


How can I use context effectively for cancellation and timeouts in Go?
How to Use Context Effectively for Cancellation and Timeouts in Go
Go's context
package provides a powerful mechanism for managing cancellation and timeouts in concurrent programs. It's crucial for writing robust and efficient code, especially when dealing with long-running operations. The context.Context
interface represents a deadline, a cancellation signal, and other request-scoped values. You can create contexts with deadlines using context.WithTimeout
or cancellation signals using context.WithCancel
. These functions return a new context.Context
and a context.CancelFunc
. The CancelFunc
allows you to manually cancel the context, triggering cancellation signals downstream. When a context is canceled, all operations using that context should gracefully terminate.
Let's illustrate with an example:
package main import ( "context" "fmt" "time" ) func longRunningTask(ctx context.Context) { for { select { case <-ctx.Done(): fmt.Println("Task cancelled") return case <-time.After(1 * time.Second): fmt.Println("Task is running...") } } } func main() { ctx, cancel := context.WithTimeout(context.Background(), 5*time.Second) defer cancel() go longRunningTask(ctx) <-ctx.Done() // Wait for the context to be done (either timeout or cancellation) fmt.Println("Main function exiting") }
In this example, longRunningTask
continuously runs until the context is canceled. The context.WithTimeout
creates a context that will be canceled after 5 seconds. The defer cancel()
ensures that the context is canceled even if there are errors. The <-ctx.Done()
channel waits for the context to be canceled, allowing the main function to exit gracefully.
Best Practices for Handling Context Cancellation in Go Concurrent Programs
Effective context cancellation in concurrent Go programs hinges on proper propagation and handling of the context.Context
. Here are some best practices:
- Pass context down: Always pass the context to any goroutine or function that might perform long-running operations. This allows the operation to be canceled if the context is canceled.
- Check ctx.Done(): Regularly check
ctx.Done()
within your goroutines. This channel closes when the context is canceled. Use aselect
statement to handle both the cancellation and other events concurrently. - Graceful shutdown: Upon receiving a cancellation signal (by checking
ctx.Done()
), perform cleanup operations like closing files, releasing resources, and ensuring data consistency. Avoid panics; instead, handle errors gracefully. - Avoid blocking operations: If a long-running operation might block indefinitely, use a select statement to check
ctx.Done()
periodically to prevent the goroutine from hanging. - Context propagation in libraries: If you're creating reusable components or libraries, design them to accept a context and propagate it to all their internal operations.
How to Properly Propagate Context Across Goroutines to Manage Timeouts and Cancellations
Context propagation ensures that all parts of your concurrent program are aware of the overall timeout or cancellation. This is done by passing the context to every goroutine that needs to be aware of it. The context should be the first argument to any function that performs potentially long-running operations.
Example illustrating context propagation:
package main import ( "context" "fmt" "time" ) func longRunningTask(ctx context.Context) { for { select { case <-ctx.Done(): fmt.Println("Task cancelled") return case <-time.After(1 * time.Second): fmt.Println("Task is running...") } } } func main() { ctx, cancel := context.WithTimeout(context.Background(), 5*time.Second) defer cancel() go longRunningTask(ctx) <-ctx.Done() // Wait for the context to be done (either timeout or cancellation) fmt.Println("Main function exiting") }
This example demonstrates how the context is passed to each worker
goroutine. Each worker checks ctx.Done()
and exits gracefully when the context is canceled.
Common Pitfalls to Avoid When Using context.WithTimeout and context.WithCancel in Go
Several common pitfalls can arise when using context.WithTimeout
and context.WithCancel
:
- Ignoring context: The most significant pitfall is failing to pass the context to all relevant functions and goroutines. This prevents cancellation from working correctly.
- Leaking goroutines: If you don't properly handle context cancellation, you might end up with goroutines that continue running indefinitely, consuming resources even after the main program has finished.
