Mockito Spy: Mocking a Method in the Same Class Example
Mockito Spy: Mocking a Method in the Same Class Example
This example demonstrates how to use Mockito's spy
functionality to mock a specific method within a class. Let's say we have a class called MyClass
:
public class MyClass { public int add(int a, int b) { return a + b + internalMethod(); } private int internalMethod() { return 5; // This is the method we want to isolate } public int anotherMethod() { return 10; } }
We want to test the add
method, but we don't want the result to be affected by the internalMethod
. We can use a spy to mock just the internalMethod
:
import org.junit.jupiter.api.Test; import org.mockito.Mockito; import static org.junit.jupiter.api.Assertions.assertEquals; import static org.mockito.Mockito.spy; import static org.mockito.Mockito.when; public class MyClassTest { @Test void testAddMethod() { MyClass myClassSpy = spy(MyClass.class); when(myClassSpy.internalMethod()).thenReturn(10); // Mock the internal method int result = myClassSpy.add(2, 3); assertEquals(15, result); // 2 + 3 + 10 = 15 } }
In this example, we create a spy of MyClass
. Then, using when(myClassSpy.internalMethod()).thenReturn(10);
, we stub the internalMethod
to return 10, isolating its behavior from the add
method's test. The assertion then verifies that the add
method behaves correctly given the mocked internalMethod
.
How can I use Mockito's spy
functionality to isolate and test a specific method within a class?
Mockito's spy
allows you to create a partial mock of an existing object. This means you can retain the real implementation of most methods while selectively mocking specific methods. To use it, you create a spy using Mockito.spy(yourObject)
. Then, you use Mockito's when()
method to specify the behavior of the methods you want to mock. For instance:
MyClass myClass = new MyClass(); MyClass myClassSpy = spy(myClass); when(myClassSpy.internalMethod()).thenReturn(10); // Mock only internalMethod
This will create a spy object myClassSpy
. Calls to internalMethod
on myClassSpy
will return 10. All other methods will use their real implementation. This enables targeted testing of a specific method's behavior in isolation from the rest of the class. Remember that you must use when
to define behavior for the method you want to mock; otherwise, it will call the real implementation.
What are the potential pitfalls of using Mockito spies compared to mocks when testing methods within the same class?
While spies offer the advantage of testing interactions with real implementations, they introduce several potential pitfalls:
-
Unintended Side Effects: Since spies retain the original implementation, any side effects of the unmocked methods will still occur. This can lead to unexpected behavior during testing and make it difficult to isolate the unit under test. If
internalMethod
modifies the object's state, that modification will still happen, even though you've mocked its return value. - Difficult Debugging: When unexpected behavior occurs, it can be challenging to pinpoint the source of the error. Is it a problem with the method under test, or a side effect from an unmocked method?
- Tight Coupling: Spies can lead to tighter coupling between your test and the implementation details of your class. Changes in the implementation can break your tests even if the functionality remains the same.
- Unnecessary Complexity: If you can effectively test a method using a simple mock, there's no need for the added complexity of a spy. Mocking is generally simpler and less prone to unexpected side effects.
When should I choose a Mockito spy over a mock when dealing with internal method calls during unit testing?
You should generally favor mocking over spying unless you have a compelling reason to use a spy. Choose a spy when:
- Testing Interactions: You need to test the interactions between the method under test and its internal methods, and the internal methods have significant side effects or dependencies that cannot be easily mocked.
- Legacy Code: You are working with legacy code that is difficult or impossible to refactor to allow for easier mocking.
- Limited Control: You have limited control over the class's internal methods, such as when dealing with final methods or methods with complex dependencies.
However, even in these scenarios, carefully consider the potential pitfalls mentioned above. If possible, refactoring your code to make it more testable is usually a better long-term solution than relying on spies to work around complex dependencies or side effects. Often, a well-structured design with clear separation of concerns will allow for simpler and more reliable tests using mocks.
The above is the detailed content of Mockito Spy: Mocking a Method in the Same Class Example. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


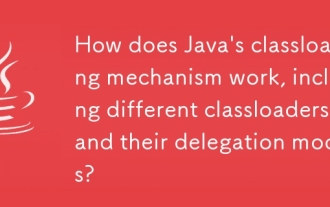
Java's classloading involves loading, linking, and initializing classes using a hierarchical system with Bootstrap, Extension, and Application classloaders. The parent delegation model ensures core classes are loaded first, affecting custom class loa
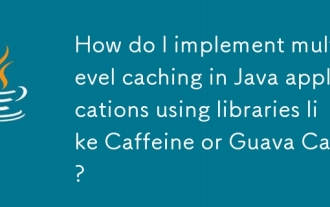
The article discusses implementing multi-level caching in Java using Caffeine and Guava Cache to enhance application performance. It covers setup, integration, and performance benefits, along with configuration and eviction policy management best pra
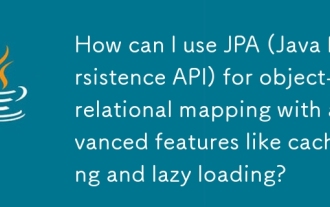
The article discusses using JPA for object-relational mapping with advanced features like caching and lazy loading. It covers setup, entity mapping, and best practices for optimizing performance while highlighting potential pitfalls.[159 characters]
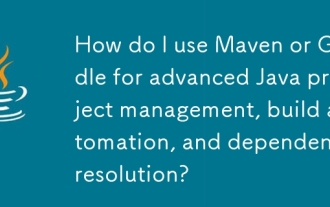
The article discusses using Maven and Gradle for Java project management, build automation, and dependency resolution, comparing their approaches and optimization strategies.
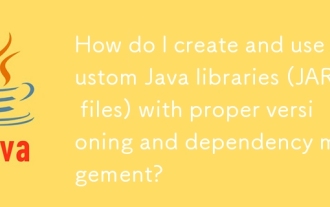
The article discusses creating and using custom Java libraries (JAR files) with proper versioning and dependency management, using tools like Maven and Gradle.
