How to Use the HTML5 History API for Single-Page Applications?
This article explains the HTML5 History API for building Single-Page Applications (SPAs). It details pushState() and replaceState() for manipulating browser history without page reloads, emphasizing clean URLs and improved user experience. The arti
How to Use the HTML5 History API for Single-Page Applications?
The HTML5 History API provides a powerful way to manipulate the browser's history stack without performing full page reloads, a crucial feature for building smooth and responsive Single-Page Applications (SPAs). It primarily utilizes two methods: pushState()
and replaceState()
.
pushState()
adds a new state to the history stack. It takes three arguments:
-
state
: An object representing the state associated with the new history entry. This object is accessible via thepopstate
event. It's crucial to keep this object relatively small, as it's stored in the browser's memory. Often, you'll use it to store data relevant to the current view, like IDs or parameters. -
title
: A title for the history entry. Currently, most browsers ignore this value, though future browsers might utilize it. It's best practice to provide a descriptive title. -
url
: A URL representing the new state. This URL is reflected in the browser's address bar, but it doesn't necessarily trigger a page reload. This URL should be relative to the current page's location. The browser doesn't actually navigate to this URL unless the user uses the back/forward buttons or refreshes the page.
Example: history.pushState({ page: 1 }, 'Page 1', '/page1');
This adds a new history entry with the state { page: 1 }
, title 'Page 1', and URL '/page1'.
replaceState()
is similar to pushState()
, but instead of adding a new entry, it replaces the current entry in the history stack. This is useful for updating the URL without adding unnecessary entries to the history. Example: history.replaceState({ page: 2 }, 'Page 2', '/page2');
The popstate
event fires when the user navigates through the browser's history using the back or forward buttons, or when the page is restored from a bookmark. You need to listen for this event to update the SPA's content accordingly.
window.addEventListener('popstate', function(event) { if (event.state) { // Update the SPA based on event.state console.log("State:", event.state); renderPage(event.state.page); } });
This code snippet listens for the popstate
event and, if a state object exists, updates the SPA using a hypothetical renderPage
function.
What are the benefits of using the HTML5 History API over traditional methods for single-page application navigation?
Traditional methods for SPA navigation often involve manipulating the URL using hash fragments (#
) or relying solely on JavaScript to manage the application state. The HTML5 History API offers several key advantages:
- Clean URLs: The History API allows for clean, SEO-friendly URLs without hash fragments, improving the user experience and search engine indexing.
- Improved User Experience: By avoiding full page reloads, the History API provides a smoother, more responsive user experience.
- Better Browser Integration: The use of the browser's history stack provides a more natural and intuitive navigation experience, making the SPA feel more integrated with the browser.
- Enhanced State Management: The ability to associate data with each history entry simplifies state management within the SPA.
- Bookmarking and Sharing: URLs generated using the History API are bookmarkable and shareable, unlike URLs relying solely on hash fragments which often contain only fragments of the application state.
How can I handle browser back/forward button functionality correctly with the HTML5 History API in my SPA?
Correctly handling back/forward button functionality is crucial for a seamless user experience. The core of this is listening to the popstate
event, as described above.
When using pushState()
or replaceState()
, ensure that you're updating the UI to reflect the state associated with the new URL. This involves fetching the appropriate data, updating the DOM, and generally ensuring the application's state is consistent with the URL in the address bar.
Crucially, the popstate
event does not fire when the initial page loads or when pushState()
or replaceState()
is called directly. It only fires when the user interacts with the browser's back/forward buttons. Therefore, you'll likely need initial state handling separate from the popstate
event listener. This could involve checking the URL on page load and rendering the appropriate content.
What are some common pitfalls to avoid when implementing the HTML5 History API in a single-page application?
Several pitfalls can hinder the effective implementation of the History API:
-
Ignoring the
popstate
event: Failing to listen for thepopstate
event will result in the SPA not responding correctly to browser back/forward button interactions. -
Large state objects: Storing excessively large objects in the
state
parameter can negatively impact browser performance and memory usage. Keep the state object concise and relevant. - Inconsistent URL structure: Maintain a consistent and predictable URL structure to ensure proper navigation and bookmarking functionality.
- Not handling page reloads correctly: When a user refreshes the page, the browser will load the URL as it appears in the address bar. You need to handle this scenario appropriately, potentially re-fetching data and rendering the correct view based on the URL.
- Forgetting server-side routing: If your SPA interacts with a server, you need to implement server-side routing to handle requests for URLs generated by the History API. If the server doesn't recognize the URL, it might return a 404 error.
- Lack of error handling: Implement proper error handling to gracefully manage potential issues like network errors during data fetching. This helps prevent a broken user experience.
The above is the detailed content of How to Use the HTML5 History API for Single-Page Applications?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics




