How Can I Reduce the Memory Footprint of My PHP Application?
How Can I Reduce the Memory Footprint of My PHP Application?
Reducing the memory footprint of your PHP application involves a multi-pronged approach focusing on code optimization, efficient data handling, and leveraging appropriate tools. There's no single magic bullet, but a combination of strategies will yield the best results. Here's a breakdown of key techniques:
1. Efficient Data Structures: Choosing the right data structure is crucial. Arrays in PHP are versatile but can become memory-intensive if they grow excessively large. Consider using alternative structures like SplFixedArray
for situations where you know the array size in advance. This avoids the overhead of dynamic resizing. For key-value storage, consider using SplObjectStorage
for objects or WeakMap
(available in PHP 7.4 and later) for weak references to avoid memory leaks.
2. Optimize Database Interactions: Database queries are a major source of memory consumption. Fetch only the necessary data using appropriate SELECT
statements with LIMIT
clauses. Avoid fetching entire tables into memory unless absolutely necessary. Utilize database caching mechanisms (e.g., Redis, Memcached) to reduce the load on the database and minimize repeated queries. Use prepared statements to prevent query compilation overhead.
3. Effective String Manipulation: String operations can be memory-intensive. Avoid unnecessary string concatenations, especially within loops. Instead, use sprintf()
or similar functions for efficient string formatting. Consider using mb_substr()
for multi-byte character string manipulation to prevent unexpected memory issues.
4. Garbage Collection Awareness: While PHP's garbage collector (GC) handles memory cleanup automatically, understanding its behavior can help. Avoid creating excessively large objects that linger unnecessarily. Break down large tasks into smaller, manageable chunks to allow the GC to work more effectively. Using techniques like object cloning judiciously can minimize unnecessary duplication.
5. Utilize OPcache: OPcache stores precompiled bytecode, reducing the overhead of parsing and compiling scripts on each request. This can significantly improve performance and indirectly reduce memory usage by minimizing the resources needed for script execution.
6. Proper Error Handling and Resource Management: Ensure proper closure of database connections, file handles, and other resources using finally
blocks or similar constructs. Handle exceptions gracefully to prevent resource leaks.
What are the common causes of high memory consumption in PHP applications?
High memory consumption in PHP applications often stems from several common culprits:
1. Memory Leaks: These occur when objects or resources are allocated but not properly released, leading to a gradual increase in memory usage over time. This is especially problematic in long-running applications or scripts processing large datasets.
2. Inefficient Algorithms and Data Structures: Poorly designed algorithms or the use of inappropriate data structures can lead to excessive memory allocation. For instance, using nested loops inefficiently or choosing arrays when a more efficient structure like a hash map would suffice.
3. Unoptimized Database Queries: Fetching large datasets without proper filtering or limiting can overwhelm memory. Queries that return entire tables or unnecessary columns contribute significantly to high memory usage.
4. Large Images and Files: Handling large images or files without proper buffering or streaming can lead to memory exhaustion. Process these assets in chunks instead of loading them entirely into memory.
5. Unclosed Resources: Failing to close database connections, file handles, or other resources after use prevents the system from reclaiming the associated memory.
6. Unintentional Object Duplication: Creating unnecessary copies of objects or large data structures without need increases memory consumption.
7. Recursive Functions without Proper Termination Conditions: Recursive functions without a proper base case can lead to stack overflow errors, a form of memory exhaustion.
How can I optimize my PHP code to use less memory?
Optimizing PHP code for lower memory usage involves careful consideration of several aspects:
1. Code Profiling and Analysis: Use profiling tools (see the next section) to identify memory bottlenecks and hotspots in your code. This provides data-driven insights into areas needing improvement.
2. Reduce Object Creation: Minimize the creation of unnecessary objects, particularly large ones. Reuse objects where possible instead of repeatedly creating new ones.
3. Avoid Unnecessary Variable Assignments: Don't assign large datasets to variables unless absolutely necessary. Stream data directly from sources (e.g., databases or files) when feasible.
4. Utilize Generators: Generators allow you to produce data iteratively, reducing the need to hold entire datasets in memory at once. This is particularly useful for processing large datasets.
5. Employ Caching Strategies: Implement caching mechanisms to store frequently accessed data in memory, reducing the need for repeated computations or database queries.
6. Optimize Loops: Optimize loops to minimize iterations and reduce redundant calculations. Use appropriate data structures and algorithms to enhance efficiency.
7. Refactor Large Functions: Break down large functions into smaller, more manageable units. This improves readability, maintainability, and can help the garbage collector work more effectively.
What tools and techniques can help me profile and identify memory leaks in your PHP application?
Several tools and techniques can assist in profiling and identifying memory leaks:
1. Xdebug: Xdebug is a powerful debugging and profiling tool for PHP. It provides detailed memory usage information, allowing you to pinpoint memory-intensive parts of your code. Its profiling capabilities enable you to track memory allocation and deallocation over time.
2. Blackfire.io: This is a commercial profiling service that provides detailed performance and memory usage analysis. It can identify bottlenecks and memory leaks effectively.
3. Memory Profilers: Several memory profilers are available, either integrated into IDEs or as standalone tools. These tools provide insights into memory allocation, object sizes, and potential leaks.
4. Memory Limit Settings: Experiment with setting a lower memory limit in your PHP configuration (memory_limit
directive in php.ini
). This can help expose memory leaks sooner, as they will lead to fatal errors when the limit is reached.
5. Valgrind (for extensions): If you're working with PHP extensions written in C/C , Valgrind can be invaluable in detecting memory leaks within the extension code itself.
6. Manual Logging: In some cases, adding strategic logging statements to track object creation and destruction can provide insights into potential memory leaks. This approach is less automated but can be helpful for targeted investigations.
By combining these tools and techniques with careful code optimization, you can significantly reduce the memory footprint of your PHP application and improve its performance and stability. Remember that a proactive approach, including regular profiling and attention to memory management best practices, is key to preventing and resolving memory-related issues.
The above is the detailed content of How Can I Reduce the Memory Footprint of My PHP Application?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


