What are Python Tuples and When Should I Use Them?
This article explains Python tuples: ordered, immutable sequences. It details their key differences from lists (mutability, hashability), optimal use cases (data integrity, fixed collections), and unpacking techniques. Tuples are advocated for situ
What are Python Tuples and When Should I Use Them?
Python tuples are ordered, immutable sequences of items. This means they can contain elements of different data types (integers, strings, floats, even other tuples), and the order of those elements matters. However, unlike lists, tuples cannot be changed after they are created. You cannot add, remove, or modify elements within a tuple once it's been defined. This immutability is a key characteristic that distinguishes them from lists and determines when they are the appropriate choice.
You should use tuples when:
- Data integrity is paramount: If you need to ensure that a collection of data remains unchanged throughout your program's execution, a tuple provides this guarantee. This is particularly useful when dealing with sensitive data or configuration settings where accidental modification could have serious consequences.
- Representing fixed collections: When you have a collection of items whose number and values are known and shouldn't change, a tuple is a natural fit. Examples include coordinates (x, y), RGB color values (R, G, B), or database records.
- Improving code readability: Because tuples are immutable, the code that uses them can be easier to understand and reason about. The compiler can make certain optimizations knowing that a tuple's contents won't change unexpectedly.
- Using tuples as dictionary keys: Unlike lists, tuples are hashable, meaning they can be used as keys in dictionaries. This is because their immutability allows for consistent hashing.
What are the key differences between Python tuples and lists?
The primary difference between Python tuples and lists lies in their mutability:
Feature | Tuple | List |
---|---|---|
Mutability | Immutable (cannot be changed after creation) | Mutable (can be changed after creation) |
Syntax | Defined using parentheses ()
|
Defined using square brackets []
|
Use Cases | Representing fixed collections, data integrity | Storing and manipulating collections of data |
Hashability | Hashable (can be used as dictionary keys) | Not hashable (cannot be used as dictionary keys) |
Methods | Fewer built-in methods | More built-in methods |
Performance | Slightly faster than lists (due to immutability) | Generally slower than tuples |
In essence, choose tuples when you need a fixed collection of data that won't change, and lists when you need a dynamic collection that can be modified.
How do I unpack a tuple in Python?
Unpacking a tuple involves assigning the elements of the tuple to individual variables. This is a concise and efficient way to work with tuples, especially when they contain multiple values that you need to access separately. There are several ways to unpack tuples:
Simple Unpacking:
my_tuple = (10, 20, 30) a, b, c = my_tuple # a will be 10, b will be 20, c will be 30 print(a, b, c)
Unpacking with the asterisk operator (*
): This allows you to unpack a portion of the tuple into a list while assigning the remaining elements to individual variables. This is particularly useful when dealing with tuples of varying lengths.
my_tuple = (10, 20, 30, 40, 50) a, b, *rest = my_tuple # a=10, b=20, rest=[30, 40, 50] print(a, b, rest) a, *middle, c = my_tuple # a=10, middle=[20,30,40], c=50 print(a, middle, c)
Nested Tuple Unpacking:
nested_tuple = ((1, 2), (3, 4)) (x, y), (z, w) = nested_tuple print(x, y, z, w)
What are some common use cases for tuples in Python programming?
Tuples find applications in various areas of Python programming:
- Returning multiple values from a function: A function can return a tuple containing multiple values, making it easier to handle multiple results.
- Representing data records: Tuples are ideal for representing records in a database or other structured data, ensuring data integrity.
- Working with dictionaries: As mentioned earlier, tuples can be used as keys in dictionaries due to their immutability and hashability.
- Data serialization and deserialization: Tuples can be easily serialized (converted into a format suitable for storage or transmission) and deserialized (converted back into a Python object).
- Image processing: Representing pixel coordinates or color values.
- GUI programming: Defining coordinates or other fixed parameters.
- Machine learning: Representing features or data points.
In summary, while lists are versatile for mutable data, tuples offer a valuable alternative when immutability, hashability, and enhanced code readability are priorities. Their efficient implementation and specific use cases make them a fundamental part of Python's data structures.
The above is the detailed content of What are Python Tuples and When Should I Use Them?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
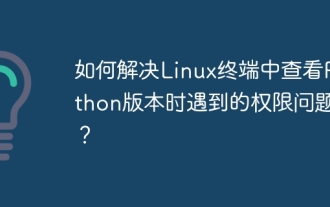
Solution to permission issues when viewing Python version in Linux terminal When you try to view Python version in Linux terminal, enter python...
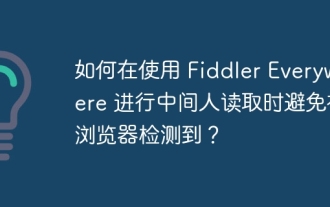
How to avoid being detected when using FiddlerEverywhere for man-in-the-middle readings When you use FiddlerEverywhere...
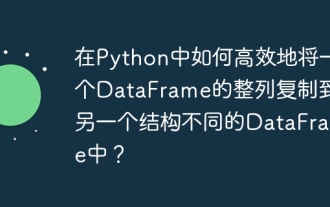
When using Python's pandas library, how to copy whole columns between two DataFrames with different structures is a common problem. Suppose we have two Dats...
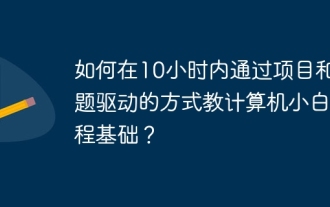
How to teach computer novice programming basics within 10 hours? If you only have 10 hours to teach computer novice some programming knowledge, what would you choose to teach...
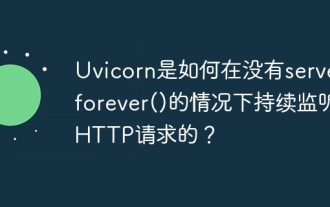
How does Uvicorn continuously listen for HTTP requests? Uvicorn is a lightweight web server based on ASGI. One of its core functions is to listen for HTTP requests and proceed...
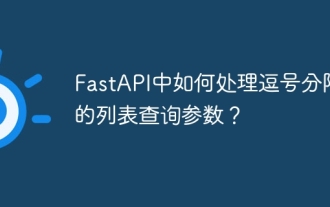
Fastapi ...
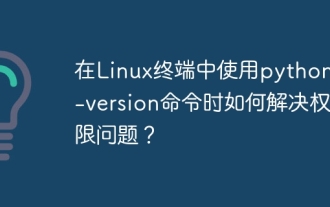
Using python in Linux terminal...
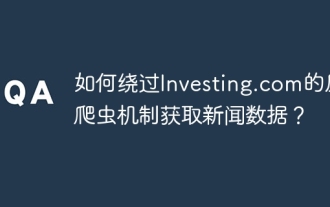
Understanding the anti-crawling strategy of Investing.com Many people often try to crawl news data from Investing.com (https://cn.investing.com/news/latest-news)...
