How to Implement Drag and Drop Functionality in HTML5?
This article explains HTML5 drag-and-drop implementation. It details the dragstart, dragover, dragenter, dragleave, and drop events, emphasizing event.preventDefault() for drop functionality. Customizing visual feedback using dataTransfer.setDrag
How to Implement Drag and Drop Functionality in HTML5?
Implementing drag and drop functionality in HTML5 involves leveraging several events and attributes. The core process revolves around three main stages: initiating the drag, performing the drag, and dropping the dragged element.
1. Making an element draggable: You need to set the draggable
attribute of the HTML element you want to make draggable to true
. For example: <div id="myElement" draggable="true">Drag me!</div>
.
2. Handling the drag events: Several events are crucial for managing the drag operation:
-
dragstart
: This event fires when the user starts dragging the element. Here, you'll typically set the data to be transferred usingdataTransfer.setData()
. This data can be any string, often representing an ID or other relevant information about the dragged element. You might also want to set a custom drag image usingdataTransfer.setDragImage()
. This allows you to display a different visual representation of the dragged item during the drag operation. -
dragover
: This event fires repeatedly while the element is being dragged over a potential drop target. Crucially, you must callevent.preventDefault()
within thedragover
event handler to allow the drop to occur. Without this, the drop will be prevented by default browser behavior. -
dragenter
: This event fires when the dragged element enters a potential drop target. You can use this to provide visual feedback, such as highlighting the drop target. -
dragleave
: This event fires when the dragged element leaves a potential drop target. Use this to revert any visual feedback applied in thedragenter
event. -
drop
: This event fires when the user releases the mouse button over a drop target. Here, you'll retrieve the data transferred usingdataTransfer.getData()
and perform the necessary actions, such as moving the element or updating the application state.
3. Setting up drop targets: The elements where you want to allow dropping need to have event listeners attached to handle the dragover
, dragenter
, dragleave
, and drop
events. Remember, event.preventDefault()
is crucial in the dragover
handler to enable dropping.
Here's a simplified example:
const draggableElement = document.getElementById('myElement'); const dropTarget = document.getElementById('dropZone'); draggableElement.addEventListener('dragstart', (event) => { event.dataTransfer.setData('text/plain', draggableElement.id); }); dropTarget.addEventListener('dragover', (event) => { event.preventDefault(); }); dropTarget.addEventListener('drop', (event) => { event.preventDefault(); const data = event.dataTransfer.getData('text/plain'); const draggedElement = document.getElementById(data); dropTarget.appendChild(draggedElement); });
Remember to include appropriate HTML elements with IDs myElement
and dropZone
. This is a basic example; more sophisticated implementations will require error handling and more robust data management.
What are the key browser compatibility considerations for HTML5 drag and drop?
While HTML5 drag and drop is widely supported, some minor inconsistencies exist across browsers. Key considerations include:
- Event handling nuances: The exact behavior of events like
dragenter
anddragover
can subtly differ between browsers. Thorough testing across major browsers (Chrome, Firefox, Safari, Edge) is essential to ensure consistent functionality. - Data transfer limitations: The type and size of data that can be transferred via
dataTransfer.setData()
might have browser-specific limitations. For large data transfers, consider alternative approaches. - Visual feedback differences: The default visual feedback (e.g., the cursor appearance) can vary. To maintain consistent visual cues, you'll likely need to customize the feedback using
dataTransfer.setDragImage()
as described above. - Older browsers: For older browsers lacking full HTML5 support, you might need to implement fallback mechanisms using JavaScript libraries or other techniques to provide drag-and-drop functionality. Feature detection can help determine which methods to use based on browser capabilities.
How can I customize the visual feedback during a drag and drop operation in HTML5?
Customizing visual feedback significantly improves the user experience. The primary method is using dataTransfer.setDragImage()
. This allows you to specify a custom image to represent the dragged element during the drag operation.
draggableElement.addEventListener('dragstart', (event) => { event.dataTransfer.setData('text/plain', draggableElement.id); // Create a custom drag image const img = new Image(); img.src = 'custom_drag_image.png'; // Replace with your image path event.dataTransfer.setDragImage(img, 0, 0); // 0, 0 sets the image's offset });
In this example, custom_drag_image.png
would be displayed as the drag image. Adjusting the x
and y
coordinates (0, 0 in this case) allows you to position the image relative to the cursor.
Beyond setDragImage()
, you can enhance visual feedback by:
-
Modifying CSS classes: Add or remove CSS classes from drop targets based on
dragenter
anddragleave
events to change their appearance (e.g., highlighting on hover). - Using visual cues: Display temporary elements (e.g., a "drop here" message) near the drop target to guide the user.
- Providing progress indicators: For long drag-and-drop operations, show a progress bar to keep the user informed.
What are some common use cases for implementing drag and drop functionality in HTML5 applications?
HTML5 drag and drop offers a highly intuitive way to interact with web applications. Common use cases include:
- File uploads: Allowing users to drag and drop files directly into a web form for uploading simplifies the file submission process.
