Custom Hooks in React: Creating Reusable Logic with Examples
Custom Hooks in React: Creating Reusable Logic with Examples
Custom hooks in React are functions that let you reuse stateful logic across multiple components. They start with the word use
and, importantly, must follow the rules of React Hooks (e.g., only called from functional components, not within loops or conditional statements). They allow you to extract complex state management or side-effect logic into reusable units, improving code organization and maintainability. Let's illustrate with an example:
Imagine you need to implement a counter component in multiple places within your application. Instead of rewriting the counter logic each time, you can create a custom hook:
1 2 3 4 5 6 7 8 9 10 11 12 13 |
|
Now, any component can easily use this hook:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 |
|
This drastically reduces code duplication and makes your components cleaner and easier to understand. This example showcases a simple counter, but custom hooks can manage much more complex state, including fetching data, handling form submissions, and integrating with third-party libraries.
What are the benefits of using custom hooks over writing the same logic multiple times in React components?
Using custom hooks offers several significant advantages over repeating the same logic within multiple React components:
- Reduced Code Duplication: This is the most obvious benefit. Instead of writing the same code multiple times, you write it once in a custom hook and reuse it anywhere. This minimizes the risk of inconsistencies and bugs.
- Improved Readability and Maintainability: Custom hooks encapsulate complex logic, making your components cleaner and easier to understand. If you need to modify the logic, you only need to change it in one place (the custom hook), rather than across many components.
- Enhanced Reusability: Custom hooks promote code reusability across different parts of your application. This saves time and effort and helps to create a more consistent user experience.
- Better Organization: Custom hooks help to organize your code into logical units, making it easier to navigate and understand the overall structure of your application. This is especially important in larger projects.
- Easier Testing: Testing custom hooks is generally simpler than testing the same logic embedded within multiple components. You can write unit tests for your custom hooks independently, ensuring their correctness.
How do I effectively structure and organize my custom hooks to maintain code readability and reusability in larger React projects?
Effective structuring and organization are crucial for maintaining readability and reusability in larger projects. Here are some best practices:
- Single Responsibility Principle: Each custom hook should ideally have one specific responsibility. Avoid creating "god hooks" that handle too many unrelated tasks. Smaller, focused hooks are easier to understand, test, and maintain.
-
Descriptive Naming: Use clear and concise names for your custom hooks. The name should accurately reflect the hook's purpose (e.g.,
useFetchData
,useFormValidation
,useAuth
). - Clear Documentation: Write clear and concise documentation for each custom hook, explaining its purpose, parameters, and return values. This helps other developers (and your future self) understand how to use the hook correctly.
- Folder Structure: Organize your custom hooks into a dedicated folder within your project. You might further categorize them based on functionality (e.g., data fetching hooks, form handling hooks, authentication hooks).
- Type Safety: Use TypeScript to add type annotations to your custom hooks. This helps to prevent runtime errors and improves code maintainability.
- Abstraction: Abstract away implementation details within your custom hooks. Users of the hook should only need to interact with a simple, well-defined API.
- Testing: Write unit tests for your custom hooks to ensure their correctness and prevent regressions.
Can I share custom hooks across different React projects, and if so, what's the best way to manage them for optimal version control and deployment?
Yes, you can absolutely share custom hooks across different React projects. The best way to manage them depends on the scale and complexity of your projects:
- npm Package: For larger, widely used custom hooks, creating an npm package is the recommended approach. This allows you to easily install and update the hooks in different projects using npm or yarn. This method provides excellent version control and allows you to manage dependencies effectively.
- Git Submodules or Git Subtrees: For smaller projects or sets of related projects, you can use Git submodules or subtrees to include your custom hooks as a separate Git repository within your main project. This keeps the hooks version controlled but requires more manual management compared to an npm package.
- Shared Library: If the projects are closely related and share a common codebase, you can create a shared library containing your custom hooks. This approach simplifies the management of shared code, but it can make refactoring more complex.
Regardless of the method chosen, version control (using Git) is essential for managing changes, tracking updates, and collaborating on the custom hooks. Using semantic versioning (semver) for your npm package (or even internally for shared libraries) helps maintain consistency and prevents breaking changes across projects. Consider using a continuous integration/continuous deployment (CI/CD) pipeline to automate the building, testing, and deployment of your custom hook library.
The above is the detailed content of Custom Hooks in React: Creating Reusable Logic with Examples. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
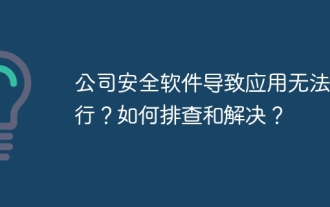
Troubleshooting and solutions to the company's security software that causes some applications to not function properly. Many companies will deploy security software in order to ensure internal network security. ...
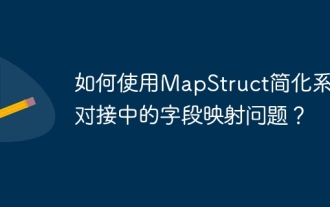
Field mapping processing in system docking often encounters a difficult problem when performing system docking: how to effectively map the interface fields of system A...
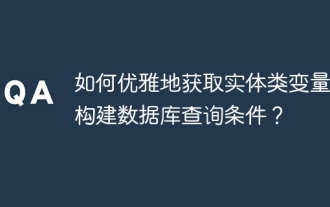
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
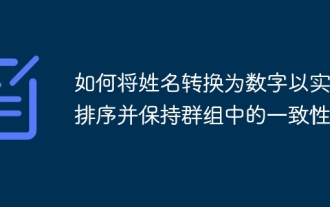
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
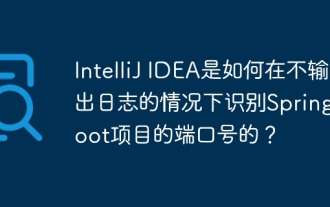
Start Spring using IntelliJIDEAUltimate version...
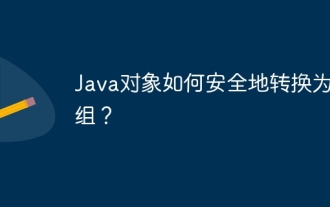
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
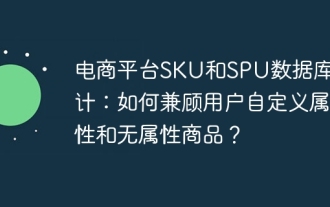
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
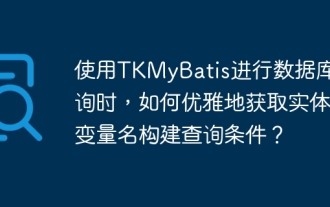
When using TKMyBatis for database queries, how to gracefully get entity class variable names to build query conditions is a common problem. This article will pin...
