


What Are the Best Practices for Optimizing Laravel Performance in High-Traffic Applications?
This article details best practices for optimizing Laravel applications under high traffic. Key strategies include efficient querying, code optimization, robust caching (opcode, application-level, database, HTTP), server configuration, asynchronous
What Are the Best Practices for Optimizing Laravel Performance in High-Traffic Applications?
Optimizing Laravel for High-Traffic Applications: Best Practices
Optimizing Laravel for high-traffic applications requires a multi-faceted approach focusing on code efficiency, database optimization, and efficient resource utilization. Here's a breakdown of key best practices:
- Efficient Querying: Avoid N 1 queries. Use eager loading extensively to retrieve related data in a single database query. Employ proper indexing on your database tables to speed up data retrieval. Utilize query builders effectively and analyze slow queries using tools like Laravel Debugbar or database profiling features. Consider using techniques like pagination to reduce the amount of data retrieved at once.
- Code Optimization: Profile your application to identify performance bottlenecks. Optimize your code for efficiency – avoid unnecessary loops, function calls, and complex calculations. Leverage Laravel's built-in features like caching and queuing to offload heavy processing tasks. Use efficient data structures and algorithms where applicable. Regularly review and refactor your code to remove redundancy and improve clarity.
- Caching: Implement robust caching strategies using various levels of caching: opcode caching (e.g., Opcache), application-level caching (e.g., Redis, Memcached), and database query caching. Cache frequently accessed data to reduce database load and improve response times. Choose the right caching mechanism based on your application's needs and data characteristics.
- Server Configuration: Ensure your web server (e.g., Nginx, Apache) is properly configured for optimal performance. This includes adjusting settings like worker processes, connection limits, and buffer sizes. Use a load balancer to distribute traffic across multiple servers. Employ techniques like reverse proxies to enhance security and performance.
- Asynchronous Tasks: Utilize queues (e.g., Laravel Queues with Redis or RabbitMQ) to handle time-consuming tasks asynchronously. This prevents blocking the main application thread and improves responsiveness. This is especially crucial for operations like sending emails, processing images, or performing complex calculations.
- Monitoring and Logging: Implement comprehensive monitoring and logging to track application performance, identify potential issues, and proactively address bottlenecks. Use tools like Prometheus, Grafana, or Laravel Telescope to monitor key metrics and gain insights into your application's behavior under load.
How can I effectively scale my Laravel application to handle a sudden surge in user traffic?
Scaling a Laravel Application for Traffic Surges
Handling sudden traffic surges requires a proactive approach involving both vertical and horizontal scaling strategies:
- Vertical Scaling: Upgrade your server resources (CPU, RAM, storage) to handle increased load. This is a simpler solution for smaller applications but has limitations. It's often a temporary solution before implementing horizontal scaling.
- Horizontal Scaling: Distribute the load across multiple servers. This involves using a load balancer (e.g., Nginx, HAProxy) to distribute incoming requests across several application servers. Each server runs a copy of your application, sharing the workload. This provides better scalability and fault tolerance.
- Database Scaling: The database is often a bottleneck. Consider using database replication (read replicas) to distribute read operations across multiple database servers. For extremely high loads, explore database sharding to partition your data across multiple databases.
- Caching Strategies: Aggressive caching is crucial during traffic surges. Ensure your caching mechanisms are well-configured and can handle the increased requests. Consider using distributed caching solutions like Redis or Memcached, which can be accessed from multiple servers.
- Load Testing: Regularly perform load testing to simulate high-traffic scenarios and identify potential bottlenecks before they occur in production. This allows you to proactively adjust your infrastructure and optimize your application for peak performance.
- Autoscaling: Implement autoscaling capabilities using cloud platforms (e.g., AWS, Google Cloud, Azure) to automatically adjust server resources based on real-time demand. This ensures your application can handle traffic fluctuations dynamically without manual intervention.
What database optimization strategies are most crucial for maintaining Laravel application performance under heavy load?
Database Optimization for High-Load Laravel Applications
Database optimization is critical for maintaining Laravel application performance under heavy load. Key strategies include:
- Database Indexing: Properly index your database tables to speed up data retrieval. Analyze query execution plans to identify missing or inefficient indexes. Avoid over-indexing, as it can slow down write operations.
-
Query Optimization: Analyze slow queries and optimize them. Use EXPLAIN to understand how queries are executed. Avoid using
SELECT *
, instead select only the necessary columns. Use efficient joins and avoid using functions withinWHERE
clauses whenever possible. - Database Connection Pooling: Use connection pooling to reuse database connections, reducing the overhead of establishing new connections for each request.
- Read Replicas: Implement read replicas to offload read operations from the primary database server. This significantly reduces the load on the primary server, improving write performance.
- Database Tuning: Configure your database server (e.g., MySQL, PostgreSQL) for optimal performance. Adjust settings like buffer pool size, query cache size, and connection limits based on your application's needs and server resources.
- Data Normalization: Properly normalize your database schema to minimize data redundancy and improve data integrity. This can lead to more efficient queries and reduce storage space.
- Database Monitoring: Monitor your database performance using tools like MySQL Workbench or pgAdmin to identify bottlenecks and potential issues. Track metrics like query execution time, connection usage, and disk I/O.
What caching mechanisms are most effective for improving the response time of a high-traffic Laravel application?
Effective Caching Mechanisms for High-Traffic Laravel Applications
Leveraging different caching layers is essential for optimizing response times in high-traffic Laravel applications. Here's a breakdown of effective mechanisms:
- Opcode Caching (Opcache): This caches compiled PHP code, reducing the time it takes to execute scripts. It's usually enabled at the server level and is a fundamental optimization.
- Application-Level Caching (Redis, Memcached): These are in-memory data stores that are incredibly fast for storing and retrieving frequently accessed data. Use them to cache results of expensive database queries, API responses, or frequently rendered views. Redis offers more features and flexibility than Memcached.
- Database Query Caching: Laravel provides tools for caching database query results. This can significantly improve performance for queries that are repeatedly executed with the same parameters. However, be mindful of cache invalidation strategies to ensure data consistency.
- HTTP Caching: Leverage browser caching and CDN caching to store static assets (images, CSS, JavaScript) closer to users. This reduces the load on your application servers and improves page load times.
- Full Page Caching: Cache entire pages to reduce the server-side processing required for each request. This is particularly effective for pages that don't change frequently. However, carefully consider cache invalidation strategies to avoid stale data.
- Choosing the Right Mechanism: The optimal caching strategy depends on the specific needs of your application. Consider factors like data volatility, data size, and access patterns when choosing a caching mechanism. Often, a combination of different caching levels provides the best results.
The above is the detailed content of What Are the Best Practices for Optimizing Laravel Performance in High-Traffic Applications?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics

