How do I implement custom network protocols in Go?
This article details implementing custom network protocols in Go using the net package. It covers defining message formats (e.g., using Protocol Buffers or JSON), handling connections, data transmission, and error management. Best practices for des
Implementing Custom Network Protocols in Go
This section details how to implement custom network protocols in Go. The core process involves leveraging Go's networking capabilities, specifically the net
package, to handle low-level socket operations. You'll need to define your protocol's message format (often using a serialization method like Protocol Buffers or JSON), handle connection establishment and termination, manage data transmission and reception, and implement error handling.
A basic example involves creating a TCP server and client. The server listens for incoming connections, receives data, processes it according to your protocol's specifications, and sends a response. The client initiates a connection, sends data formatted according to the protocol, and receives and processes the server's response. Here's a simplified illustration:
// Server package main import ( "fmt" "net" ) func handleConnection(conn net.Conn) { defer conn.Close() buffer := make([]byte, 1024) for { n, err := conn.Read(buffer) if err != nil { break } // Process received data according to your protocol fmt.Printf("Received: %s\n", buffer[:n]) // Send response conn.Write([]byte("Server response")) } } func main() { listener, err := net.Listen("tcp", ":8080") if err != nil { panic(err) } defer listener.Close() fmt.Println("Server listening on :8080") for { conn, err := listener.Accept() if err != nil { fmt.Println("Error accepting connection:", err) continue } go handleConnection(conn) } } //Client package main import ( "fmt" "net" ) func main() { conn, err := net.Dial("tcp", "localhost:8080") if err != nil { panic(err) } defer conn.Close() message := []byte("Client message") _, err = conn.Write(message) if err != nil { panic(err) } buffer := make([]byte, 1024) n, err := conn.Read(buffer) if err != nil { panic(err) } fmt.Printf("Received from server: %s\n", buffer[:n]) }
This is a rudimentary example. A real-world implementation would require more sophisticated error handling, data serialization, and potentially more complex state management. Remember to choose a suitable serialization format (like Protocol Buffers for efficiency and structured data or JSON for human readability) and handle potential network issues like dropped packets and connection failures robustly.
Best Practices for Designing Custom Network Protocols in Go
Designing a robust and maintainable custom network protocol requires careful consideration of several factors. Here are some best practices:
- Clearly Defined Message Format: Use a well-defined and documented message format. This ensures consistent interpretation of data between client and server. Protocol Buffers or similar serialization methods are highly recommended.
- Versioning: Implement versioning to allow for future protocol updates without breaking compatibility with older clients. Include a version number in each message.
- Error Handling: Handle network errors gracefully. Implement robust error detection and recovery mechanisms. Use appropriate error codes and messages.
- Security: Consider security implications early in the design process. Implement appropriate encryption and authentication mechanisms if necessary.
- Modularity: Design the protocol in a modular way to improve maintainability and allow for easier extension.
- Testability: Write unit and integration tests to verify the protocol's functionality and ensure correctness.
- Documentation: Provide clear and comprehensive documentation for the protocol, including message formats, error codes, and usage examples.
Existing Go Libraries for Custom Network Protocol Implementation
Several Go libraries can simplify custom network protocol implementation:
-
encoding/gob
: This package provides a simple mechanism for encoding and decoding Go data structures. It's suitable for internal protocols where compatibility with other systems isn't crucial. It's not ideal for interoperability with other languages. -
encoding/json
: Handles JSON encoding and decoding. JSON is human-readable and widely supported, making it suitable for protocols requiring interoperability with various systems. However, it can be less efficient than binary serialization methods. -
Protocol Buffers (protobuf): A language-neutral, platform-neutral extensible mechanism for serializing structured data. It's highly efficient and widely used for network protocols. The
google.golang.org/protobuf
package provides Go support.
Common Challenges and Pitfalls to Avoid
Implementing custom network protocols presents several challenges:
- Endianness: Ensure consistent handling of byte order (endianness) across different systems.
- Network Latency and Packet Loss: Account for network latency and potential packet loss. Implement mechanisms for reliable data transmission (e.g., using TCP or adding checksums).
