What are Python Modules and How Do I Import Them?
What are Python Modules and How Do I Import Them?
Python modules are files containing Python code, typically organized into functions, classes, and variables. They provide a way to structure and reuse code, promoting modularity and maintainability. Think of them as pre-built LEGO bricks – you can use them to construct larger, more complex programs without having to build every single piece from scratch. This reusability is a cornerstone of efficient and organized Python development.
Importing a module brings its contents into your current program's namespace. The primary way to do this is using the import
statement. For example, to import the math
module, which contains mathematical functions, you would write:
import math result = math.sqrt(25) # Use the sqrt function from the math module print(result) # Output: 5.0
You can also import specific functions or classes from a module using the from...import
statement:
from math import sqrt, pi result = sqrt(16) # No need to prefix with math. print(result) # Output: 4.0 print(pi) # Output: 3.141592653589793
Finally, you can import a module with an alias using the as
keyword:
import math as m result = m.sin(m.pi/2) # Use 'm' as a shorter alias for math print(result) # Output: 1.0
What are some common Python modules and their uses?
Python boasts a vast standard library, offering a wide array of modules for various tasks. Here are a few common ones:
math
: Provides mathematical functions likesqrt
,sin
,cos
,log
, etc. Useful for scientific computing, data analysis, and game development.os
: Offers functions for interacting with the operating system, such as creating directories, reading files, and managing processes. Essential for tasks involving file system manipulation.random
: Generates pseudo-random numbers. Used in simulations, games, and cryptography.datetime
: Provides classes for working with dates and times. Crucial for handling time-related data in applications.sys
: Provides access to system-specific parameters and functions. Useful for interacting with the Python interpreter itself.requests
: (Not part of the standard library; needs to be installed separately usingpip install requests
) A powerful library for making HTTP requests, crucial for interacting with web APIs and fetching data from the internet.json
: Handles JSON data encoding and decoding. Essential for working with web APIs and data interchange.re
: Provides support for regular expressions, enabling powerful pattern matching in strings. Used for data cleaning, text processing, and parsing.
How can I create my own Python module?
Creating your own module is straightforward. Simply write your Python code in a file (e.g., my_module.py
), and save it. This file then becomes your module. Let's say my_module.py
contains:
import math result = math.sqrt(25) # Use the sqrt function from the math module print(result) # Output: 5.0
Now, you can import and use it in another Python file:
from math import sqrt, pi result = sqrt(16) # No need to prefix with math. print(result) # Output: 4.0 print(pi) # Output: 3.141592653589793
Remember to place my_module.py
in the same directory as your main script, or specify the correct path when importing. For larger projects, organizing modules into packages (directories containing multiple modules and an __init__.py
file) is highly recommended.
What are the best practices for importing and using Python modules in a large project?
In large projects, efficient and organized module importing is crucial for maintainability and performance. Here are some best practices:
-
Use explicit imports: Avoid wildcard imports (
from module import *
) as they can lead to naming conflicts and make code harder to understand. - Import modules at the top of your files: This improves readability and makes it easier to see all dependencies at a glance.
- Organize imports: Group imports alphabetically or by category (standard library modules, third-party modules, your own modules).
- Use descriptive aliases (when necessary): Shorten long module names with meaningful aliases to improve readability.
- Minimize imports: Only import the specific functions or classes you need. Importing entire modules can increase load times and memory usage, especially in large projects.
- Utilize packages: Organize related modules into packages to improve structure and prevent naming collisions.
- Consider using a virtual environment: This isolates project dependencies and prevents conflicts between different projects.
- Document your imports: Clearly document why you're using a specific module and its purpose within your code. This aids in understanding and maintenance. Using comments to explain the reason for importing specific modules is extremely beneficial.
By following these best practices, you can ensure your large Python projects remain well-organized, efficient, and easy to maintain.
The above is the detailed content of What are Python Modules and How Do I Import Them?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
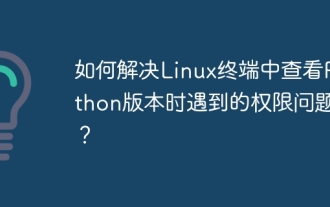
Solution to permission issues when viewing Python version in Linux terminal When you try to view Python version in Linux terminal, enter python...
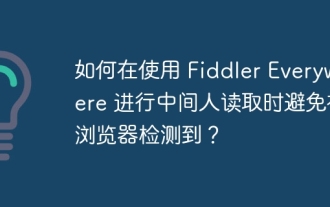
How to avoid being detected when using FiddlerEverywhere for man-in-the-middle readings When you use FiddlerEverywhere...
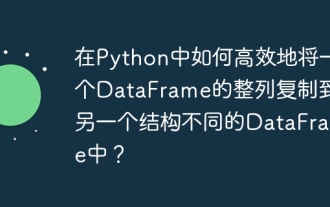
When using Python's pandas library, how to copy whole columns between two DataFrames with different structures is a common problem. Suppose we have two Dats...
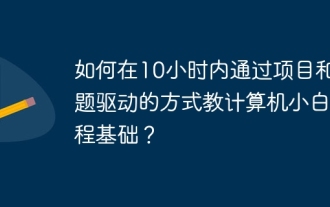
How to teach computer novice programming basics within 10 hours? If you only have 10 hours to teach computer novice some programming knowledge, what would you choose to teach...
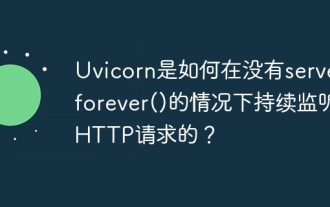
How does Uvicorn continuously listen for HTTP requests? Uvicorn is a lightweight web server based on ASGI. One of its core functions is to listen for HTTP requests and proceed...
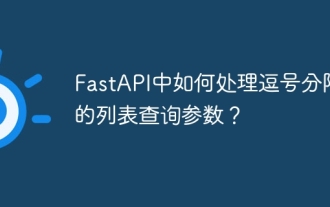
Fastapi ...
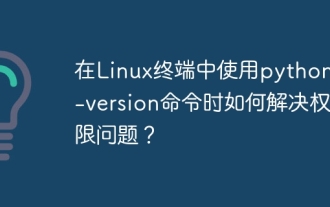
Using python in Linux terminal...
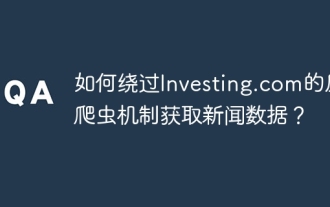
Understanding the anti-crawling strategy of Investing.com Many people often try to crawl news data from Investing.com (https://cn.investing.com/news/latest-news)...
