What are the best practices for working with large datasets in Go?
Best Practices for Working with Large Datasets in Go
Working with large datasets in Go requires careful planning and the utilization of efficient techniques to avoid memory exhaustion and performance bottlenecks. Here are some best practices:
- Chunking: Instead of loading the entire dataset into memory at once, process it in smaller, manageable chunks. Read data from disk or a database in batches, process each chunk, and then discard it before loading the next. The optimal chunk size will depend on your available RAM and the nature of your data. Experimentation is key to finding the sweet spot. This minimizes memory usage significantly.
-
Data Streaming: Leverage streaming techniques where possible. Libraries like
bufio
can help read and process data in streams, avoiding the need to hold the entire dataset in memory. This is particularly useful for datasets that are too large to fit in RAM. -
Efficient Data Structures: Choose data structures appropriate for your task. If you need to perform frequent lookups, consider using a hash map (
map[string]interface{}
). For sorted data where range queries are common, a sorted slice or a more sophisticated data structure might be more efficient. Avoid unnecessary allocations and data copying. -
Memory Profiling: Use Go's built-in profiling tools (
go test -bench=. -cpuprofile cpu.prof -memprofile mem.prof
) to identify memory leaks or areas of high memory consumption. This helps pinpoint inefficiencies in your code. Tools likepprof
allow visualization and analysis of these profiles. - Data Serialization: Consider using efficient serialization formats like Protocol Buffers or FlatBuffers for compact storage and fast data transfer. These formats are generally more compact than JSON or XML, reducing I/O overhead.
Efficiently Processing Terabyte-Sized Datasets in Go Without Running Out of Memory
Processing terabyte-sized datasets in Go without exceeding memory limits demands a strategic approach focused on minimizing memory footprint and leveraging external storage:
- Out-of-Core Processing: For datasets exceeding available RAM, out-of-core processing is essential. This involves reading and processing data in chunks from disk or a database, writing intermediate results to disk as needed, and only keeping a small portion of the data in memory at any given time.
- Database Integration: Utilize a database (like PostgreSQL, MySQL, or a NoSQL database like MongoDB) to store and manage the large dataset. Go's database/sql package provides a convenient interface for interacting with databases. This offloads the burden of managing the data to the database system.
- Data Partitioning: Divide the dataset into smaller, independent partitions. Each partition can then be processed concurrently, reducing the memory requirements for each individual process.
- External Sorting: For tasks requiring sorted data, employ external sorting algorithms that operate on disk instead of in memory. These algorithms read chunks of data from disk, sort them, and merge the sorted chunks to produce a fully sorted result.
- Memory-Mapped Files: For read-only datasets, memory-mapped files can provide efficient access without loading the entire file into RAM. The operating system handles paging, allowing access to data on demand.
Common Go Libraries or Tools Optimized for Handling Large Datasets and Improving Performance
Several Go libraries and tools are designed to streamline the handling of large datasets and enhance performance:
-
bufio
package: Provides buffered I/O operations for efficient reading and writing of data, minimizing disk access. -
encoding/gob
package: Offers efficient binary encoding and decoding for Go data structures, reducing serialization overhead compared to text-based formats like JSON. -
database/sql
package: Facilitates interaction with various database systems, allowing for efficient storage and retrieval of large datasets. -
sync
package: Provides synchronization primitives (mutexes, channels, etc.) for managing concurrent access to shared resources when parallelizing data processing. -
Third-party libraries: Libraries like
go-fastcsv
for CSV processing,parquet-go
for Parquet file handling, and various libraries for database interactions (e.g., database drivers for specific databases) can significantly improve efficiency.
Strategies to Parallelize the Processing of Large Datasets in Go for Faster Results
Parallelization is crucial for accelerating the processing of large datasets. Go's concurrency features make it well-suited for this task:
- Goroutines and Channels: Use goroutines to concurrently process different chunks of the dataset. Channels can facilitate communication between goroutines, allowing them to exchange data or signals.