- Debugging: Debugging network protocols can be challenging. Use logging and monitoring tools effectively.
- Security Vulnerabilities: Carelessly designed protocols can be vulnerable to various attacks. Thoroughly review the security implications of your design.
- Scalability: Consider the scalability of your protocol as the number of clients increases.
By following best practices and carefully addressing these challenges, you can successfully implement robust and efficient custom network protocols in Go. Remember that thorough testing and documentation are crucial for long-term maintainability and success.
The above is the detailed content of How do I implement custom network protocols in Go?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics




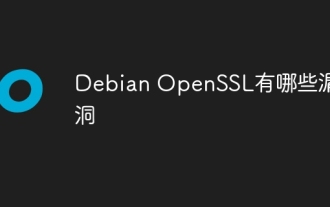
OpenSSL, as an open source library widely used in secure communications, provides encryption algorithms, keys and certificate management functions. However, there are some known security vulnerabilities in its historical version, some of which are extremely harmful. This article will focus on common vulnerabilities and response measures for OpenSSL in Debian systems. DebianOpenSSL known vulnerabilities: OpenSSL has experienced several serious vulnerabilities, such as: Heart Bleeding Vulnerability (CVE-2014-0160): This vulnerability affects OpenSSL 1.0.1 to 1.0.1f and 1.0.2 to 1.0.2 beta versions. An attacker can use this vulnerability to unauthorized read sensitive information on the server, including encryption keys, etc.
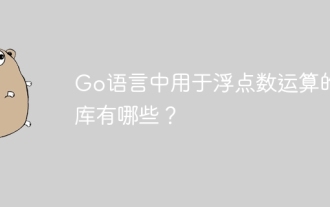
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
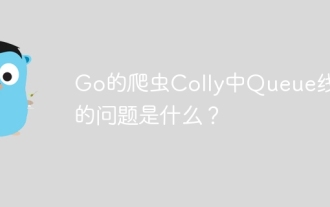
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
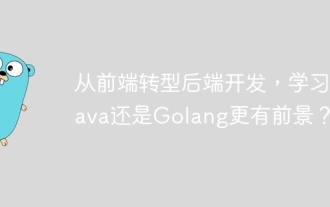
Backend learning path: The exploration journey from front-end to back-end As a back-end beginner who transforms from front-end development, you already have the foundation of nodejs,...
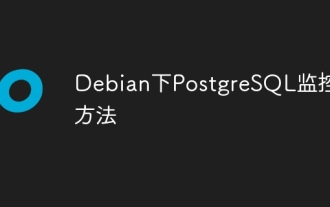
This article introduces a variety of methods and tools to monitor PostgreSQL databases under the Debian system, helping you to fully grasp database performance monitoring. 1. Use PostgreSQL to build-in monitoring view PostgreSQL itself provides multiple views for monitoring database activities: pg_stat_activity: displays database activities in real time, including connections, queries, transactions and other information. pg_stat_replication: Monitors replication status, especially suitable for stream replication clusters. pg_stat_database: Provides database statistics, such as database size, transaction commit/rollback times and other key indicators. 2. Use log analysis tool pgBadg
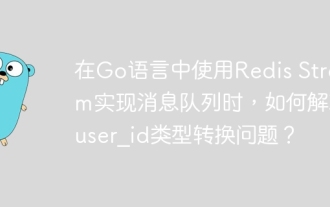
The problem of using RedisStream to implement message queues in Go language is using Go language and Redis...
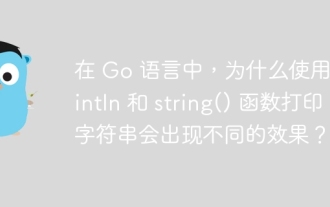
The difference between string printing in Go language: The difference in the effect of using Println and string() functions is in Go...
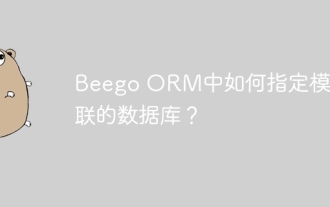
Under the BeegoORM framework, how to specify the database associated with the model? Many Beego projects require multiple databases to be operated simultaneously. When using Beego...