Both Django and Laravel are full-stack frameworks. Django is suitable for Python developers and complex business logic, while Laravel is suitable for PHP developers and elegant syntax. 1.Django is based on Python and follows the "battery-complete" philosophy, suitable for rapid development and high concurrency. 2.Laravel is based on PHP, emphasizing the developer experience, and is suitable for small to medium-sized projects.
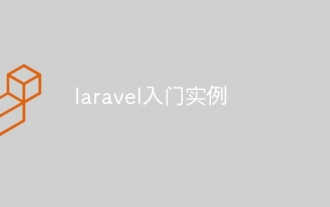
Laravel is a PHP framework for easy building of web applications. It provides a range of powerful features including: Installation: Install the Laravel CLI globally with Composer and create applications in the project directory. Routing: Define the relationship between the URL and the handler in routes/web.php. View: Create a view in resources/views to render the application's interface. Database Integration: Provides out-of-the-box integration with databases such as MySQL and uses migration to create and modify tables. Model and Controller: The model represents the database entity and the controller processes HTTP requests.

How does Laravel play a role in backend logic? It simplifies and enhances backend development through routing systems, EloquentORM, authentication and authorization, event and listeners, and performance optimization. 1. The routing system allows the definition of URL structure and request processing logic. 2.EloquentORM simplifies database interaction. 3. The authentication and authorization system is convenient for user management. 4. The event and listener implement loosely coupled code structure. 5. Performance optimization improves application efficiency through caching and queueing.
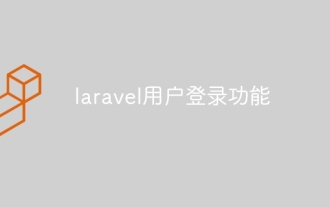
Laravel provides a comprehensive Auth framework for implementing user login functions, including: Defining user models (Eloquent model), creating login forms (Blade template engine), writing login controllers (inheriting Auth\LoginController), verifying login requests (Auth::attempt) Redirecting after login is successful (redirect) considering security factors: hash passwords, anti-CSRF protection, rate limiting and security headers. In addition, the Auth framework also provides functions such as resetting passwords, registering and verifying emails. For details, please refer to the Laravel documentation: https://laravel.com/doc

LaravelisabackendframeworkbuiltonPHP,designedforwebapplicationdevelopment.Itfocusesonserver-sidelogic,databasemanagement,andapplicationstructure,andcanbeintegratedwithfrontendtechnologieslikeVue.jsorReactforfull-stackdevelopment.

PHP and Laravel are not directly comparable, because Laravel is a PHP-based framework. 1.PHP is suitable for small projects or rapid prototyping because it is simple and direct. 2. Laravel is suitable for large projects or efficient development because it provides rich functions and tools, but has a steep learning curve and may not be as good as pure PHP.
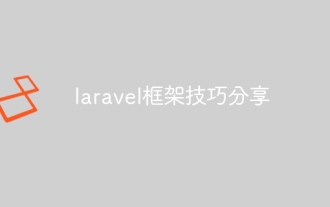
In this era of continuous technological advancement, mastering advanced frameworks is crucial for modern programmers. This article will help you improve your development skills by sharing little-known techniques in the Laravel framework. Known for its elegant syntax and a wide range of features, this article will dig into its powerful features and provide practical tips and tricks to help you create efficient and maintainable web applications.
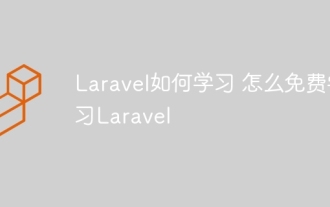
Want to learn the Laravel framework, but suffer from no resources or economic pressure? This article provides you with free learning of Laravel, teaching you how to use resources such as online platforms, documents and community forums to lay a solid foundation for your PHP development journey from getting started to master.