- Worker Pools: Create a pool of worker goroutines to process data chunks concurrently. This limits the number of concurrently running goroutines, preventing excessive resource consumption.
- Data Partitioning (revisited): Divide the dataset into partitions, and assign each partition to a separate goroutine for parallel processing.
- MapReduce Pattern: Implement a MapReduce-style approach, where the "map" phase processes individual data elements in parallel, and the "reduce" phase aggregates the results.
- Parallel Libraries: Explore parallel processing libraries specifically designed for Go, which might offer optimized implementations of common parallel algorithms. Careful consideration of data dependencies and synchronization mechanisms is crucial to avoid race conditions and ensure correct results. Benchmarking different parallelization strategies is crucial to identify the most effective approach for a specific dataset and processing task.
The above is the detailed content of What are the best practices for working with large datasets in Go?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
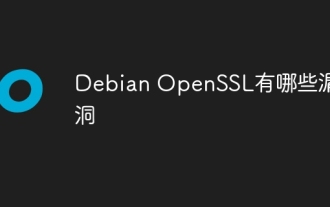
OpenSSL, as an open source library widely used in secure communications, provides encryption algorithms, keys and certificate management functions. However, there are some known security vulnerabilities in its historical version, some of which are extremely harmful. This article will focus on common vulnerabilities and response measures for OpenSSL in Debian systems. DebianOpenSSL known vulnerabilities: OpenSSL has experienced several serious vulnerabilities, such as: Heart Bleeding Vulnerability (CVE-2014-0160): This vulnerability affects OpenSSL 1.0.1 to 1.0.1f and 1.0.2 to 1.0.2 beta versions. An attacker can use this vulnerability to unauthorized read sensitive information on the server, including encryption keys, etc.
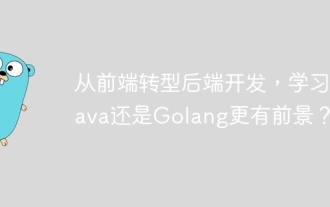
Backend learning path: The exploration journey from front-end to back-end As a back-end beginner who transforms from front-end development, you already have the foundation of nodejs,...
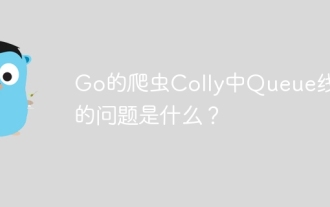
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
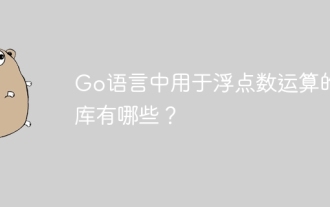
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
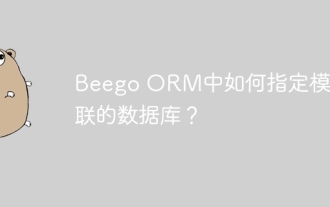
Under the BeegoORM framework, how to specify the database associated with the model? Many Beego projects require multiple databases to be operated simultaneously. When using Beego...
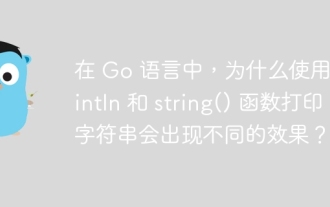
The difference between string printing in Go language: The difference in the effect of using Println and string() functions is in Go...
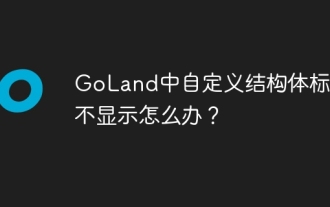
What should I do if the custom structure labels in GoLand are not displayed? When using GoLand for Go language development, many developers will encounter custom structure tags...
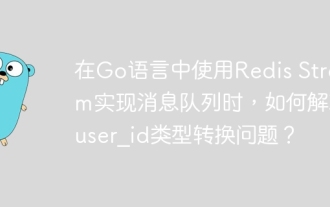
The problem of using RedisStream to implement message queues in Go language is using Go language and Redis...
