Building High-Performance RPC Services with gRPC and Spring Boot
Building High-Performance RPC Services with gRPC and Spring Boot
This section details how to build high-performance RPC services leveraging the power of gRPC and Spring Boot. The combination provides a robust framework for creating efficient and scalable services. The key lies in understanding and effectively utilizing gRPC's features alongside Spring Boot's capabilities.
gRPC, being a high-performance RPC framework, already provides a significant advantage in speed and efficiency compared to RESTful services. Spring Boot, with its auto-configuration and simplified development process, further enhances this advantage. To build high-performance services, consider these crucial aspects:
-
Protocol Buffers Optimization: Efficiently designed Protocol Buffer (protobuf) definitions are paramount. Avoid unnecessary fields and use optimal data types. Consider using
repeated
fields sparingly, as they can impact performance if not managed carefully. Regularly review and refine your protobuf definitions based on performance profiling. - Efficient Resource Management: Proper resource management is crucial. Spring Boot's dependency injection and lifecycle management features can help manage connections and resources effectively. Use connection pooling for database interactions and implement proper cleanup mechanisms to avoid resource leaks. Consider asynchronous processing where appropriate to handle concurrent requests efficiently.
- Load Balancing and Scaling: Deploy your gRPC services across multiple instances using a load balancer to distribute traffic evenly. This ensures that no single instance is overwhelmed and maintains consistent performance under heavy load. Cloud-based solutions like Kubernetes are particularly well-suited for managing and scaling gRPC services.
- Caching: Implement caching mechanisms strategically to reduce the load on your backend systems. Cache frequently accessed data to minimize database queries and improve response times. Spring Boot provides integration with various caching solutions like Redis or Ehcache.
How can I optimize gRPC services built with Spring Boot for maximum throughput?
Optimizing gRPC services built with Spring Boot for maximum throughput involves a multi-faceted approach encompassing both client-side and server-side optimizations.
Server-Side Optimizations:
- Asynchronous Processing: Leverage Spring's reactive programming model or utilize asynchronous programming constructs to handle requests concurrently without blocking threads. This significantly improves the ability to handle a high volume of requests.
- Connection Pooling: Efficiently manage connections to external resources (databases, message queues) using connection pooling to avoid the overhead of establishing new connections for each request.
- Server-Side Streaming: For scenarios where the server needs to send a large amount of data to the client, utilize server-side streaming to avoid sending all data at once. This improves responsiveness and reduces memory consumption.
- Performance Tuning: Profile your application to identify bottlenecks. Use tools like JProfiler or YourKit to pinpoint performance issues and optimize accordingly. Consider adjusting JVM parameters (heap size, garbage collection settings) based on your workload.
Client-Side Optimizations:
- Connection Reuse: Clients should reuse connections to reduce the overhead of establishing new connections for each request.
- Batching: Group multiple requests into a single batch to reduce the number of round trips between client and server.
- Load Balancing: Use a client-side load balancer to distribute requests across multiple server instances.
What are the best practices for error handling and logging in gRPC services using Spring Boot?
Robust error handling and comprehensive logging are crucial for maintaining the stability and debuggability of your gRPC services.
Error Handling:
- Use gRPC Status Codes: Utilize gRPC's built-in status codes to communicate errors effectively. Map application-specific errors to appropriate gRPC status codes to provide meaningful error information to the client.
-
Structured Exception Handling: Implement structured exception handling using
try-catch
blocks to gracefully handle exceptions and prevent unexpected crashes. Provide informative error messages to clients. - Centralized Error Handling: Consider using a centralized error handling mechanism to manage errors consistently across your application. Spring Boot provides mechanisms for this through exception handlers and advice.
Logging:
- Use a Structured Logging Library: Employ a structured logging library like Logback or SLF4j to log events in a structured format (e.g., JSON). This makes it easier to analyze logs and monitor the health of your services.
- Log Important Events: Log crucial events such as request start and completion, exceptions, and critical system information. Use different log levels (DEBUG, INFO, WARN, ERROR) appropriately to filter logs based on severity.
- Contextual Logging: Include relevant context information in your log messages, such as request ID, user ID, and timestamps, to aid in debugging and troubleshooting. Spring Boot's MDC (Mapped Diagnostic Context) can be utilized for this purpose.
What are the security considerations when building high-performance RPC services with gRPC and Spring Boot?
Security is paramount when building high-performance RPC services. Several security considerations must be addressed:
- Authentication and Authorization: Implement robust authentication and authorization mechanisms to control access to your services. Use technologies like JWT (JSON Web Tokens) or OAuth 2.0 for authentication. Spring Security provides excellent support for securing Spring Boot applications.
- Transport Layer Security (TLS): Always use TLS/SSL to encrypt communication between clients and servers. This prevents eavesdropping and tampering with data in transit. Configure your gRPC server to require TLS.
- Input Validation: Validate all inputs from clients to prevent injection attacks (SQL injection, command injection, etc.). Use Spring's validation framework to validate data against defined constraints.
- Access Control Lists (ACLs): Implement fine-grained access control using ACLs to restrict access to specific resources or operations based on user roles or permissions.
- Regular Security Audits: Conduct regular security audits and penetration testing to identify and address vulnerabilities. Stay updated with the latest security best practices and patches for your dependencies.
- Rate Limiting: Implement rate limiting to prevent denial-of-service (DoS) attacks by restricting the number of requests from a single client or IP address within a given time window. Spring Cloud provides tools for implementing rate limiting.
By addressing these security concerns proactively, you can build secure and reliable high-performance gRPC services using Spring Boot.
The above is the detailed content of Building High-Performance RPC Services with gRPC and Spring Boot. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


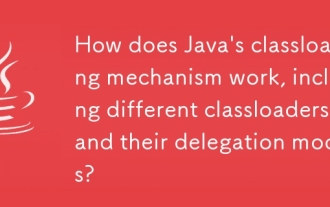
Java's classloading involves loading, linking, and initializing classes using a hierarchical system with Bootstrap, Extension, and Application classloaders. The parent delegation model ensures core classes are loaded first, affecting custom class loa
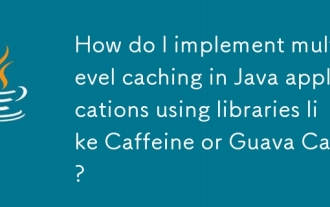
The article discusses implementing multi-level caching in Java using Caffeine and Guava Cache to enhance application performance. It covers setup, integration, and performance benefits, along with configuration and eviction policy management best pra
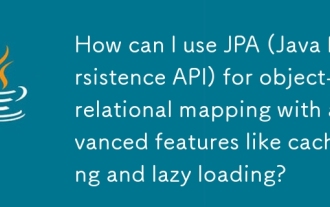
The article discusses using JPA for object-relational mapping with advanced features like caching and lazy loading. It covers setup, entity mapping, and best practices for optimizing performance while highlighting potential pitfalls.[159 characters]
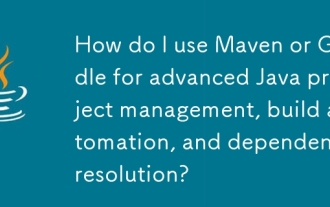
The article discusses using Maven and Gradle for Java project management, build automation, and dependency resolution, comparing their approaches and optimization strategies.
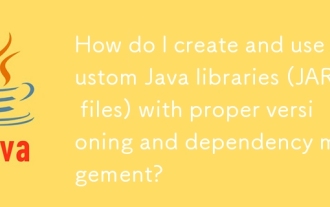
The article discusses creating and using custom Java libraries (JAR files) with proper versioning and dependency management, using tools like Maven and Gradle.
