How does Yii's autoloader work and how can I customize it?
How does Yii's autoloader work and how can I customize it?
Yii's autoloader, based on PSR-4, is a crucial component responsible for automatically loading classes as needed without requiring explicit require
or include
statements. It works by mapping namespaces to directory paths. When your code references a class, Yii's autoloader attempts to find a corresponding file based on the namespace and class name. For example, if your code uses \app\models\User
, the autoloader searches for a file located at app/models/User.php
. This mapping is typically defined in the application's configuration, often within the components
section under autoload
.
Yii's default autoloader configuration usually includes a classmap
array (for explicitly mapping class names to file paths) and a psr4
array (for PSR-4 autoloading). The psr4
array is the more commonly used method. It maps namespaces to directories. You can customize it by adding or modifying entries in your application configuration. For instance, to add a new namespace mapping, you might add the following to your application's configuration file (e.g., config/main.php
):
'components' => [ 'autoload' => [ 'psr4' => [ 'app\\' => [ '@app', // Alias to your application's base directory ], 'vendor\\mylibrary\\' => [ '@vendor/mylibrary', // Path to your third-party library ], ], ], ],
This example adds a mapping for the vendor\mylibrary
namespace to the @vendor/mylibrary
directory. You can adjust these paths to reflect your project structure. Modifying the classmap
array works similarly; you explicitly map class names to their file paths. Remember to clear the application's runtime cache after making changes to the autoloader configuration for the changes to take effect.
Can I improve Yii's autoloading performance for larger projects?
Yes, you can improve Yii's autoloading performance in larger projects by employing several strategies:
- Optimize Namespace Structure: A well-organized namespace structure reduces the search space for the autoloader. Avoid deeply nested namespaces if possible. Group related classes logically to minimize the number of directories the autoloader needs to traverse.
- Classmap for Frequently Used Classes: For classes that are heavily used throughout your application, adding them to the
classmap
array can significantly boost performance. Theclassmap
provides a direct mapping, bypassing the directory traversal inherent in PSR-4. - Caching: Yii's autoloader uses caching mechanisms internally. Ensure that your caching system is properly configured and functioning efficiently. A slow or improperly configured cache can negate the performance benefits of the autoloader.
- Opcode Caching: Implement an opcode caching mechanism like APC, OPcache, or Xcache. Opcode caching stores compiled PHP code in memory, reducing the overhead of repeated file parsing and compilation, thereby improving overall application performance, including autoloading.
- Avoid Unnecessary Autoloading: Minimize the number of classes loaded unnecessarily. Use dependency injection or lazy loading techniques to load classes only when they're actually required.
What are the common pitfalls to avoid when customizing Yii's autoloader?
Several pitfalls can arise when customizing Yii's autoloader:
- Incorrect Namespace Mappings: Double-check that your namespace mappings in the
psr4
array correctly map namespaces to the actual directory locations of your classes. Typos or incorrect paths are common sources of autoloading errors. - Circular Dependencies: Avoid creating circular dependencies between classes. If class A depends on class B, and class B depends on class A, the autoloader might enter an infinite loop, resulting in errors.
- Conflicting Namespace Mappings: Ensure that you don't have conflicting namespace mappings in your
psr4
array. If multiple entries map to the same namespace, unpredictable behavior can occur. - Ignoring Cache: Remember to clear the application's cache after making any changes to the autoloader configuration. Failing to do so might prevent the changes from taking effect.
- Overuse of
classmap
: Whileclassmap
offers performance advantages for frequently used classes, overusing it can lead to a large configuration file and potentially negate the benefits of autoloading. Use it judiciously.
How can I integrate a third-party library with Yii's autoloading mechanism?
Integrating a third-party library with Yii's autoloading mechanism usually involves adding a namespace mapping to your application's configuration. Assume your third-party library is located in the vendor
directory (a standard location for Composer-managed packages). If the library uses PSR-4 autoloading (as most modern libraries do), you'll need to add a mapping for its namespace to the psr4
array in your config/main.php
file. For example:
'components' => [ 'autoload' => [ 'psr4' => [ // ... existing mappings ... 'MyVendor\\MyLibrary\\' => ['@vendor/mylibrary'], // Replace with actual vendor and library path ], ], ],
This assumes the library's namespace is MyVendor\MyLibrary
and its source code is located in @vendor/mylibrary
. If the library uses a different autoloading mechanism (e.g., PSR-0 or a custom autoloader), you might need to consult its documentation for specific instructions on integration. In some cases, you may need to manually include the library's autoloader file before Yii's autoloader begins. Remember to replace placeholders like MyVendor
, MyLibrary
, and @vendor/mylibrary
with your actual library's details. If your library isn't using Composer, you may need to manually add the library's path to your PHP include path.
