


How does the Go scheduler work and how can I optimize my code for it?
How Does the Go Scheduler Work and How Can I Optimize My Code for It?
The Go scheduler is a sophisticated, work-stealing scheduler designed for concurrency and efficiency. It manages goroutines, lightweight, independently executing functions, and maps them to operating system threads. It doesn't use a traditional one-to-one mapping of goroutines to threads; instead, it employs a many-to-many model. This means multiple goroutines can run on a single OS thread, and a single OS thread can execute multiple goroutines. This flexibility is crucial for efficient resource utilization.
The scheduler's core components include:
- M: Machine: Represents an OS thread.
- P: Processor: A logical processor that schedules goroutines. Each P has its own run queue of ready-to-run goroutines. The number of Ps typically equals the number of available CPU cores.
- G: Goroutine: A lightweight, independently executing function.
The scheduler works as follows:
-
Goroutine creation: When a
go
statement is encountered, a new goroutine (G) is created and placed on the run queue of a P. - Run queue: Each P maintains its own run queue. When a P is idle, it searches for runnable goroutines from its own queue.
- Work stealing: If a P's run queue is empty, it attempts to "steal" a goroutine from another P's run queue. This prevents thread starvation and ensures efficient CPU utilization.
- Context switching: The scheduler performs context switching between goroutines, allowing multiple goroutines to run concurrently on a single thread.
- Synchronization primitives: Go provides synchronization primitives (mutexes, channels, etc.) to coordinate access to shared resources among concurrent goroutines.
Optimizing code for the Go scheduler:
- Avoid excessive goroutine creation: Creating too many goroutines can overwhelm the scheduler and lead to performance degradation. Favor using goroutines strategically for truly concurrent tasks.
- Use appropriate synchronization primitives: Choose the right synchronization primitive for the task. Unnecessary locking can create bottlenecks.
- Balance work: Ensure that work is distributed evenly among goroutines. Uneven work distribution can lead to some goroutines being idle while others are overloaded.
- Consider using worker pools: For managing a large number of concurrent tasks, worker pools can be more efficient than creating a goroutine for each task. They limit the number of concurrently running goroutines, reducing scheduler overhead.
What Are Common Pitfalls to Avoid When Writing Concurrent Go Code, and How Does the Scheduler Relate to These Issues?
Several common pitfalls can arise when writing concurrent Go code:
- Race conditions: Occur when multiple goroutines access and modify shared resources concurrently without proper synchronization. The scheduler's role here is to interleave the execution of these goroutines in unpredictable ways, making race conditions difficult to detect and debug.
- Deadlocks: A deadlock occurs when two or more goroutines are blocked indefinitely, waiting for each other to release resources. The scheduler cannot resolve this; it simply reflects the program's logic flaw.
- Data races: A specific type of race condition where data is accessed concurrently without proper synchronization, leading to unpredictable behavior. The scheduler's non-deterministic execution order makes data races particularly insidious.
- Starvation: A goroutine might be unable to acquire necessary resources because other goroutines are constantly monopolizing them. While the scheduler tries to prevent this through work stealing, imbalanced work distribution can still lead to starvation.
- Leaky goroutines: Goroutines that never exit can consume system resources and potentially lead to memory leaks. The scheduler continues to manage these "zombie" goroutines, adding to overhead.
The scheduler is intimately related to these issues because its job is to manage the execution of goroutines. The scheduler's non-deterministic nature means that the order in which goroutines execute can vary, making race conditions and data races harder to reproduce and debug. Effective synchronization mechanisms are crucial to mitigate these problems, allowing the scheduler to manage concurrent execution safely and efficiently.
How Can I Profile My Go Application to Identify Bottlenecks Related to the Scheduler's Performance?
Profiling your Go application is crucial for identifying performance bottlenecks, including those related to the scheduler. The pprof
tool is a powerful built-in profiling tool in Go. You can use it to profile CPU usage, memory allocation, blocking profiles and more.
To profile your application:
-
Enable profiling: Use the
runtime/pprof
package within your Go code to enable profiling. You can profile CPU usage, memory allocation, and blocking profiles. - Run your application: Run your application under load to generate profiling data.
-
Generate profile data: Use the
pprof
commands to generate profile files. For example:go tool pprof <binary> cpu.prof # For CPU profiling go tool pprof <binary> mem.prof # For memory profiling go tool pprof <binary> block.prof # For blocking profile
Copy after login -
Analyze the profile: Use the
pprof
interactive tool to analyze the profile data. Look for functions that consume a significant portion of CPU time or memory. Blocking profiles can highlight goroutines waiting on synchronization primitives, indicating potential bottlenecks. - Interpret the results: High CPU usage in the scheduler itself or functions related to synchronization primitives can indicate scheduler-related bottlenecks. Memory leaks or excessive garbage collection can also indirectly impact scheduler performance.
By systematically analyzing these profiles, you can pinpoint areas of your code that are causing scheduler-related performance issues. Focus on optimizing these areas to improve overall application performance.
What Are Some Best Practices for Structuring Go Programs to Maximize the Efficiency of the Go Scheduler, Especially in Highly Concurrent Scenarios?
Structuring your Go programs effectively is crucial for maximizing scheduler efficiency, particularly in highly concurrent scenarios. Here are some best practices:
- Use goroutines judiciously: Don't overuse goroutines. Create them only when necessary for true concurrency. Overusing goroutines can overwhelm the scheduler and lead to context switching overhead.
