Clean Code Principles: Writing Maintainable and Scalable Software
Clean Code Principles: Writing Maintainable and Scalable Software
This question delves into the core tenets of writing clean code. Clean code is not just about making the code work; it's about making it understandable, maintainable, and scalable. Several principles contribute to this goal:
-
Meaningful Names: Choose names that clearly reflect the purpose and function of variables, functions, and classes. Avoid abbreviations or single-letter names unless their meaning is entirely unambiguous within the context. For example,
customerOrderTotal
is far superior tocot
. - Keep Functions Small and Focused: Each function should ideally perform a single, well-defined task. Long functions are difficult to understand and test. Aim for functions that are concise and easy to grasp at a glance. The "Single Responsibility Principle" (SRP) is crucial here.
- Minimize Dependencies: Reduce the number of dependencies between different parts of your code. Highly coupled code is brittle and difficult to change. Strive for loose coupling using techniques like dependency injection.
- Comments Should Explain Why, Not What: Good code should be self-explanatory. Comments should clarify the reasoning behind a particular design choice or a complex algorithm, not simply restate what the code already says.
- Consistent Formatting and Style: Adhere to a consistent coding style guide (e.g., PEP 8 for Python). Consistent formatting improves readability and makes it easier for multiple developers to collaborate on the same project. Use linters and formatters to enforce consistency automatically.
- Test-Driven Development (TDD): Writing tests before writing the code helps ensure that the code meets its requirements and remains functional as the project evolves. This leads to more robust and maintainable code.
How can I improve the readability and maintainability of my existing codebase?
Improving an existing codebase requires a systematic approach:
- Refactoring: This involves restructuring existing code without changing its functionality. Focus on small, incremental changes. Refactor one function or class at a time, ensuring that tests remain green throughout the process.
- Code Reviews: Regular code reviews by peers can identify areas for improvement in readability and maintainability. A fresh pair of eyes can often spot subtle issues that the original author might have missed.
- Static Analysis Tools: Use static analysis tools (like SonarQube, ESLint, or Pylint) to automatically detect potential issues such as code smells, bugs, and violations of coding style guidelines. These tools can help identify areas that need refactoring.
- Documentation: If the existing codebase lacks documentation, add comments and documentation to explain the purpose and functionality of different parts of the code. Consider generating API documentation automatically using tools like Swagger or JSDoc.
- Modularization: Break down large, monolithic components into smaller, more manageable modules. This improves code organization and makes it easier to understand and maintain individual parts of the system.
What are the best practices for writing clean code that scales effectively with growing project complexity?
Scaling clean code requires anticipating future growth and designing for flexibility:
- Design Patterns: Utilize established design patterns (e.g., Model-View-Controller (MVC), Singleton, Factory) to create flexible and reusable code. These patterns provide solutions to common design problems.
- Abstraction: Hide implementation details behind abstract interfaces. This allows you to change the implementation without affecting other parts of the system.
- Loose Coupling: Minimize dependencies between modules. This makes it easier to modify or replace individual components without causing cascading changes throughout the system.
- Modularity: Divide the system into independent modules with well-defined interfaces. This makes it easier to understand, test, and maintain individual parts of the system.
- Scalable Architecture: Consider using a scalable architecture (e.g., microservices) to distribute the workload across multiple machines. This allows the system to handle increased traffic and data volume.
- Version Control: Use a robust version control system (like Git) to track changes and facilitate collaboration among developers.
What tools and techniques can help me enforce clean code principles throughout the software development lifecycle?
Several tools and techniques can assist in maintaining clean code principles:
- Linters and Formatters: These tools automatically check for coding style violations and formatting inconsistencies. Examples include ESLint for JavaScript, Pylint for Python, and SonarLint for many languages.
- Static Analysis Tools: These tools analyze code without executing it to detect potential bugs, security vulnerabilities, and code smells. SonarQube is a popular example.
- Code Review Tools: Tools like GitHub, GitLab, and Bitbucket facilitate code reviews by providing features for commenting and collaboration.
- Continuous Integration/Continuous Delivery (CI/CD): Automate the build, testing, and deployment process to ensure that code changes are integrated and deployed frequently. This helps catch problems early and reduces the risk of introducing bugs.
- Automated Testing Frameworks: Use testing frameworks (like JUnit, pytest, or Jest) to write and run automated tests. This ensures that the code continues to function correctly as it evolves.
- Code Style Guides and Linters: Enforce a consistent coding style throughout the project using a style guide and a linter that automatically checks for adherence to that style.
By consistently applying these principles, tools, and techniques, developers can create a codebase that is not only functional but also maintainable, scalable, and a pleasure to work with.
The above is the detailed content of Clean Code Principles: Writing Maintainable and Scalable Software. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
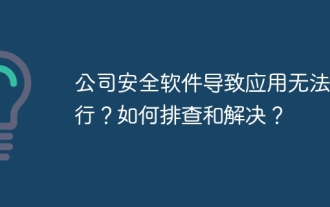
Troubleshooting and solutions to the company's security software that causes some applications to not function properly. Many companies will deploy security software in order to ensure internal network security. ...
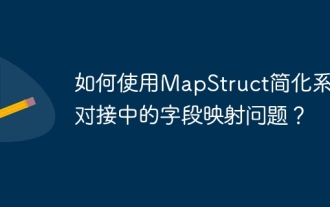
Field mapping processing in system docking often encounters a difficult problem when performing system docking: how to effectively map the interface fields of system A...
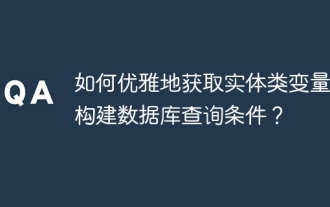
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
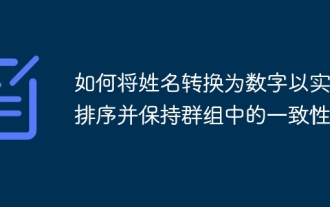
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
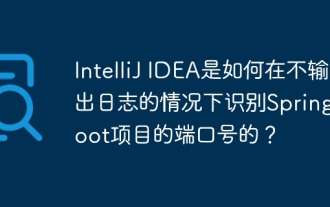
Start Spring using IntelliJIDEAUltimate version...
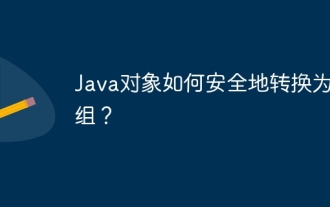
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
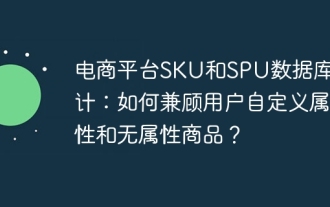
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
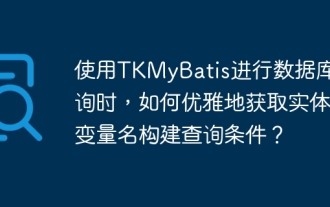
When using TKMyBatis for database queries, how to gracefully get entity class variable names to build query conditions is a common problem. This article will pin...