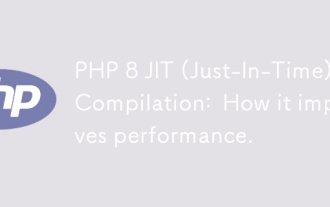
PHP 8's JIT compilation enhances performance by compiling frequently executed code into machine code, benefiting applications with heavy computations and reducing execution times.
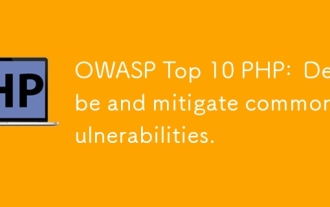
The article discusses OWASP Top 10 vulnerabilities in PHP and mitigation strategies. Key issues include injection, broken authentication, and XSS, with recommended tools for monitoring and securing PHP applications.
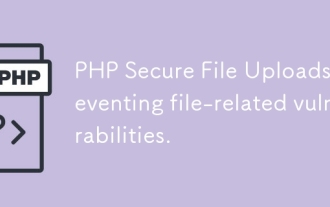
The article discusses securing PHP file uploads to prevent vulnerabilities like code injection. It focuses on file type validation, secure storage, and error handling to enhance application security.
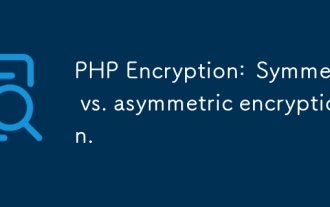
The article discusses symmetric and asymmetric encryption in PHP, comparing their suitability, performance, and security differences. Symmetric encryption is faster and suited for bulk data, while asymmetric is used for secure key exchange.

The article discusses implementing robust authentication and authorization in PHP to prevent unauthorized access, detailing best practices and recommending security-enhancing tools.
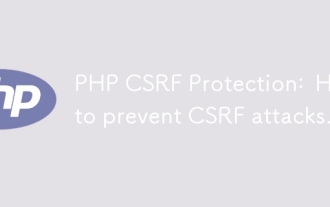
The article discusses strategies to prevent CSRF attacks in PHP, including using CSRF tokens, Same-Site cookies, and proper session management.

Prepared statements in PHP enhance database security and efficiency by preventing SQL injection and improving query performance through compilation and reuse.Character count: 159
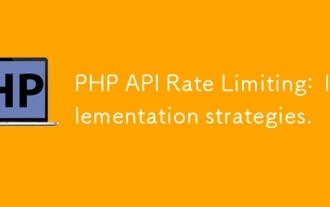
The article discusses strategies for implementing API rate limiting in PHP, including algorithms like Token Bucket and Leaky Bucket, and using libraries like symfony/rate-limiter. It also covers monitoring, dynamically adjusting rate limits, and hand