- Reordering items: Drag and drop is ideal for rearranging items in lists, such as tasks in a to-do list or files in a file manager.
- Interactive canvases: In applications involving visual editing (e.g., image editors, diagramming tools), drag and drop enables moving and manipulating elements on the canvas.
- Building interactive dashboards: Drag and drop allows users to customize dashboards by moving widgets and rearranging their layout.
- Creating interactive games: Drag and drop mechanics can be used to create various game interactions, such as moving game pieces or placing objects in a game world.
- Building visual editors: Many visual editors (like WYSIWYG editors) utilize drag and drop for adding images, videos, and other content.
These are just a few examples; the versatility of HTML5 drag and drop makes it a valuable tool for creating engaging and user-friendly web applications across diverse domains.
The above is the detailed content of How to Implement Drag and Drop Functionality in HTML5?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


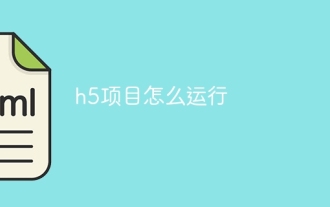
Running the H5 project requires the following steps: installing necessary tools such as web server, Node.js, development tools, etc. Build a development environment, create project folders, initialize projects, and write code. Start the development server and run the command using the command line. Preview the project in your browser and enter the development server URL. Publish projects, optimize code, deploy projects, and set up web server configuration.
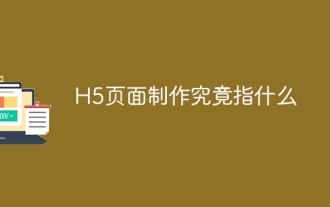
H5 page production refers to the creation of cross-platform compatible web pages using technologies such as HTML5, CSS3 and JavaScript. Its core lies in the browser's parsing code, rendering structure, style and interactive functions. Common technologies include animation effects, responsive design, and data interaction. To avoid errors, developers should be debugged; performance optimization and best practices include image format optimization, request reduction and code specifications, etc. to improve loading speed and code quality.
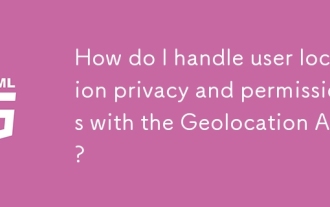
The article discusses managing user location privacy and permissions using the Geolocation API, emphasizing best practices for requesting permissions, ensuring data security, and complying with privacy laws.
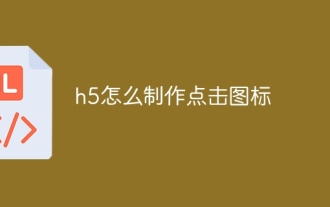
The steps to create an H5 click icon include: preparing a square source image in the image editing software. Add interactivity in the H5 editor and set the click event. Create a hotspot that covers the entire icon. Set the action of click events, such as jumping to the page or triggering animation. Export H5 documents as HTML, CSS, and JavaScript files. Deploy the exported files to a website or other platform.
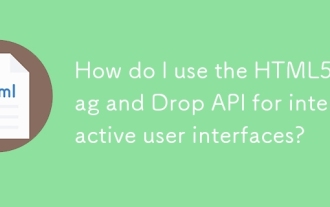
The article explains how to use the HTML5 Drag and Drop API to create interactive user interfaces, detailing steps to make elements draggable, handle key events, and enhance user experience with custom feedback. It also discusses common pitfalls to a
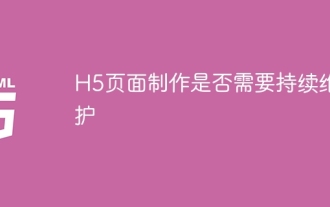
The H5 page needs to be maintained continuously, because of factors such as code vulnerabilities, browser compatibility, performance optimization, security updates and user experience improvements. Effective maintenance methods include establishing a complete testing system, using version control tools, regularly monitoring page performance, collecting user feedback and formulating maintenance plans.
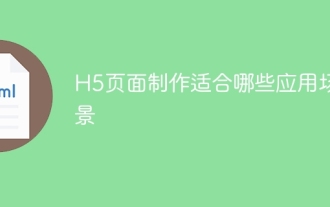
H5 (HTML5) is suitable for lightweight applications, such as marketing campaign pages, product display pages and corporate promotion micro-websites. Its advantages lie in cross-platformity and rich interactivity, but its limitations lie in complex interactions and animations, local resource access and offline capabilities.
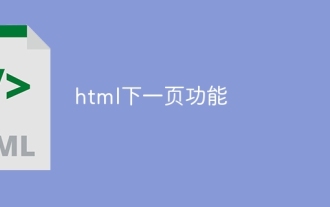
<p>The next page function can be created through HTML. The steps include: creating container elements, splitting content, adding navigation links, hiding other pages, and adding scripts. This feature allows users to browse segmented content, displaying only one page at a time, and is suitable for displaying large amounts of data or content. </p>