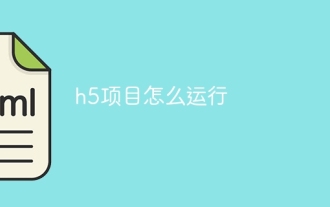
Running the H5 project requires the following steps: installing necessary tools such as web server, Node.js, development tools, etc. Build a development environment, create project folders, initialize projects, and write code. Start the development server and run the command using the command line. Preview the project in your browser and enter the development server URL. Publish projects, optimize code, deploy projects, and set up web server configuration.
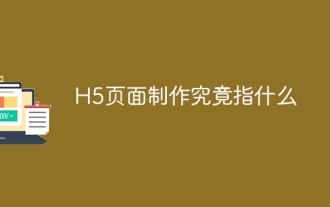
H5 page production refers to the creation of cross-platform compatible web pages using technologies such as HTML5, CSS3 and JavaScript. Its core lies in the browser's parsing code, rendering structure, style and interactive functions. Common technologies include animation effects, responsive design, and data interaction. To avoid errors, developers should be debugged; performance optimization and best practices include image format optimization, request reduction and code specifications, etc. to improve loading speed and code quality.
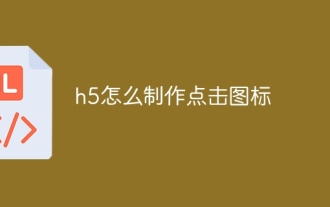
The steps to create an H5 click icon include: preparing a square source image in the image editing software. Add interactivity in the H5 editor and set the click event. Create a hotspot that covers the entire icon. Set the action of click events, such as jumping to the page or triggering animation. Export H5 documents as HTML, CSS, and JavaScript files. Deploy the exported files to a website or other platform.
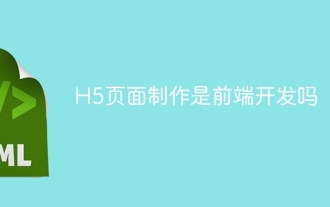
Yes, H5 page production is an important implementation method for front-end development, involving core technologies such as HTML, CSS and JavaScript. Developers build dynamic and powerful H5 pages by cleverly combining these technologies, such as using the <canvas> tag to draw graphics or using JavaScript to control interaction behavior.
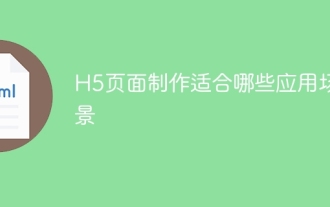
H5 (HTML5) is suitable for lightweight applications, such as marketing campaign pages, product display pages and corporate promotion micro-websites. Its advantages lie in cross-platformity and rich interactivity, but its limitations lie in complex interactions and animations, local resource access and offline capabilities.

H5 is not a standalone programming language, but a collection of HTML5, CSS3 and JavaScript for building modern web applications. 1. HTML5 defines the web page structure and content, and provides new tags and APIs. 2. CSS3 controls style and layout, and introduces new features such as animation. 3. JavaScript implements dynamic interaction and enhances functions through DOM operations and asynchronous requests.

H5referstoHTML5,apivotaltechnologyinwebdevelopment.1)HTML5introducesnewelementsandAPIsforrich,dynamicwebapplications.2)Itsupportsmultimediawithoutplugins,enhancinguserexperienceacrossdevices.3)SemanticelementsimprovecontentstructureandSEO.4)H5'srespo

H5 pop-up window creation steps: 1. Determine the triggering method (click, time, exit, scroll); 2. Design content (title, text, action button); 3. Set style (size, color, font, background); 4. Implement code (HTML, CSS, JavaScript); 5. Test and deployment.
