How to Transition to Manifest V3 for Chrome Extensions
Recently, one of my clients rejected my extension because it was "outdated", which prompted me to dig into the Manifest V3 of Chrome extensions. There is a lack of information about Manifest V3 online, and it is difficult to understand.
After some hard work, I finally completed the task, but I didn't want my research results to be wasted, so I decided to share my learning experience.
The importance of Manifest V3 migration
Manifest V3 is Google's API for Chrome, the successor to the current API Manifest V2, which manages how Chrome extensions interact with the browser. Manifest V3 has made significant changes to the extension’s rules, some of which will become the new pillars of the V2 we are used to using.
The migration of Manifest V3 can be summarized as follows:
- The migration has been underway since 2018.
- Manifest V3 will officially start launching in January 2023.
- By June 2023, extensions running Manifest V2 will no longer be available in the Chrome Web Store.
- Extensions that do not comply with the new rules introduced in Manifest V3 will eventually be removed from the Chrome Web Store.
One of the main goals of Manifest V3 is to improve user security and improve the overall browser experience. Previously, many browser extensions depended on cloud-based code, which meant it was difficult to evaluate whether the extension was risky. Manifest V3 is designed to solve this problem by requiring the extension to include all the code that will be run, allowing Google to scan them and detect potential risks. It also forces the extension to request permission from Google for changes it can implement on the browser.
It is important to keep up with Google's migration to Manifest V3 because it introduces new rules for extensions designed to improve user security and overall browser experience, and extensions that do not comply with these rules will eventually be removed from the Chrome Web Store.
In short, if you don't migrate as quickly as possible, all your efforts to create extensions that use Manifest V2 can go to waste.
Key Differences between Manifest V2 and V3
There are many differences between the two, and while I strongly recommend you read Chrome's "Migrate to Manifest V3" guide, here's a brief summary of the key points:
- In Manifest V3, service workers replace the backend page.
- Net request modification is handled in Manifest V3 using the new declarativeNetRequest API.
- In Manifest V3, extensions can only execute JavaScript code contained in their packages, and cannot use remotely managed code.
- Manifest V3 introduces Promise support for many methods, although callbacks are still supported as an alternative.
- Host permissions in Manifest V3 are a separate element and must be specified in the "host_permissions" field.
- The content security policy in Manifest V3 is an object whose members represent an alternative content security policy (CSP) context, rather than a string in Manifest V2.
In a list of Chrome extensions that simply change the background of a web page, it might look like this:
<code>// Manifest V2 { "manifest_version": 2, "name": "Shane's Extension", "version": "1.0", "description": "A simple extension that changes the background of a webpage to Shane's face.", "background": { "scripts": ["background.js"], "persistent": true }, "browser_action": { "default_popup": "popup.html" }, "permissions": [ "activeTab", ], "optional_permissions": ["<all_urls>"] }</all_urls></code>
<code>// Manifest V3 { "manifest_version": 3, "name": "Shane's Extension", "version": "1.0", "description": "A simple extension that changes the background of a webpage to Shane's face.", "background": { "service_worker": "background.js" }, "action": { "default_popup": "popup.html" }, "permissions": [ "activeTab", ], "host_permissions": [ "<all_urls>" ] }</all_urls></code>
If you find some of the tags above look strange, keep reading to learn what you need to know.
How to migrate to Manifest V3
I have summarized the migration to Manifest V3 into four key areas. Of course, while there are a lot of new features in the new Manifest V3 that need to be implemented from the old Manifest V2, making changes in these four areas will prepare your Chrome extension for the final migration.
The four key areas are:
- Update the basic structure of the list.
- Modify host permissions.
- Update content security policy.
- Modify network request processing.
Through these four aspects, the basic elements of your list will be ready to migrate to Manifest V3. Let's take a look at each key aspect in detail and learn how to work to protect your Chrome extension from this migration.
Basic structure of update list
The basic structure of updating the manifest is the first step in moving to Manifest V3. The most important change you need to make is to change the value of the "manifest_version" element to 3, which means you are using the Manifest V3 feature set.
One of the main differences between Manifest V2 and V3 is the replacement of the backend page with a single extension service worker in Manifest V3. You need to register a service worker under the "background" field, use the "service_worker" key and specify a single JavaScript file. Even if Manifest V3 does not support multiple background scripts, you can choose to declare the service worker as an ES module by specifying "type": "module", which allows you to import more code.
In Manifest V3, the "browser_action" and "page_action" attributes are merged into a single "action" attribute. You need to replace these properties with "action" in the manifest. Similarly, the chrome.browserAction and chrome.pageAction APIs in Manifest V3 are merged into a single 'Action' API, which you need to migrate to.
