPS: Always use HTTPS to encrypt communication between clients and your Workerman server.
- Avoid Session Fixation: Don't allow clients to choose their own session IDs. Generate them randomly on the server.
- Regular Security Audits: Conduct regular security audits to identify and address potential vulnerabilities.
Security Considerations When Binding Users to Workerman Connections
- Input Validation: Always validate user input to prevent injection attacks (SQL injection, XSS, etc.).
- Secure Password Storage: If using passwords, use strong hashing algorithms (like bcrypt or Argon2) and salt each password individually. Never store passwords in plain text.
- Secure Token Generation: If using tokens, use cryptographically secure random number generators.
- Protection Against Brute-Force Attacks: Implement rate limiting to prevent brute-force attempts to guess credentials.
- Regular Updates: Keep your Workerman installation and any dependent libraries up-to-date with security patches.
- HTTPS: As mentioned before, using HTTPS is crucial for protecting data in transit.
- Access Control: Implement proper access control mechanisms to restrict user access to specific resources or functionalities based on their roles or permissions.
Remember that these are general guidelines. The specific implementation details will depend on your application's requirements and the chosen authentication method. Always prioritize security best practices when working with user authentication and session management.
The above is the detailed content of How to bind a workerman user workerman user binding tutorial. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


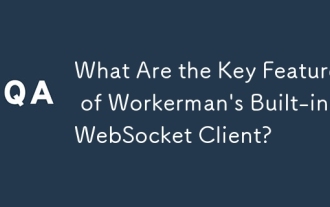
Workerman's WebSocket client enhances real-time communication with features like asynchronous communication, high performance, scalability, and security, easily integrating with existing systems.
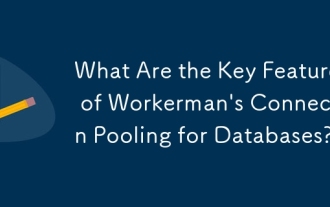
Workerman's connection pooling optimizes database connections, enhancing performance and scalability. Key features include connection reuse, limiting, and idle management. Supports MySQL, PostgreSQL, SQLite, MongoDB, and Redis. Potential drawbacks in
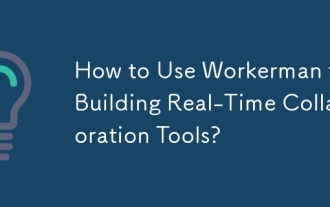
The article discusses using Workerman, a high-performance PHP server, to build real-time collaboration tools. It covers installation, server setup, real-time feature implementation, and integration with existing systems, emphasizing Workerman's key f
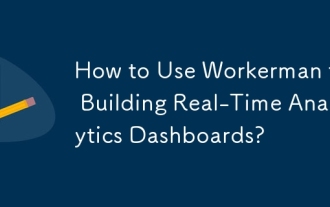
The article discusses using Workerman, a high-performance PHP server, to build real-time analytics dashboards. It covers installation, server setup, data processing, and frontend integration with frameworks like React, Vue.js, and Angular. Key featur

The article discusses implementing real-time data synchronization using Workerman and MySQL, focusing on setup, best practices, ensuring data consistency, and addressing common challenges.

The article discusses integrating Workerman into serverless architectures, focusing on scalability, statelessness, cold starts, resource management, and integration complexity. Workerman enhances performance through high concurrency, reduced cold sta
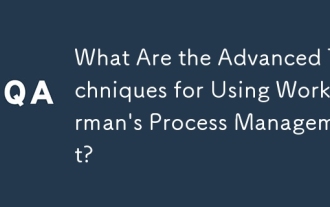
The article discusses advanced techniques for enhancing Workerman's process management, focusing on dynamic adjustments, process isolation, load balancing, and custom scripts to optimize application performance and reliability.
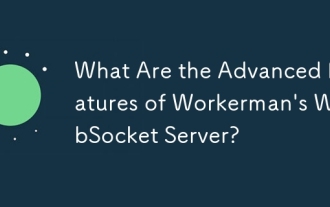
Workerman's WebSocket server enhances real-time communication with features like scalability, low latency, and security measures against common threats.
