Serverless JavaScript: Building Scalable APIs with AWS Lambda
Serverless JavaScript: Building Scalable APIs with AWS Lambda
This section explores the core concept of building serverless JavaScript APIs using AWS Lambda, highlighting its fundamental features and capabilities. AWS Lambda is a compute service that lets you run code without provisioning or managing servers. You upload your code, and Lambda takes care of everything required to run and scale it, including computing power, networking, and storage. For JavaScript developers, this means you can focus on writing your API logic, rather than worrying about infrastructure management. You write your code as functions, triggered by various events, such as HTTP requests (using API Gateway), database changes (using DynamoDB Streams), or scheduled events (using CloudWatch Events). These functions are self-contained and only execute when triggered, making it inherently cost-effective as you only pay for the compute time used. The serverless architecture provided by Lambda promotes scalability and resilience; Lambda automatically scales your functions based on incoming requests, ensuring your API can handle peak loads without manual intervention. This eliminates the need for complex scaling configurations often associated with traditional server architectures.
Key Advantages of AWS Lambda for Serverless JavaScript APIs
Compared to traditional server architectures (like those using EC2 instances or other virtual machines), AWS Lambda offers several key advantages for building JavaScript APIs:
- Scalability and Elasticity: Lambda automatically scales your functions based on demand. You don't need to worry about provisioning enough servers to handle peak traffic; Lambda handles this automatically, ensuring high availability and responsiveness. Traditional servers require manual scaling, which can be time-consuming and error-prone.
- Cost-Effectiveness: You only pay for the compute time your functions consume. Unlike traditional servers, where you pay for resources even when they are idle, Lambda offers a pay-as-you-go model, significantly reducing costs, especially for applications with fluctuating traffic.
- Reduced Operational Overhead: Lambda handles infrastructure management, including server provisioning, patching, and maintenance. This frees up developers to focus on building and improving their applications rather than managing infrastructure. Traditional servers require significant operational overhead, including server administration, security updates, and monitoring.
- Faster Deployment: Deploying code to Lambda is typically faster and simpler than deploying to traditional servers. You can quickly iterate and deploy new versions of your functions, accelerating development cycles.
- Integration with other AWS Services: Lambda integrates seamlessly with a vast ecosystem of AWS services, such as API Gateway, DynamoDB, S3, and Cognito, enabling easy access to various functionalities and data stores. This integration simplifies the development process and improves the overall application architecture.
Effectively Handling Errors and Logging in Serverless JavaScript APIs
Robust error handling and logging are crucial for maintaining the reliability and maintainability of serverless APIs. In AWS Lambda, effective error handling and logging can be implemented using the following strategies:
-
Try...Catch Blocks: Wrap your core API logic within
try...catch
blocks to handle potential errors gracefully. This prevents unexpected crashes and allows you to provide informative error messages to clients. - CloudWatch Logs: AWS CloudWatch Logs is a centralized logging service that automatically collects logs from your Lambda functions. Configure your functions to send detailed logs, including error messages, stack traces, and relevant context information. This enables effective monitoring and troubleshooting. Consider using structured logging formats (like JSON) for easier analysis.
- Custom Error Handling Middleware: For more complex error handling scenarios, consider creating custom middleware that intercepts errors and applies consistent error handling logic across your API. This middleware can log errors, format error responses, and potentially trigger alerts.
- Dead-Letter Queues (DLQs): For asynchronous invocations, utilize dead-letter queues (DLQs) to capture messages that fail to process successfully. This allows you to investigate failed events and potentially retry them later. Configure your Lambda functions to send failed events to a designated SQS queue or SNS topic.
- Monitoring and Alerting: Use CloudWatch dashboards and alarms to monitor your Lambda functions for errors and performance issues. Configure alerts to notify you of critical errors or performance degradations.
Best Practices for Designing and Deploying Scalable and Cost-Effective Serverless APIs
Building scalable and cost-effective serverless APIs requires careful consideration of design and deployment strategies. Here are some best practices:
- Function Granularity: Design your functions to be small, focused, and independent. This improves code reusability, maintainability, and scalability. Avoid creating monolithic functions that perform multiple tasks.