<code>// Manifest V2 "background": { "scripts": ["background.js"], "persistent": false }, "browser_action": { "default_popup": "popup.html" },</code>
<code>// Manifest V3 "background": { "service_worker": "background.js" }, "action": { "default_popup": "popup.html" }</code>
In general, the basic structure of the update manifest is a key step in the migration to Manifest V3, as it allows you to take advantage of new features and changes introduced in this version of the API.
Modify host permissions
The second step in migrating to Manifest V3 is to modify host permissions. In Manifest V2, you specify host permissions in the "permissions" field of the manifest file. In Manifest V3, host permissions are a separate element that you should specify in the "host_permissions" field of the manifest file.
The following is an example of how to modify host permissions:
<code>// Manifest V2 { "manifest_version": 2, "name": "Shane's Extension", "version": "1.0", "description": "A simple extension that changes the background of a webpage to Shane's face.", "background": { "scripts": ["background.js"], "persistent": true }, "browser_action": { "default_popup": "popup.html" }, "permissions": [ "activeTab", ], "optional_permissions": ["<all_urls>"] }</all_urls></code>
<code>// Manifest V3 { "manifest_version": 3, "name": "Shane's Extension", "version": "1.0", "description": "A simple extension that changes the background of a webpage to Shane's face.", "background": { "service_worker": "background.js" }, "action": { "default_popup": "popup.html" }, "permissions": [ "activeTab", ], "host_permissions": [ "<all_urls>" ] }</all_urls></code>
Update content security policy
In order to update the CSP of the Manifest V2 extension to be consistent with Manifest V3, you need to make some changes to the manifest file. In Manifest V2, CSP is specified as a string in the "content_security_policy" field of the manifest.
In Manifest V3, CSP is now an object whose different members represent an alternative CSP context. Instead of using a single "content_security_policy" field now, you need to specify separate fields for "content_security_policy.extension_pages" and "content_security_policy.sandbox" depending on the type of the extension page you are using.
If you have an external domain in your current CSP, you should also delete any references from the "script-src", "worker-src", "object-src", and "style-src" directives. Making these CSP updates is important to ensure the security and stability of the extension in Manifest V3.
<code>// Manifest V2 "background": { "scripts": ["background.js"], "persistent": false }, "browser_action": { "default_popup": "popup.html" },</code>
<code>// Manifest V3 "background": { "service_worker": "background.js" }, "action": { "default_popup": "popup.html" }</code>
Modify network request processing
The last step in migrating to Manifest V3 is to modify the network request processing. In Manifest V2, you will use the chrome.webRequest API to modify network requests. However, this API is replaced by the declarativeNetRequest API in Manifest V3.
To use this new API, you need to specify the declarativeNetRequest permission in the manifest and update the code to use the new API. A key difference between the two APIs is that the declarativeNetRequest API requires you to specify a list of pre-defined addresses to block, rather than blocking the entire HTTP request category like using the chrome.webRequest API.
Making these changes to the code is very important to ensure that the extension continues to function properly under Manifest V3. Here is an example of how to modify the manifest to use the declarativeNetRequest API in Manifest V3:
<code>// Manifest V2 "permissions": [ "activeTab", "storage", "http://www.css-tricks.com/", ":///*" ]</code>
<code>// Manifest V3 "permissions": [ "activeTab", "scripting", "storage" ], "host_permissions": [ "http://www.css-tricks.com/" ], "optional_host_permissions": [ ":///*" ]</code>
You also need to update the extension code to use the declarativeNetRequest API instead of the chrome.webRequest API.
Other aspects that need to be checked
What I have introduced is just the tip of the iceberg. Of course, if I wanted to cover everything, I might be here for days, and Google's Chrome Developer Guide doesn't make sense. While what I've covered will make your Chrome extension safe enough for this migration, here are some other things you might want to check out to make sure your extension works properly.
- Migrate background scripts to service worker execution context: As mentioned earlier, Manifest V3 replaces the background page with a single extension service worker, so it may be necessary to update the background scripts to suit the service worker execution context.
-
Unified
chrome.browserAction
andchrome.pageAction
API: These two equivalent APIs are merged into a single API in Manifest V3, so they may need to be migrated to the Action API. -
Migrate Expectations Functions of Manifest V2 background context:
chrome.runtime.getBackgroundPage()
The adoption of service workers in Manifest V3 is incompatible with methods such aschrome.extension.getBackgroundPage()
,chrome.extension.getExtensionTabs()
,chrome.extension.getViews()
and . It may be necessary to migrate to a design that passes messages between other contexts and backend service workers. - Move CORS requests in the content script to the background service worker: In order to comply with Manifest V3, it may be necessary to move CORS requests in the content script to the background service worker.