- Worker pools: For managing a large number of concurrent tasks, worker pools provide a controlled way to limit the number of concurrently running goroutines, preventing the scheduler from being overloaded.
- Efficient synchronization: Choose appropriate synchronization primitives (channels, mutexes, sync.WaitGroup, etc.) and use them correctly. Avoid unnecessary locking, which can create bottlenecks. Consider using channels for communication and synchronization whenever possible, as they often provide better performance and readability than mutexes.
- Non-blocking operations: Prefer non-blocking operations whenever possible. Blocking operations can stall goroutines and impact scheduler performance.
-
Context cancellation: Use the
context
package to propagate cancellation signals to goroutines, allowing them to gracefully exit when no longer needed. This prevents leaked goroutines and improves resource utilization. - Minimize shared resources: Reduce the number of shared resources accessed concurrently to minimize contention and improve performance.
- Benchmark and profile: Regularly benchmark and profile your application to identify performance bottlenecks and optimize your code accordingly.
- Consider using goroutine pools: Pre-allocate a pool of goroutines to reuse them for multiple tasks, reducing the overhead of creating and destroying goroutines.
By following these best practices, you can structure your Go programs to effectively utilize the scheduler and achieve optimal performance, even in highly concurrent environments. Remember that continuous monitoring and profiling are crucial for identifying and addressing potential bottlenecks.
The above is the detailed content of How does the Go scheduler work and how can I optimize my code for it?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


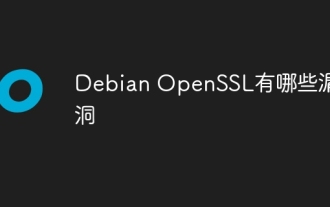
OpenSSL, as an open source library widely used in secure communications, provides encryption algorithms, keys and certificate management functions. However, there are some known security vulnerabilities in its historical version, some of which are extremely harmful. This article will focus on common vulnerabilities and response measures for OpenSSL in Debian systems. DebianOpenSSL known vulnerabilities: OpenSSL has experienced several serious vulnerabilities, such as: Heart Bleeding Vulnerability (CVE-2014-0160): This vulnerability affects OpenSSL 1.0.1 to 1.0.1f and 1.0.2 to 1.0.2 beta versions. An attacker can use this vulnerability to unauthorized read sensitive information on the server, including encryption keys, etc.
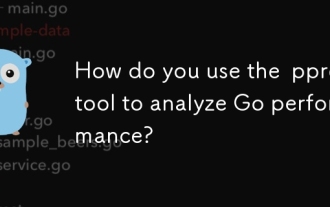
The article explains how to use the pprof tool for analyzing Go performance, including enabling profiling, collecting data, and identifying common bottlenecks like CPU and memory issues.Character count: 159
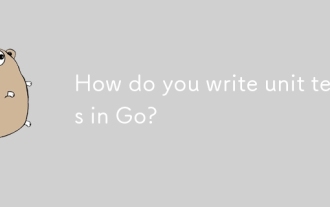
The article discusses writing unit tests in Go, covering best practices, mocking techniques, and tools for efficient test management.
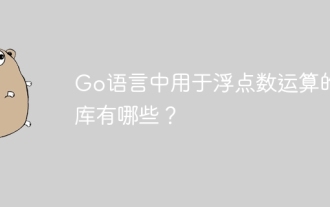
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
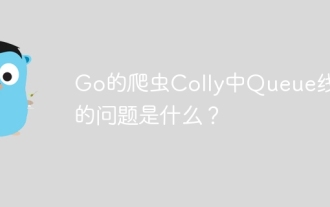
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
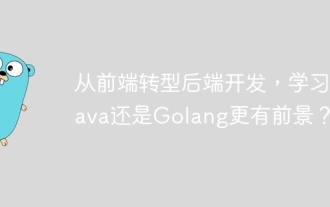
Backend learning path: The exploration journey from front-end to back-end As a back-end beginner who transforms from front-end development, you already have the foundation of nodejs,...
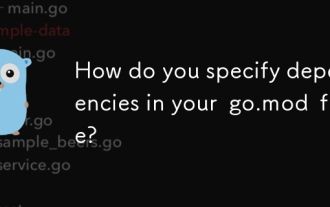
The article discusses managing Go module dependencies via go.mod, covering specification, updates, and conflict resolution. It emphasizes best practices like semantic versioning and regular updates.
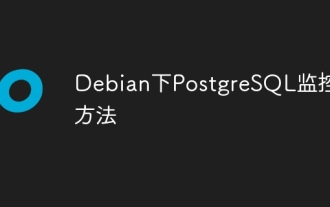
This article introduces a variety of methods and tools to monitor PostgreSQL databases under the Debian system, helping you to fully grasp database performance monitoring. 1. Use PostgreSQL to build-in monitoring view PostgreSQL itself provides multiple views for monitoring database activities: pg_stat_activity: displays database activities in real time, including connections, queries, transactions and other information. pg_stat_replication: Monitors replication status, especially suitable for stream replication clusters. pg_stat_database: Provides database statistics, such as database size, transaction commit/rollback times and other key indicators. 2. Use log analysis tool pgBadg