- Asynchronous Processing: Utilize asynchronous processing whenever possible to improve performance and scalability. This allows your functions to respond quickly to requests while processing long-running tasks in the background. Services like SQS and SNS are beneficial for this.
- Caching: Employ caching mechanisms (like Redis or ElastiCache) to reduce the load on your functions and improve response times. Caching frequently accessed data can significantly improve performance and reduce costs.
- Layer Management: Utilize Lambda Layers to share common code and dependencies among your functions. This reduces code duplication and simplifies deployment.
- Environment Variables: Use environment variables to manage configuration settings rather than hardcoding them into your code. This enhances portability and simplifies updates.
- Serverless Framework: Employ a serverless framework (like Serverless Framework or AWS SAM) to manage your infrastructure-as-code and streamline the deployment process. This improves consistency and reduces the risk of errors.
- Code Optimization: Optimize your code for performance to minimize execution time and reduce costs. Consider using efficient algorithms and data structures. Profile your code to identify performance bottlenecks.
- IAM Roles and Permissions: Use least privilege principle when configuring IAM roles for your Lambda functions. Grant only the necessary permissions to access AWS resources, minimizing security risks.
By following these best practices, you can build robust, scalable, and cost-effective serverless JavaScript APIs using AWS Lambda.
The above is the detailed content of Serverless JavaScript: Building Scalable APIs with AWS Lambda. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
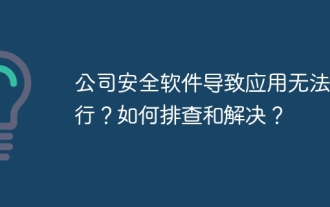
Troubleshooting and solutions to the company's security software that causes some applications to not function properly. Many companies will deploy security software in order to ensure internal network security. ...
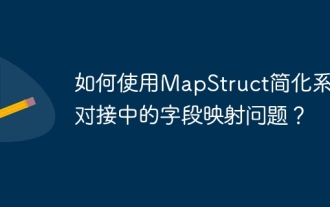
Field mapping processing in system docking often encounters a difficult problem when performing system docking: how to effectively map the interface fields of system A...
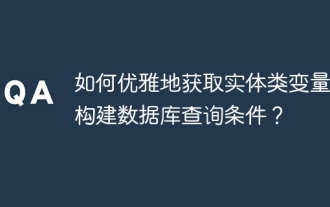
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
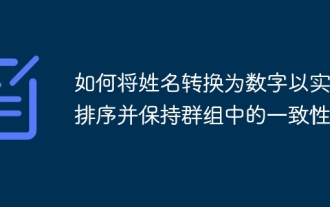
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
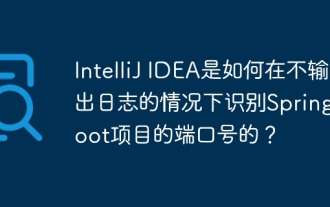
Start Spring using IntelliJIDEAUltimate version...
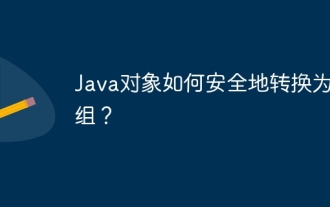
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
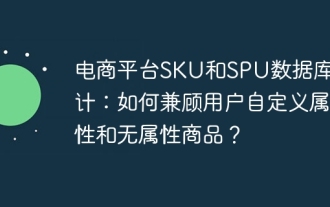
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
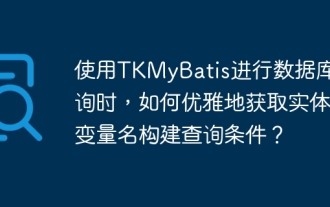
When using TKMyBatis for database queries, how to gracefully get entity class variable names to build query conditions is a common problem. This article will pin...
