How to Optimize CSS for Performance in HTML5?
This article details CSS optimization techniques for improved HTML5 performance. Key strategies include minification, compression, caching, and efficient coding practices to reduce file size and render-blocking. It emphasizes avoiding common pitfal
How to Optimize CSS for Performance in HTML5?
Optimizing CSS for performance in HTML5 involves a multifaceted approach focusing on reducing file size, minimizing render-blocking, and efficient coding practices. This ensures faster page load times and a smoother user experience. Key strategies include:
- Minification: This process removes unnecessary characters like whitespace and comments from your CSS files, significantly reducing their size without affecting functionality. Many online tools and build processes (like those using Webpack or Gulp) automate this.
- Compression: Gzipping your CSS files before serving them to the browser drastically reduces the amount of data transferred. Most web servers support Gzip compression automatically, but you should verify its configuration.
-
Caching: Leverage browser caching by setting appropriate HTTP headers (like
Cache-Control
andExpires
) to allow browsers to store CSS files locally. This eliminates the need to download them repeatedly on subsequent visits. - Use a CSS Preprocessor: Tools like Sass or Less offer features like variables, nesting, and mixins, which can improve code organization and maintainability. These preprocessors compile into standard CSS, and the resulting CSS can be further optimized using minification and compression.
- Use CSS Frameworks Wisely: While frameworks like Bootstrap or Tailwind CSS offer convenience, they can also significantly increase your CSS file size. Only include the necessary components and consider customizing or trimming the framework to fit your project's specific needs. Alternatively, explore lighter-weight alternatives or build your own CSS library tailored to your application.
- Optimize Images Used in CSS: If you use images in your CSS (e.g., for backgrounds), ensure they are optimized for web use (appropriate format, compression, and size).
What are the best practices for minimizing CSS file size to improve page load speed?
Minimizing CSS file size is crucial for fast page load times. Beyond minification and compression (discussed above), several best practices contribute to smaller CSS files:
- Avoid Redundancy: Eliminate duplicate selectors and styles. Use CSS specificity to your advantage, avoiding overly general selectors that might cascade unnecessarily.
- Use Short Class Names: Shorter class names reduce the overall file size, although readability should remain a priority.
- Remove Unused CSS: Use tools (like browser developer tools or dedicated linters) to identify and remove CSS rules that are not used on your website. This is especially important when working with large projects or frameworks.
-
Inline Critical CSS: For above-the-fold content, consider inlining critical CSS directly within the
<style></style>
tag in your HTML. This allows the browser to render the initial content without waiting for the full CSS file to download. The rest of the CSS can then be loaded asynchronously. - Sprite Images: Combine multiple small images into a single sprite sheet and use CSS to position individual images within the sprite. This reduces the number of HTTP requests needed to load images referenced in your CSS.
How can I use CSS efficiently to avoid render-blocking and improve the overall performance of my HTML5 website?
Render-blocking refers to the situation where the browser must download and parse the CSS file before it can render the page content. This significantly slows down the perceived loading speed. Here's how to avoid it:
-
Asynchronous Loading: Load CSS files asynchronously using the
async
attribute in the<link>
tag. This allows the browser to download the CSS concurrently with rendering the page content. Note that this approach might lead to a brief flash of unstyled content (FOUT). -
Defer Loading: Alternatively, use the
defer
attribute. This ensures the CSS is downloaded and parsed after the HTML is parsed but before theDOMContentLoaded
event is fired. This generally avoids FOUT but might slightly delay the styling. -
Use
<link rel="preload">
: For critical CSS, use the<link rel="preload">
tag to signal to the browser that the CSS is important and should be loaded with high priority. This allows the browser to prioritize its download and reduce the time to first paint. - Optimize CSS Loading Order: Load your CSS files in a logical order, prioritizing critical CSS and then loading other stylesheets asynchronously.
What are some common CSS performance pitfalls to avoid when developing HTML5 applications?
Several common CSS practices can negatively impact performance. Avoid these pitfalls:
-
Overuse of
!important
: While useful in specific situations, overusing!important
makes it harder to maintain your CSS and can lead to unexpected conflicts and rendering issues. - Excessive Use of Nested Selectors: Deeply nested selectors can slow down the browser's rendering engine as it has to traverse the DOM tree more extensively. Keep your selectors concise and specific.
- Using Too Many IDs: IDs are more specific than classes, so overusing them can increase CSS specificity and potentially lead to performance issues. Classes are generally preferred for styling.
- Unoptimized Images: Using large, unoptimized images as CSS backgrounds significantly impacts page load speed. Optimize images and consider using CSS gradients or smaller images where possible.
- Poorly Organized CSS: Unorganized and poorly structured CSS can make it difficult to maintain and debug, increasing the risk of performance problems. Use a consistent naming convention, leverage CSS preprocessors for better organization, and regularly review and refactor your code.
- Ignoring Browser Caching: Failing to implement proper browser caching mechanisms leads to repeated downloads of the same CSS files, slowing down subsequent page loads. Ensure appropriate HTTP headers are set to utilize browser caching effectively.
The above is the detailed content of How to Optimize CSS for Performance in HTML5?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
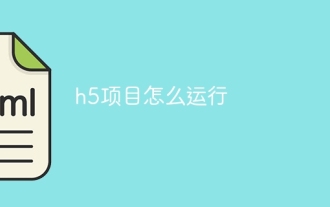
Running the H5 project requires the following steps: installing necessary tools such as web server, Node.js, development tools, etc. Build a development environment, create project folders, initialize projects, and write code. Start the development server and run the command using the command line. Preview the project in your browser and enter the development server URL. Publish projects, optimize code, deploy projects, and set up web server configuration.
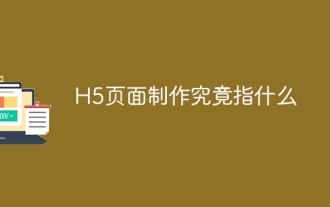
H5 page production refers to the creation of cross-platform compatible web pages using technologies such as HTML5, CSS3 and JavaScript. Its core lies in the browser's parsing code, rendering structure, style and interactive functions. Common technologies include animation effects, responsive design, and data interaction. To avoid errors, developers should be debugged; performance optimization and best practices include image format optimization, request reduction and code specifications, etc. to improve loading speed and code quality.
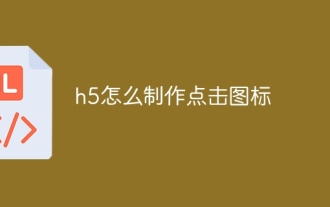
The steps to create an H5 click icon include: preparing a square source image in the image editing software. Add interactivity in the H5 editor and set the click event. Create a hotspot that covers the entire icon. Set the action of click events, such as jumping to the page or triggering animation. Export H5 documents as HTML, CSS, and JavaScript files. Deploy the exported files to a website or other platform.

