jquery get label text associated with checkbox
Get the tag text associated with the checkbox using a simple jQuery snippet.
console.log($('label[for="carHireChk"]').html()); $("label[for='comedyclubs']") $("label[for=' + this.attr("id") + ']").attr('class', 'error');
For more information, please refer to the document: https://www.php.cn/link/a5211cd8d2605ed43c70fa82884cc849
jQuery Tag Text Checkbox FAQ (FAQ)
How to use jQuery to get the text of the checkbox selected?
To use jQuery to get the text of the checkbox selected, you can use the .text()
method. This method returns the text content of the selected element. Examples are as follows:
$('input[type="checkbox"]:checked').each(function() { var checkboxText = $(this).next('label').text(); console.log(checkboxText); });
This code snippet selects all selected check boxes and gets the text of its subsequent tag element for each check box.
How to change the text of a tag using jQuery?
To use jQuery to change the text of a tag, you can use the .text()
method. This method sets the text content of the selected element. Examples are as follows:
$('label[for="myCheckbox"]').text('新的标签文本');
This code snippet selects the label of the checkbox with id "myCheckbox" and sets its text to "New Tag Text".
How to use jQuery to select checkboxes through tag text?
To use jQuery to select the checkbox by tag text, you can use the :contains()
selector. This selector selects an element that contains the specified text. Examples are as follows:
$('label:contains("标签文本")').prev('input[type="checkbox"]').prop('checked', true);
This code snippet selects a tag containing "Tag Text" and selects the check box before it.
How to use jQuery to get the value of the selected checkbox?
To use jQuery to get the value of the check box, you can use the .val()
method. This method returns the value of the selected element. Examples are as follows:
$('input[type="checkbox"]:checked').each(function() { var checkboxValue = $(this).val(); console.log(checkboxValue); });
This code snippet selects all selected check boxes and gets its value for each check box.
How to use jQuery to select or uncheck the checkbox?
To use jQuery to select or uncheck the check box, you can use the .prop()
method. This method sets the properties of the selected element. Examples are as follows:
// 选中 $('input[type="checkbox"]').prop('checked', true); // 取消选中 $('input[type="checkbox"]').prop('checked', false);
This code snippet selects all check boxes and selects or unchecks them.
How to toggle checkboxes using jQuery?
To use jQuery to switch the checkbox, you can use the .click()
method. This method simulates a mouse click on the selected element. Examples are as follows:
$('input[type="checkbox"]').click();
This code snippet selects all check boxes and toggles their status.
How to use jQuery to bind event handlers to change events in checkboxes?
To use jQuery to bind the event handler to the change event that check box, you can use the .change()
method. This method attaches the event handler function to the change event. Examples are as follows:
$('input[type="checkbox"]').change(function() { if ($(this).is(':checked')) { console.log('复选框已选中'); } else { console.log('复选框已取消选中'); } });
This code snippet selects all check boxes and binds the event handler to their change events.
How to use jQuery to select all check boxes?
To use jQuery to select all check boxes, you can use the :checkbox
selector. This selector selects all check box input. Examples are as follows:
console.log($('label[for="carHireChk"]').html()); $("label[for='comedyclubs']") $("label[for=' + this.attr("id") + ']").attr('class', 'error');
This code snippet selects all check boxes and selects them.
How to use jQuery to deselect all check boxes?
To use jQuery to deselect all check boxes, you can use the :checkbox
selector. This selector selects all check box input. Examples are as follows:
$('input[type="checkbox"]:checked').each(function() { var checkboxText = $(this).next('label').text(); console.log(checkboxText); });
This code snippet selects all check boxes and unchecks them.
How to use jQuery to get the number of check boxes selected?
To use jQuery to get the number of selected check boxes, you can use the .length
property. This property returns the number of elements in the jQuery object. Examples are as follows:
$('label[for="myCheckbox"]').text('新的标签文本');
This code snippet selects all selected check boxes and gets their number.
The above is the detailed content of jquery get label text associated with checkbox. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


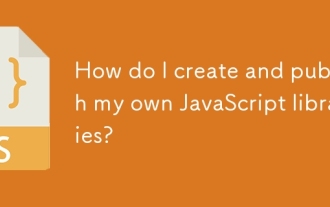
Article discusses creating, publishing, and maintaining JavaScript libraries, focusing on planning, development, testing, documentation, and promotion strategies.
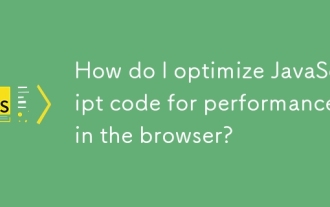
The article discusses strategies for optimizing JavaScript performance in browsers, focusing on reducing execution time and minimizing impact on page load speed.
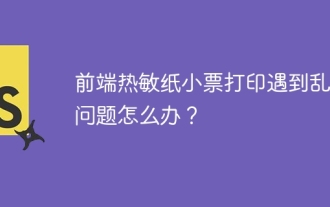
Frequently Asked Questions and Solutions for Front-end Thermal Paper Ticket Printing In Front-end Development, Ticket Printing is a common requirement. However, many developers are implementing...
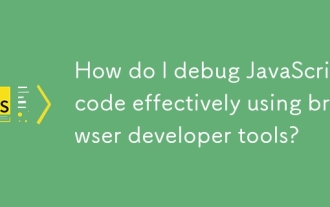
The article discusses effective JavaScript debugging using browser developer tools, focusing on setting breakpoints, using the console, and analyzing performance.
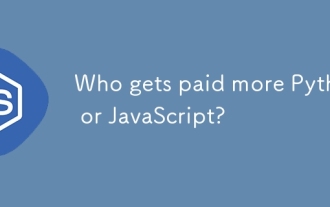
There is no absolute salary for Python and JavaScript developers, depending on skills and industry needs. 1. Python may be paid more in data science and machine learning. 2. JavaScript has great demand in front-end and full-stack development, and its salary is also considerable. 3. Influencing factors include experience, geographical location, company size and specific skills.
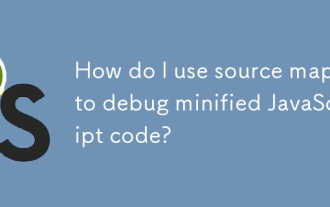
The article explains how to use source maps to debug minified JavaScript by mapping it back to the original code. It discusses enabling source maps, setting breakpoints, and using tools like Chrome DevTools and Webpack.

This tutorial will explain how to create pie, ring, and bubble charts using Chart.js. Previously, we have learned four chart types of Chart.js: line chart and bar chart (tutorial 2), as well as radar chart and polar region chart (tutorial 3). Create pie and ring charts Pie charts and ring charts are ideal for showing the proportions of a whole that is divided into different parts. For example, a pie chart can be used to show the percentage of male lions, female lions and young lions in a safari, or the percentage of votes that different candidates receive in the election. Pie charts are only suitable for comparing single parameters or datasets. It should be noted that the pie chart cannot draw entities with zero value because the angle of the fan in the pie chart depends on the numerical size of the data point. This means any entity with zero proportion
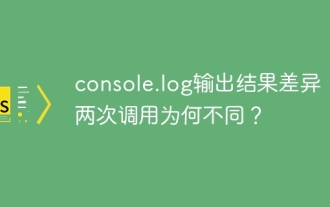
In-depth discussion of the root causes of the difference in console.log output. This article will analyze the differences in the output results of console.log function in a piece of code and explain the reasons behind it. �...
