Java and Distributed Systems: Implementing Raft Consensus Algorithm
Java and Distributed Systems: Implementing Raft Consensus Algorithm
This section delves into the implementation of the Raft consensus algorithm within a Java environment. Raft is a consensus algorithm designed to manage replicated state machines in distributed systems. Implementing it in Java leverages the language's mature ecosystem and robust libraries, particularly those focused on networking and concurrency. The core components involve defining the Raft roles (Leader, Follower, Candidate), implementing the state machine (persisting logs and applying changes), and managing communication between nodes using techniques like TCP/IP sockets or higher-level frameworks such as Netty. The implementation requires careful consideration of thread safety and concurrency control, given the distributed nature of the system and the need for efficient handling of concurrent requests and messages. Java's built-in concurrency utilities, such as java.util.concurrent
package, are crucial for this aspect. Finally, robust error handling and fault tolerance mechanisms are essential to ensure the system's reliability and availability in the face of network partitions or node failures.
What are the key challenges in implementing the Raft consensus algorithm in a Java environment?
Several key challenges arise when implementing Raft in Java:
- Concurrency Control: Java's multi-threading model requires meticulous attention to concurrency issues. Incorrectly synchronized access to shared resources (like the log) can lead to data corruption and inconsistencies. Proper use of locks, atomic variables, and other concurrency control mechanisms is crucial. This involves carefully managing access to the replicated state machine and ensuring that concurrent operations do not interfere with each other.
- Network Handling: Robustly handling network partitions and delays is paramount. Raft relies on reliable communication between nodes. Java's networking capabilities need to be used effectively to deal with potential network failures, timeouts, and message loss. Strategies like heartbeat mechanisms, reliable message delivery, and retransmission protocols are necessary.
- Persistence: The Raft algorithm requires persistent storage of the log. Choosing and implementing a suitable persistent storage mechanism (e.g., file system, database) in Java is critical for fault tolerance. The persistence mechanism must be durable and efficient to ensure data safety and system performance. Considerations include data integrity, recovery mechanisms, and the performance overhead of writing to persistent storage.
- Testing and Debugging: Testing a distributed system is inherently complex. Simulating network partitions and node failures to thoroughly test the Raft implementation is challenging. Employing techniques like unit testing, integration testing, and simulation frameworks is vital to ensure correctness and robustness. Debugging distributed systems also requires specialized tools and techniques to track down concurrency bugs and network-related issues.
How can I optimize the performance of a Raft-based distributed system built using Java?
Optimizing the performance of a Java-based Raft system requires focusing on several areas:
- Efficient Communication: Minimize network latency by using efficient serialization/deserialization techniques (e.g., Protocol Buffers, Avro) for messages. Optimize network communication patterns to reduce the number of messages exchanged. Consider using asynchronous communication to avoid blocking operations.
- Log Replication Optimization: Efficient log replication is crucial. Techniques like log compaction and snapshotting can significantly reduce the amount of data that needs to be replicated. Optimizing the log storage mechanism can also improve performance.
- Concurrency Optimization: Use efficient data structures and algorithms that minimize contention. Profile the code to identify performance bottlenecks and optimize critical sections. Consider using thread pools to manage concurrent requests effectively.
- Hardware Optimization: Consider using hardware acceleration for computationally intensive tasks, if appropriate. Properly sizing the hardware (CPU, memory, network) for the expected workload is crucial for optimal performance.
- Profiling and Tuning: Use Java profiling tools (e.g., JProfiler, YourKit) to identify performance bottlenecks and optimize the code accordingly. Experiment with different configurations (e.g., number of nodes, timeout values) to find the optimal settings for your system.
What are some common pitfalls to avoid when implementing the Raft consensus algorithm in a Java distributed system?
Several common pitfalls can derail a Raft implementation:
- Incorrect Concurrency Handling: Ignoring concurrency issues can lead to race conditions, data corruption, and inconsistent state. Thoroughly test the code under concurrent conditions using various concurrency testing techniques.
- Ignoring Network Partitions: Failure to handle network partitions robustly can lead to system instability and data loss. Implement proper timeout mechanisms and retry strategies.
- Insufficient Log Persistence: Insufficiently durable log persistence can result in data loss upon node failures. Choose a robust and reliable persistence mechanism and regularly test its durability.
- Incorrect Handling of Timeouts: Incorrectly configured timeouts can lead to incorrect leader election or system instability. Carefully tune timeout values based on the network characteristics and system requirements.
- Ignoring Log Compaction: Failing to implement log compaction can lead to excessively large logs, impacting performance and scalability.
- Insufficient Testing: Thorough testing is crucial to ensure the correctness and robustness of the implementation. Employ a comprehensive testing strategy covering various scenarios, including network partitions and node failures. Using a dedicated testing framework and mocking external dependencies are crucial for effective testing.
The above is the detailed content of Java and Distributed Systems: Implementing Raft Consensus Algorithm. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


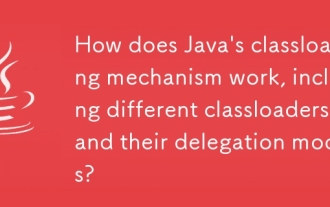
Java's classloading involves loading, linking, and initializing classes using a hierarchical system with Bootstrap, Extension, and Application classloaders. The parent delegation model ensures core classes are loaded first, affecting custom class loa
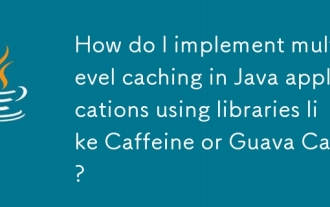
The article discusses implementing multi-level caching in Java using Caffeine and Guava Cache to enhance application performance. It covers setup, integration, and performance benefits, along with configuration and eviction policy management best pra
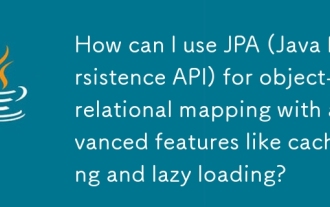
The article discusses using JPA for object-relational mapping with advanced features like caching and lazy loading. It covers setup, entity mapping, and best practices for optimizing performance while highlighting potential pitfalls.[159 characters]
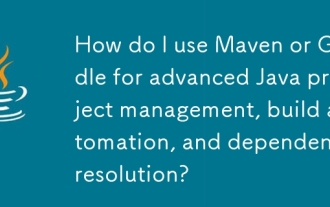
The article discusses using Maven and Gradle for Java project management, build automation, and dependency resolution, comparing their approaches and optimization strategies.
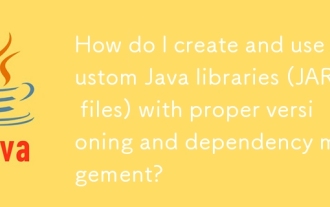
The article discusses creating and using custom Java libraries (JAR files) with proper versioning and dependency management, using tools like Maven and Gradle.
