


How do Java's concurrency utilities (Executors, Locks, Atomic Variables) work?
This article explains Java's concurrency utilities: Executors, Locks, and Atomic Variables. It details their functionalities, best practices for avoiding deadlocks and race conditions, and guidance on choosing the appropriate utility based on task c
How do Java's concurrency utilities (Executors, Locks, Atomic Variables) work?
Java's concurrency utilities provide powerful tools for managing concurrent access to shared resources and improving application performance. Let's break down how Executors, Locks, and Atomic Variables function:
Executors: Executors are high-level abstractions for managing threads. They simplify thread creation and management, allowing you to create and manage thread pools efficiently. The ExecutorService
interface is central; common implementations include ThreadPoolExecutor
(a flexible, customizable pool), ScheduledThreadPoolExecutor
(for scheduling tasks), and ForkJoinPool
(designed for divide-and-conquer algorithms). Executors
provides factory methods for easily creating these executors. They handle thread lifecycle (creation, termination, reuse) and often incorporate features like queuing submitted tasks, limiting the number of concurrently running threads, and handling exceptions.
Locks: Locks provide exclusive access to shared resources, preventing race conditions. The Lock
interface offers more flexibility than the synchronized
keyword. ReentrantLock
is a common implementation; it allows a thread to acquire the lock multiple times (reentrancy), preventing deadlocks in certain scenarios. ReadWriteLock
allows multiple readers but only one writer at a time, improving concurrency when read operations are far more frequent than writes. Lock
implementations offer methods like lock()
, tryLock()
, unlock()
, and tryLock(long time, TimeUnit unit)
for finer control over lock acquisition and release. Crucially, they require explicit unlocking; forgetting to unlock can lead to deadlocks.
Atomic Variables: Atomic variables provide atomic operations on variables, ensuring that operations on them are indivisible and thread-safe. Classes like AtomicInteger
, AtomicLong
, AtomicBoolean
, and AtomicReference
are provided. They use low-level atomic instructions to guarantee that reads and writes are atomic, eliminating the need for explicit synchronization mechanisms like locks for simple update operations. Methods like getAndIncrement()
, compareAndSet()
, and getAndSet()
perform atomic updates, returning the old value or indicating success/failure of a conditional update.
What are the best practices for using Java's concurrency utilities to avoid common pitfalls like deadlocks and race conditions?
Avoiding concurrency pitfalls requires careful design and coding practices:
- Minimize Shared Mutable State: Reduce the amount of shared data that multiple threads can modify. Immutability is a powerful tool; if data doesn't change, there's no need for synchronization.
- Use Appropriate Synchronization: Choose the right tool for the job. For simple atomic updates, use atomic variables. For more complex scenarios requiring exclusive access, use locks. For managing threads, use executors.
-
Avoid Deadlocks: Deadlocks occur when two or more threads are blocked indefinitely, waiting for each other to release resources. Careful ordering of lock acquisition, using timeouts in
tryLock()
, and avoiding circular dependencies are crucial. -
Handle Exceptions Properly: Ensure that locks are released even if exceptions occur. Use
finally
blocks to guaranteeunlock()
calls. -
Use Thread-Safe Collections: Instead of synchronizing access to regular collections (like
ArrayList
), use thread-safe alternatives likeConcurrentHashMap
,CopyOnWriteArrayList
, orConcurrentLinkedQueue
. - Proper Executor Configuration: Configure executors appropriately for your workload. Set appropriate thread pool sizes to avoid resource exhaustion or underutilization. Use bounded queues to prevent unbounded task accumulation.
- Testing and Monitoring: Thoroughly test your concurrent code with various scenarios and load conditions. Use monitoring tools to observe thread activity and resource usage.
How do I choose the appropriate concurrency utility (Executor, Lock, Atomic Variable) for a specific task in Java?
The choice depends on the nature of the task:
- Atomic Variables: Use for simple atomic updates to single variables. Suitable when you only need to perform indivisible operations like incrementing a counter or setting a flag.
-
Locks: Use when multiple threads need exclusive access to a shared resource.
ReentrantLock
is suitable for most scenarios;ReadWriteLock
is beneficial when reads significantly outnumber writes. -
Executors: Use for managing threads, especially when dealing with multiple tasks. Choose the appropriate executor type based on your needs (e.g.,
ThreadPoolExecutor
for general-purpose tasks,ScheduledThreadPoolExecutor
for scheduling).
When should I prefer using Java's concurrency utilities over simpler synchronization mechanisms like synchronized
blocks?
While synchronized
blocks are simple, Java's concurrency utilities often offer advantages:
-
Flexibility:
Lock
provides finer-grained control over locking thansynchronized
. You can usetryLock()
for non-blocking acquisition and implement more complex locking strategies. -
Performance: For certain tasks,
Lock
implementations (especiallyReentrantLock
) can offer performance advantages oversynchronized
, particularly in highly contended scenarios. Executors provide efficient thread management and pooling. -
Readability and Maintainability: Executors and well-structured lock usage can improve code clarity and reduce the risk of errors compared to intricate
synchronized
block usage. Atomic variables improve code readability by explicitly indicating atomic operations.
However, synchronized
remains useful for simple synchronization needs where its simplicity outweighs the benefits of more advanced utilities. For straightforward synchronization of small code blocks protecting shared resources, synchronized
can be perfectly adequate and easier to understand. The key is choosing the right tool for the job based on complexity and performance requirements.
The above is the detailed content of How do Java's concurrency utilities (Executors, Locks, Atomic Variables) work?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


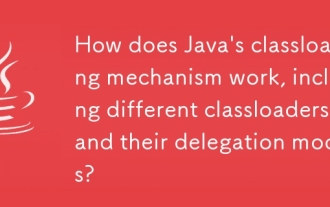
Java's classloading involves loading, linking, and initializing classes using a hierarchical system with Bootstrap, Extension, and Application classloaders. The parent delegation model ensures core classes are loaded first, affecting custom class loa
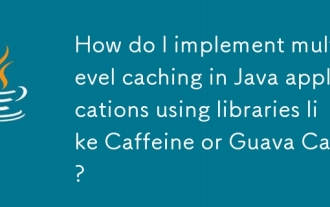
The article discusses implementing multi-level caching in Java using Caffeine and Guava Cache to enhance application performance. It covers setup, integration, and performance benefits, along with configuration and eviction policy management best pra
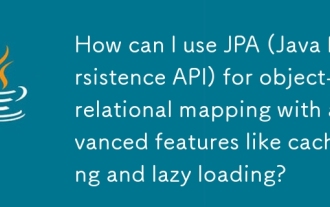
The article discusses using JPA for object-relational mapping with advanced features like caching and lazy loading. It covers setup, entity mapping, and best practices for optimizing performance while highlighting potential pitfalls.[159 characters]
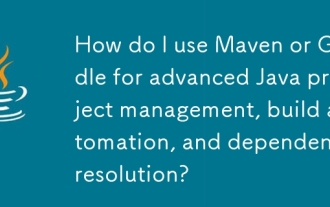
The article discusses using Maven and Gradle for Java project management, build automation, and dependency resolution, comparing their approaches and optimization strategies.
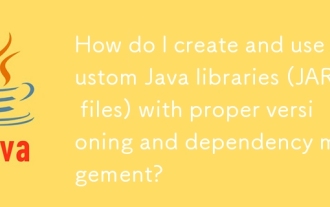
The article discusses creating and using custom Java libraries (JAR files) with proper versioning and dependency management, using tools like Maven and Gradle.