The above is the detailed content of How does Yii's autoloader work and how can I customize it?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
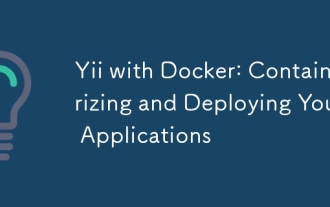
The steps to containerize and deploy Yii applications using Docker include: 1. Create a Dockerfile and define the image building process; 2. Use DockerCompose to launch Yii applications and MySQL database; 3. Optimize image size and performance. This involves not only specific technical operations, but also understanding the working principles and best practices of Dockerfile to ensure efficient and reliable deployment.
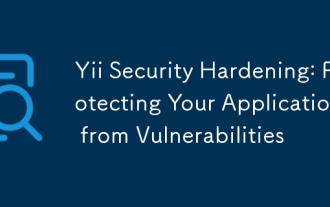
In the Yii framework, the application can be protected by the following steps: 1) Enable CSRF protection, 2) Implement input verification, and 3) Use output escape. These measures protect against CSRF, SQL injection and XSS attacks by embedding CSRF tokens, defining verification rules and automatic HTML escapes, ensuring the security of the application.

YiiremainspopularbutislessfavoredthanLaravel,withabout14kGitHubstars.ItexcelsinperformanceandActiveRecord,buthasasteeperlearningcurveandasmallerecosystem.It'sidealfordevelopersprioritizingefficiencyoveravastecosystem.
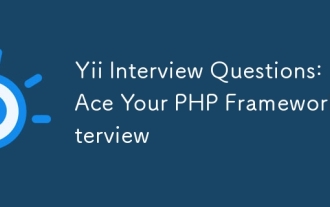
When preparing for an interview with Yii framework, you need to know the following key knowledge points: 1. MVC architecture: Understand the collaborative work of models, views and controllers. 2. ActiveRecord: Master the use of ORM tools and simplify database operations. 3. Widgets and Helpers: Familiar with built-in components and helper functions, and quickly build the user interface. Mastering these core concepts and best practices will help you stand out in the interview.
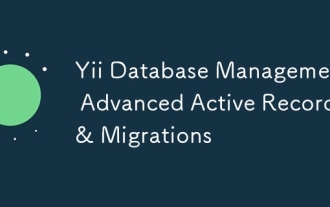
Advanced ActiveRecord and migration tools in the Yii framework are the key to efficiently managing databases. 1) Advanced ActiveRecord supports complex queries and data operations, such as associated queries and batch updates. 2) The migration tool is used to manage database structure changes and ensure secure updates to the schema.

Yii is a high-performance PHP framework designed for fast development and efficient code generation. Its core features include: MVC architecture: Yii adopts MVC architecture to help developers separate application logic and make the code easier to maintain and expand. Componentization and code generation: Through componentization and code generation, Yii reduces the repetitive work of developers and improves development efficiency. Performance Optimization: Yii uses latency loading and caching technologies to ensure efficient operation under high loads and provides powerful ORM capabilities to simplify database operations.
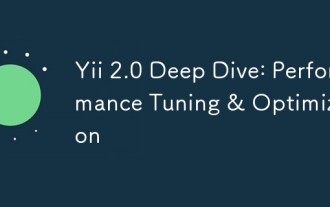
Strategies to improve Yii2.0 application performance include: 1. Database query optimization, using QueryBuilder and ActiveRecord to select specific fields and limit result sets; 2. Caching strategy, rational use of data, query and page cache; 3. Code-level optimization, reducing object creation and using efficient algorithms. Through these methods, the performance of Yii2.0 applications can be significantly improved.
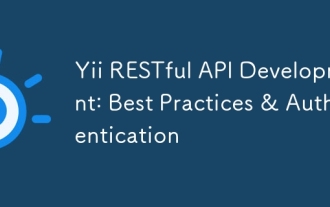
Developing a RESTful API in the Yii framework can be achieved through the following steps: Defining a controller: Use yii\rest\ActiveController to define a resource controller, such as UserController. Configure authentication: Ensure the security of the API by adding HTTPBearer authentication mechanism. Implement paging and sorting: Use yii\data\ActiveDataProvider to handle complex business logic. Error handling: Configure yii\web\ErrorHandler to customize error responses, such as handling when authentication fails. Performance optimization: Use Yii's caching mechanism to optimize frequently accessed resources and improve API performance.
