WordPress Multi-Multisite: A Case Study
The Challenge: Building a WordPress admin dashboard to efficiently display Google Analytics data from approximately 900 blogs spread across 25 multisite instances. The key was to overcome the performance hurdles inherent in processing such a large dataset.
This article details the development process, highlighting key decisions and challenges encountered. We'll explore the WordPress REST API, the PHP vs. JavaScript debate, production environment limitations, security considerations, database design, and even the role of AI.
Key Terminology
Before diving in, let's clarify some terms:
- WordPress Multisite: A core WordPress feature enabling the management of multiple blogs from a single installation. Blogs share core files and the database, but have individual media folders and blog-specific database tables.
- WordPress Multi-Multisite: A term for managing multiple instances of WordPress multisite installations. This approach avoids the complexities of sharing a single multisite among different clients.
- Dashboard Site: The site hosting the custom dashboard for viewing aggregated analytics data.
- Client Sites: The 25 WordPress multisite instances from which data is collected.
Implementation Strategy
The solution involved a single WordPress plugin installed on both the dashboard site and all 25 client sites. This plugin has two main functions:
- Exposes data via custom API endpoints on client sites.
- Scrapes data from client sites on the dashboard site, caches it, and displays it on the dashboard.
The WordPress REST API: The Foundation
The WordPress REST API was central to this project. Its extensibility allowed the creation of custom endpoints to expose the necessary data.
Code Snippet: API Endpoint Registration
<?php [...] function register(\WP_REST_Server $server) { $endpoints = $this->get(); foreach ($endpoints as $endpoint_slug => $endpoint) { register_rest_route( $endpoint['namespace'], $endpoint['route'], $endpoint['args'] ); } } // ... (rest of the endpoint definitions) ...
PHP vs. JavaScript: The Asynchronous Advantage
Initially, a PHP-based approach was considered. However, synchronous PHP processing and server-side execution time limits made this impractical. JavaScript's asynchronous capabilities offered a superior solution, enabling concurrent data retrieval from all sites.
The JavaScript implementation significantly reduced data retrieval time: from an estimated 925 seconds (synchronous) to approximately 2 seconds (asynchronous). However, browser and server request limits necessitated a 150-millisecond delay between requests.
Code Snippet: Asynchronous Data Fetching
async function getBlogsDetails(blogs) { let promises = []; blogs.forEach((blog, index) => { // ... (code for delayed fetch requests) ... }); // ... (code for Promise.all and error handling) ... }
Connecting PHP and JavaScript
The PHP endpoints and JavaScript code were integrated using wp_localize_script()
, seamlessly passing endpoint URLs and other necessary data to the JavaScript.
Security: Authentication and CORS
Security was addressed through application passwords for API authentication and CORS (Cross-Origin Resource Sharing) headers to allow cross-domain requests from the dashboard site to the client sites. The principle of least privilege was followed, restricting CORS access only to necessary endpoints.
Code Snippet: CORS Header Implementation
<?php [...] function register(\WP_REST_Server $server) { $endpoints = $this->get(); foreach ($endpoints as $endpoint_slug => $endpoint) { register_rest_route( $endpoint['namespace'], $endpoint['route'], $endpoint['args'] ); } } // ... (rest of the endpoint definitions) ...
Database Caching
To improve performance, data is cached in a custom database table on the dashboard site, utilizing a relational database model. The database schema was initially designed using DocBlocks and then refined with the assistance of an LLM.
Code Snippet: Database Table Creation SQL
async function getBlogsDetails(blogs) { let promises = []; blogs.forEach((blog, index) => { // ... (code for delayed fetch requests) ... }); // ... (code for Promise.all and error handling) ... }
Results and Future Considerations
The MVP is functional, providing valuable insights into blog traffic patterns. Future improvements could include using a modern JavaScript framework and exploring serverless solutions like AWS Lambda for improved scalability and performance. The use of cron jobs for pre-emptive data compilation is also a potential enhancement.
This article provides a high-level overview of the development process. The specific challenges and solutions encountered offer valuable insights for developers working with large-scale WordPress multi-multisite deployments.
The above is the detailed content of WordPress Multi-Multisite: A Case Study. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
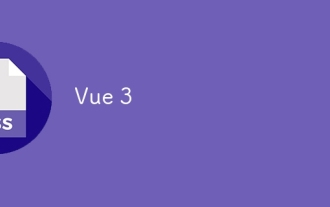
It's out! Congrats to the Vue team for getting it done, I know it was a massive effort and a long time coming. All new docs, as well.
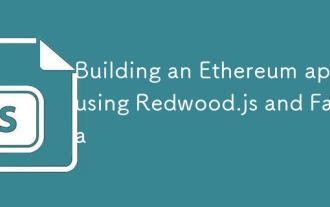
With the recent climb of Bitcoin’s price over 20k $USD, and to it recently breaking 30k, I thought it’s worth taking a deep dive back into creating Ethereum
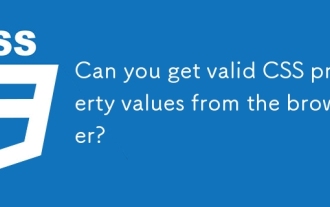
I had someone write in with this very legit question. Lea just blogged about how you can get valid CSS properties themselves from the browser. That's like this.
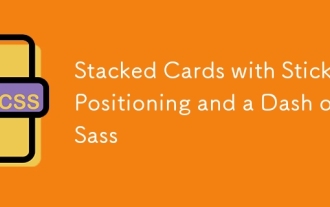
The other day, I spotted this particularly lovely bit from Corey Ginnivan’s website where a collection of cards stack on top of one another as you scroll.
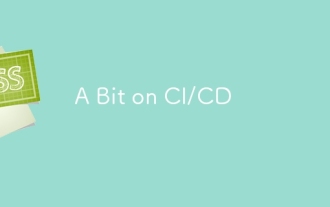
I'd say "website" fits better than "mobile app" but I like this framing from Max Lynch:
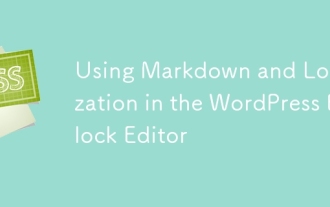
If we need to show documentation to the user directly in the WordPress editor, what is the best way to do it?
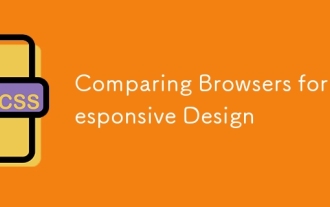
There are a number of these desktop apps where the goal is showing your site at different dimensions all at the same time. So you can, for example, be writing
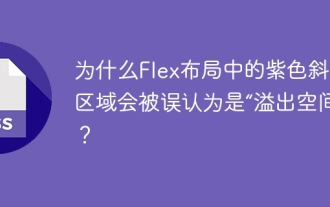
Questions about purple slash areas in Flex layouts When using Flex layouts, you may encounter some confusing phenomena, such as in the developer tools (d...