-
Migrate away from executing external code or arbitrary strings:
chrome.scripting.executeScript({code: '...'})
Manifest V3 is no longer allowed to execute external logic usingeval()
,new Function()
andchrome.runtime.getURL()
. It may be necessary to move all external code (JavaScript, WebAssembly, CSS) into the extension package, update scripts and style references to load resources from the extension package, and build the resource URL at runtime using . - Update some scripts and CSS methods in the tab API: As mentioned earlier, some methods move from the tab API to the script API in Manifest V3. Any calls to these methods may need to be updated to use the correct Manifest V3 API.
and more!
Please take some time to understand all the changes. After all, this change is inevitable, and if you don't want to lose the Manifest V2 extension because of avoiding this migration, take some time to master the necessary knowledge.
On the other hand, if you are new to Chrome extension programming and want to get started, a great way is to dig into the world of Chrome's web developer tools. I did this with a course on Linkedin Learning, which gave me a quick grasp of the speed. Once you have mastered these basics, please go back to this article and translate what you know based on Manifest V3!
So, how do I use the features in the new Manifest V3 in the future?
chrome.webRequestAPI
For me, the migration to Manifest V3 and the removal of
The rise of artificial intelligence tools in recent years, many of which provide available APIs, has inspired a large number of new and fresh SaaS applications. I personally think this is right up to the time toward more Chrome extensions based on applications! While many old extensions may be cleared by this migration, many new extensions built around novel SaaS ideas will be replaced.
So this is an exciting update that can be used to improve old extensions or build new ones! I personally think there are many possibilities to use APIs involving artificial intelligence in extensions to enhance the user browsing experience. But this is really just the tip of the iceberg. If you want to really use your own professional extension or contact a company to build/update extensions for them, I recommend upgrading your Gmail account because it has advantages in collaborating, developing and publishing extensions to the Chrome Web Store.
However, remember that each developer needs differently, so learn what you need to keep the current extension running, or have a new extension running!
The above is the detailed content of How to Transition to Manifest V3 for Chrome Extensions. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
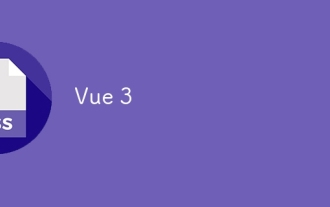
It's out! Congrats to the Vue team for getting it done, I know it was a massive effort and a long time coming. All new docs, as well.
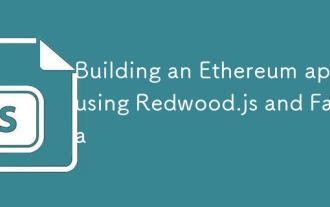
With the recent climb of Bitcoin’s price over 20k $USD, and to it recently breaking 30k, I thought it’s worth taking a deep dive back into creating Ethereum
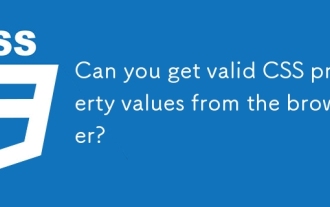
I had someone write in with this very legit question. Lea just blogged about how you can get valid CSS properties themselves from the browser. That's like this.
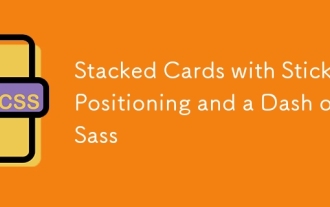
The other day, I spotted this particularly lovely bit from Corey Ginnivan’s website where a collection of cards stack on top of one another as you scroll.
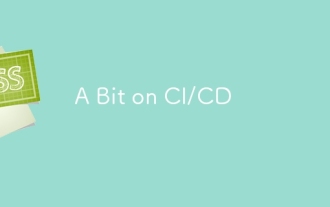
I'd say "website" fits better than "mobile app" but I like this framing from Max Lynch:
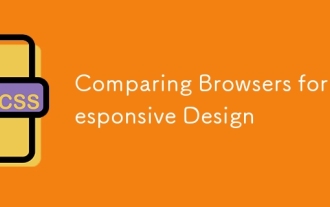
There are a number of these desktop apps where the goal is showing your site at different dimensions all at the same time. So you can, for example, be writing
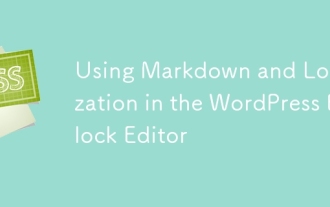
If we need to show documentation to the user directly in the WordPress editor, what is the best way to do it?
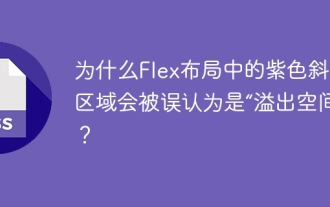
Questions about purple slash areas in Flex layouts When using Flex layouts, you may encounter some confusing phenomena, such as in the developer tools (d...
