Handling JSON in Node.js: Performance Tips with fast-json-stringify
Handling JSON in Node.js: Performance Tips with fast-json-stringify
This article explores the performance benefits of using the fast-json-stringify
library for JSON handling in Node.js applications. We'll address common bottlenecks, explore optimization techniques, and discuss whether it's a suitable replacement for the built-in JSON.stringify
.
Common Performance Bottlenecks When Handling JSON in Node.js, and How fast-json-stringify
Addresses Them
Node.js's built-in JSON.stringify
method, while convenient, can become a performance bottleneck, especially when dealing with large or complex JSON objects in high-throughput applications. Several factors contribute to this:
-
Reflection and Type Checking:
JSON.stringify
performs extensive reflection and type checking for each property. This involves iterating through the object's properties, determining their types, and converting them to their JSON representations. This process is inherently slow, especially for deeply nested objects or objects with numerous properties.fast-json-stringify
pre-compiles the serialization process, eliminating runtime reflection and significantly reducing overhead. -
String Concatenation:
JSON.stringify
often uses string concatenation internally to build the JSON string. String concatenation in JavaScript can be inefficient, particularly with many concatenations.fast-json-stringify
utilizes a more efficient approach, often employing buffer manipulation for faster string building. -
Error Handling: The built-in method includes robust error handling to catch and report issues during serialization. While beneficial for debugging, this adds overhead.
fast-json-stringify
allows for configurable error handling, enabling you to trade off robustness for speed in performance-critical scenarios. You can opt for less rigorous error checking if you are confident in the data integrity.
fast-json-stringify
directly addresses these bottlenecks by:
-
Pre-compilation: It compiles a serialization function specifically tailored to the structure of your JSON object. This eliminates the runtime reflection and type checking performed by
JSON.stringify
. - Optimized String Building: It employs efficient buffer manipulation techniques, minimizing string concatenation overhead.
- Configurable Error Handling: It provides options to control the level of error checking, allowing you to fine-tune the balance between speed and robustness.
How Can I Significantly Improve the Speed of My JSON Serialization in Node.js Applications Using fast-json-stringify
?
Improving the speed of JSON serialization with fast-json-stringify
is relatively straightforward. The key is to leverage its pre-compilation capabilities:
-
Installation: Install the library using npm or yarn:
npm install fast-json-stringify
-
Schema Definition (Optional but Recommended): For optimal performance, define a schema that describes the structure of your JSON objects. This allows
fast-json-stringify
to generate a highly optimized serialization function. The schema can be a simple object defining the properties and their types. -
Compile and Use: Compile the schema into a serialization function using
fastJsonStringify.compile
. This function will return a custom serialization function optimized for the given schema. Then, use this compiled function instead ofJSON.stringify
.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 |
|
-
Benchmarking: Always benchmark your changes to quantify the performance improvements. Use tools like
benchmark.js
to compare the speed ofJSON.stringify
versus your compiledfast-json-stringify
function.
Is fast-json-stringify
a Suitable Replacement for the Built-in JSON.stringify
Method in Node.js, and What are the Trade-offs Involved?
fast-json-stringify
can be a suitable replacement for JSON.stringify
in performance-critical applications where speed is paramount. However, there are trade-offs to consider:
-
Performance:
fast-json-stringify
generally offers significantly faster serialization speeds, especially for large or complex objects. -
Flexibility: The built-in
JSON.stringify
offers more flexibility in handling various data types and edge cases.fast-json-stringify
, while supporting a wide range of types, may require more careful schema definition to handle unusual data structures. -
Error Handling:
fast-json-stringify
offers configurable error handling, allowing for a speed-robustness trade-off. The built-in method provides more robust error handling but at the cost of speed. -
Complexity: Using
fast-json-stringify
introduces an additional dependency and requires a slightly more complex setup, involving schema definition and compilation.
In summary, if your application requires extremely fast JSON serialization and you are comfortable with a slightly more complex setup and potentially less robust error handling, fast-json-stringify
is an excellent choice. However, for applications where performance is not a critical bottleneck, the built-in JSON.stringify
remains a perfectly acceptable and simpler solution.
The above is the detailed content of Handling JSON in Node.js: Performance Tips with fast-json-stringify. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
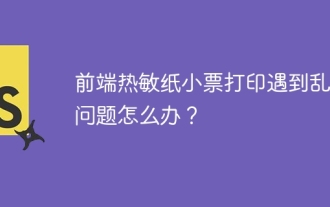
Frequently Asked Questions and Solutions for Front-end Thermal Paper Ticket Printing In Front-end Development, Ticket Printing is a common requirement. However, many developers are implementing...
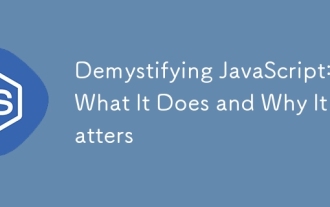
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
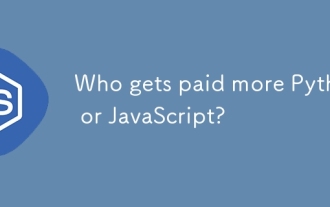
There is no absolute salary for Python and JavaScript developers, depending on skills and industry needs. 1. Python may be paid more in data science and machine learning. 2. JavaScript has great demand in front-end and full-stack development, and its salary is also considerable. 3. Influencing factors include experience, geographical location, company size and specific skills.
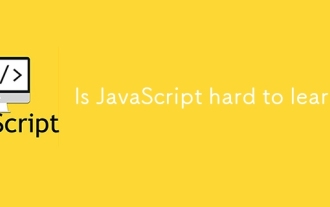
Learning JavaScript is not difficult, but it is challenging. 1) Understand basic concepts such as variables, data types, functions, etc. 2) Master asynchronous programming and implement it through event loops. 3) Use DOM operations and Promise to handle asynchronous requests. 4) Avoid common mistakes and use debugging techniques. 5) Optimize performance and follow best practices.
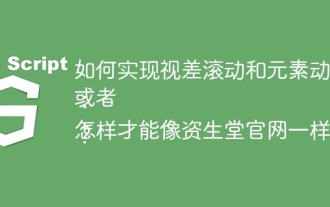
Discussion on the realization of parallax scrolling and element animation effects in this article will explore how to achieve similar to Shiseido official website (https://www.shiseido.co.jp/sb/wonderland/)...
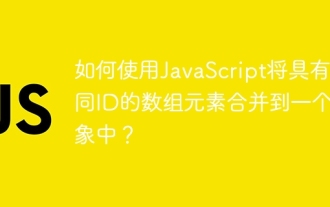
How to merge array elements with the same ID into one object in JavaScript? When processing data, we often encounter the need to have the same ID...
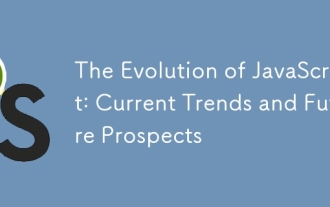
The latest trends in JavaScript include the rise of TypeScript, the popularity of modern frameworks and libraries, and the application of WebAssembly. Future prospects cover more powerful type systems, the development of server-side JavaScript, the expansion of artificial intelligence and machine learning, and the potential of IoT and edge computing.
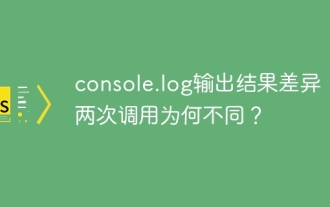
In-depth discussion of the root causes of the difference in console.log output. This article will analyze the differences in the output results of console.log function in a piece of code and explain the reasons behind it. �...
