How to Use Conditional Statements (if, else) in Python?
How to Use Conditional Statements (if, else) in Python?
Conditional statements in Python, primarily if
, elif
(else if), and else
, control the flow of your program based on whether certain conditions are true or false. They allow you to execute different blocks of code depending on the outcome of these conditions.
Basic Structure:
The basic structure of an if
statement is:
if condition: # Code to execute if the condition is True
If you want to handle multiple conditions, you can use elif
:
if condition1: # Code to execute if condition1 is True elif condition2: # Code to execute if condition1 is False and condition2 is True else: # Code to execute if neither condition1 nor condition2 is True
Example:
x = 10 if x > 5: print("x is greater than 5") elif x == 5: print("x is equal to 5") else: print("x is less than 5")
In this example, the output would be "x is greater than 5" because the first condition (x > 5
) is true. The elif
and else
blocks are skipped. Python evaluates the conditions sequentially; it stops as soon as it finds a true condition.
What are the common pitfalls to avoid when using if/else statements in Python?
Several common mistakes can occur when working with if/else
statements:
- Incorrect Indentation: Python relies heavily on indentation to define code blocks. Incorrect indentation will lead to
IndentationError
and incorrect logic. Always maintain consistent indentation (usually four spaces) within yourif
,elif
, andelse
blocks. - Confusing
=
and==
:=
is the assignment operator, while==
is the equality operator. Using=
in a conditional statement will assign a value instead of comparing, often leading to unexpected behavior. For example,if x = 5:
is incorrect; it should beif x == 5:
. - Unnecessary Nesting: Deeply nested
if/else
statements can become difficult to read and maintain. Consider refactoring your code to use simpler structures, such as functions or logical operators, to improve readability and reduce complexity. - Missing
else
orelif
: Sometimes, you might forget to include anelse
block to handle cases where none of the preceding conditions are true. This can lead to unexpected behavior or errors if your code doesn't account for all possible scenarios. - Boolean Logic Errors: Incorrect use of logical operators (
and
,or
,not
) can lead to incorrect evaluation of conditions. Double-check your boolean expressions for accuracy. For instance,if x > 5 and x < 10
correctly checks if x is between 5 and 10, butif x > 5 or x < 10
is always true because x will always satisfy at least one of the conditions. - Mutable Default Arguments: Avoid using mutable objects (like lists or dictionaries) as default arguments in functions that use conditional statements. This can lead to unexpected behavior due to the default argument being modified within the function's scope.
How can I nest conditional statements effectively in Python for complex logic?
Nesting conditional statements involves placing one if
, elif
, or else
block inside another. While this is necessary for complex logic, it's crucial to do it effectively to maintain readability and avoid errors.
Strategies for Effective Nesting:
- Keep it Flat: Try to minimize nesting levels. Deeply nested structures quickly become difficult to understand. If you find yourself with many nested levels, consider refactoring your code into smaller, more manageable functions.
- Use Early Exits: If a condition is met early in a nested structure, exit the nested structure using
return
,break
, orcontinue
to avoid unnecessary further checks. This simplifies the logic and improves readability. - Meaningful Variable Names: Use clear and descriptive variable names to enhance readability. This is especially important in nested structures where the context might not be immediately obvious.
- Comments: Add comments to explain the purpose of each nested block. This helps other developers (and your future self) understand the logic.
Example:
if condition: # Code to execute if the condition is True
This example shows a simple nested if
statement. In more complex scenarios, consider breaking down the logic into smaller functions to improve readability.
Can I use conditional statements with different data types in Python, and if so, how?
Yes, you can use conditional statements with different data types in Python. However, you need to be aware of how Python handles comparisons between different types.
- Numeric Comparisons: Comparisons between numbers (integers, floats) are straightforward. Python will perform the expected numerical comparisons.
- String Comparisons: Strings are compared lexicographically (based on their alphabetical order).
- Boolean Comparisons: Booleans (
True
andFalse
) are compared directly.True
is considered greater thanFalse
. - Type Comparisons: You can check the data type of a variable using the
type()
function and compare it to other types using==
. - Implicit Type Coercion: In some cases, Python might attempt to implicitly convert data types for comparison. For example, comparing a string that represents a number to an integer might work (but is generally discouraged for clarity). However, this can lead to unexpected results, so explicit type conversion is recommended for clarity and reliability.
Example:
if condition1: # Code to execute if condition1 is True elif condition2: # Code to execute if condition1 is False and condition2 is True else: # Code to execute if neither condition1 nor condition2 is True
Remember that implicit type conversions can lead to unexpected results. Explicit type conversion using functions like int()
, float()
, str()
is generally preferred for clarity and to avoid potential errors. Always be mindful of the data types you're comparing to ensure your conditional statements behave as expected.
The above is the detailed content of How to Use Conditional Statements (if, else) in Python?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
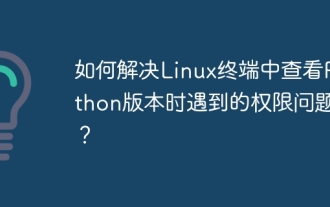
Solution to permission issues when viewing Python version in Linux terminal When you try to view Python version in Linux terminal, enter python...
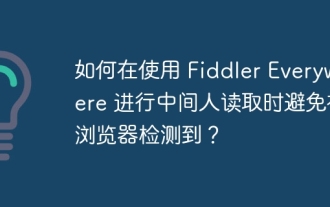
How to avoid being detected when using FiddlerEverywhere for man-in-the-middle readings When you use FiddlerEverywhere...
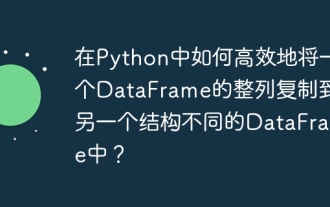
When using Python's pandas library, how to copy whole columns between two DataFrames with different structures is a common problem. Suppose we have two Dats...
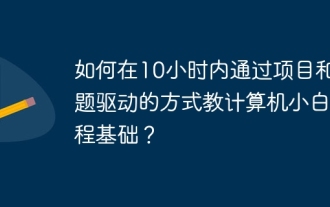
How to teach computer novice programming basics within 10 hours? If you only have 10 hours to teach computer novice some programming knowledge, what would you choose to teach...
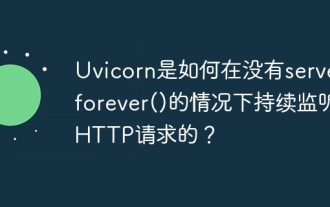
How does Uvicorn continuously listen for HTTP requests? Uvicorn is a lightweight web server based on ASGI. One of its core functions is to listen for HTTP requests and proceed...
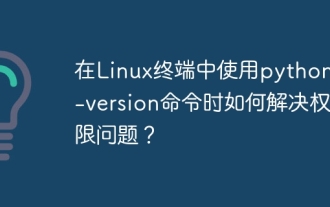
Using python in Linux terminal...
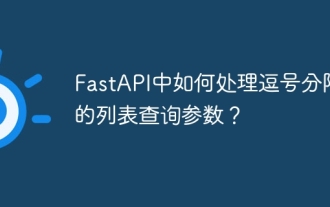
Fastapi ...
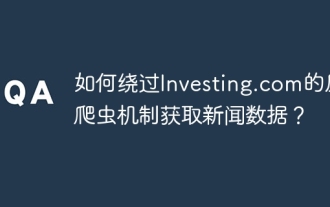
Understanding the anti-crawling strategy of Investing.com Many people often try to crawl news data from Investing.com (https://cn.investing.com/news/latest-news)...