- Ignoring errors: Always check for errors when creating or using contexts. Errors might indicate issues like invalid deadlines or resource exhaustion.
- Incorrect deadline handling: Ensure you're using the correct deadline and that it's appropriate for the task. Setting overly short or long timeouts can lead to unexpected behavior.
- Overlapping contexts: Avoid creating nested contexts without careful consideration. Incorrect nesting can lead to unexpected cancellation behavior. Use the appropriate context for the specific task.
By following these best practices and avoiding these pitfalls, you can effectively use Go's context
package to create robust, efficient, and cancellable concurrent programs.
The above is the detailed content of How can I use context effectively for cancellation and timeouts in Go?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
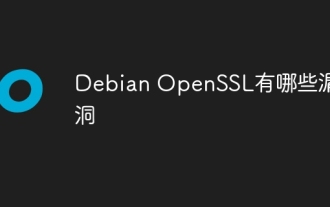
OpenSSL, as an open source library widely used in secure communications, provides encryption algorithms, keys and certificate management functions. However, there are some known security vulnerabilities in its historical version, some of which are extremely harmful. This article will focus on common vulnerabilities and response measures for OpenSSL in Debian systems. DebianOpenSSL known vulnerabilities: OpenSSL has experienced several serious vulnerabilities, such as: Heart Bleeding Vulnerability (CVE-2014-0160): This vulnerability affects OpenSSL 1.0.1 to 1.0.1f and 1.0.2 to 1.0.2 beta versions. An attacker can use this vulnerability to unauthorized read sensitive information on the server, including encryption keys, etc.
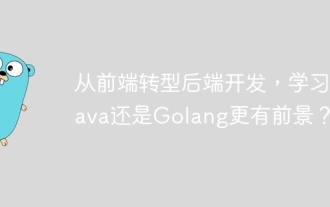
Backend learning path: The exploration journey from front-end to back-end As a back-end beginner who transforms from front-end development, you already have the foundation of nodejs,...
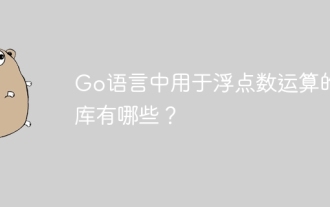
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
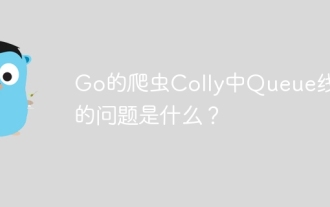
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
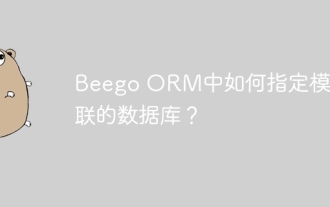
Under the BeegoORM framework, how to specify the database associated with the model? Many Beego projects require multiple databases to be operated simultaneously. When using Beego...
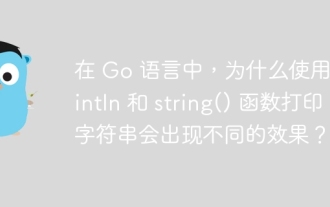
The difference between string printing in Go language: The difference in the effect of using Println and string() functions is in Go...
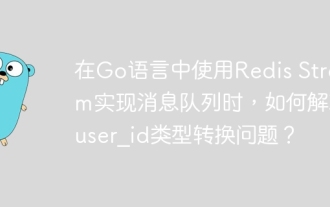
The problem of using RedisStream to implement message queues in Go language is using Go language and Redis...
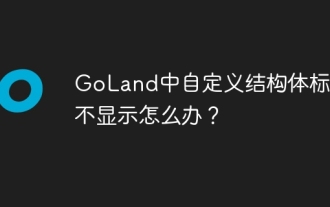
What should I do if the custom structure labels in GoLand are not displayed? When using GoLand for Go language development, many developers will encounter custom structure tags...