H5referstoHTML5,apivotaltechnologyinwebdevelopment.1)HTML5introducesnewelementsandAPIsforrich,dynamicwebapplications.2)Itsupportsmultimediawithoutplugins,enhancinguserexperienceacrossdevices.3)SemanticelementsimprovecontentstructureandSEO.4)H5'srespo

H5 is not a standalone programming language, but a collection of HTML5, CSS3 and JavaScript for building modern web applications. 1. HTML5 defines the web page structure and content, and provides new tags and APIs. 2. CSS3 controls style and layout, and introduces new features such as animation. 3. JavaScript implements dynamic interaction and enhances functions through DOM operations and asynchronous requests.
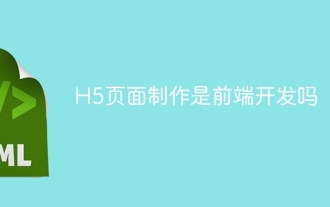
Yes, H5 page production is an important implementation method for front-end development, involving core technologies such as HTML, CSS and JavaScript. Developers build dynamic and powerful H5 pages by cleverly combining these technologies, such as using the <canvas> tag to draw graphics or using JavaScript to control interaction behavior.
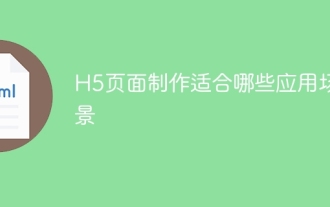
H5 (HTML5) is suitable for lightweight applications, such as marketing campaign pages, product display pages and corporate promotion micro-websites. Its advantages lie in cross-platformity and rich interactivity, but its limitations lie in complex interactions and animations, local resource access and offline capabilities.

H5 pop-up window creation steps: 1. Determine the triggering method (click, time, exit, scroll); 2. Design content (title, text, action button); 3. Set style (size, color, font, background); 4. Implement code (HTML, CSS, JavaScript); 5. Test and deployment.
