


How can I prevent deadlocks, race conditions, and other concurrency issues in Java?
This article addresses concurrency issues in Java, focusing on preventing deadlocks and race conditions. It details strategies for designing concurrent applications, utilizing synchronization primitives (locks, semaphores), employing immutable objec
How can I prevent deadlocks, race conditions, and other concurrency issues in Java?
Preventing deadlocks, race conditions, and other concurrency issues in Java requires a multi-pronged approach focusing on careful design, code implementation, and testing. Here's a breakdown:
1. Design for Concurrency: Before writing any code, carefully consider how your application will handle concurrent access to shared resources. Identify potential points of contention and plan how to manage them. This includes:
- Minimizing Shared Resources: The fewer resources multiple threads need to access concurrently, the lower the risk of concurrency problems. Favor immutable objects whenever possible, as they inherently avoid race conditions. If mutable objects are necessary, carefully control access to them.
- Decomposition into Independent Tasks: Break down your application's functionality into smaller, independent tasks that can be executed concurrently with minimal interaction. This reduces the chances of threads interfering with each other.
- Strategic Resource Ordering: If multiple threads need to acquire multiple locks, always acquire them in the same order to prevent deadlocks. A deadlock occurs when two or more threads are blocked indefinitely, waiting for each other to release the resources that they need. Consistent lock ordering ensures that circular dependencies, a common cause of deadlocks, are avoided.
2. Proper Synchronization: Use appropriate synchronization mechanisms to control access to shared resources. This includes:
-
synchronized
blocks/methods: These provide mutual exclusion, ensuring that only one thread can access a critical section of code at a time. However, overuse can lead to performance bottlenecks. -
ReentrantLock
: Offers more flexibility thansynchronized
blocks, allowing for features like tryLock and interruptible locks. It's crucial to always release the lock, even in case of exceptions, using finally blocks. -
Semaphore
: Controls access to a limited number of resources. Useful for situations where you have a fixed pool of resources (e.g., database connections, threads in a thread pool). -
CountDownLatch
: Allows one or more threads to wait until a set of operations performed by other threads completes. -
CyclicBarrier
: Synchronizes a set of threads until they all reach a common barrier point.
3. Immutable Objects: Favor immutable objects whenever possible. Since their state cannot be changed after creation, they inherently eliminate race conditions.
4. Thread-Local Storage: Use ThreadLocal
to store data specific to each thread. This avoids the need for synchronization when accessing the data, as each thread has its own copy.
5. Careful Exception Handling: Ensure that locks are always released in finally
blocks to prevent deadlocks even if exceptions occur.
What are the best practices for thread safety in Java applications?
Thread safety is paramount in concurrent Java applications. Best practices include:
- Immutability: Make as many objects as possible immutable. This eliminates the need for synchronization because their state cannot be changed after creation.
-
Synchronization: Use appropriate synchronization primitives (
synchronized
,ReentrantLock
,Semaphore
, etc.) to control access to shared mutable state. Avoid unnecessary synchronization to minimize performance overhead. -
Atomic Operations: Utilize the
java.util.concurrent.atomic
package for atomic operations on primitive data types. These operations are guaranteed to be thread-safe without explicit synchronization. -
Thread Pools: Use
ExecutorService
to manage threads effectively. This prevents the overhead of creating and destroying threads constantly. It also allows for better control over resource usage. - Avoid Shared Mutable State: Minimize the use of shared mutable state. If it's unavoidable, carefully manage access using synchronization.
- Defensive Copying: When passing mutable objects to other threads, create copies to prevent unintended modifications.
- Testing: Thoroughly test your concurrent code with various concurrency patterns and load levels to identify potential issues. Use tools like JUnit and concurrency testing frameworks.
- Code Reviews: Have your code reviewed by others, especially those experienced in concurrent programming. A fresh pair of eyes can often spot potential problems that you might have missed.
How can I effectively use synchronization primitives like locks and semaphores to manage concurrent access in Java?
Synchronization primitives are essential for managing concurrent access to shared resources in Java. Here's how to effectively use locks and semaphores:
Locks (ReentrantLock
and synchronized
):
-
synchronized
blocks/methods: The simplest approach for mutual exclusion. Asynchronized
block or method ensures that only one thread can execute the code within it at any given time. However, it can be less flexible thanReentrantLock
. -
ReentrantLock
: Provides more advanced features thansynchronized
, such as tryLock (attempting to acquire the lock without blocking), lockInterruptibly (allowing a thread to be interrupted while waiting for the lock), and fair locks (prioritizing threads that have waited the longest). Crucially, always release the lock usingfinally
to prevent deadlocks. Example:
ReentrantLock lock = new ReentrantLock(); lock.lock(); try { // Access shared resource } finally { lock.unlock(); }
Semaphores:
Semaphores control access to a limited number of resources. They maintain a counter representing the number of available resources. A thread acquires a permit from the semaphore before accessing the resource and releases the permit when finished. Example:
Semaphore semaphore = new Semaphore(5); // 5 permits, representing 5 available resources try { semaphore.acquire(); // Acquire a permit // Access the resource } finally { semaphore.release(); // Release the permit }
What are some common tools and techniques for debugging concurrency problems in Java code?
Debugging concurrency issues can be challenging due to their non-deterministic nature. Here are some common tools and techniques:
- Logging: Strategic logging can help track the execution flow of different threads and identify potential race conditions or deadlocks. However, excessive logging can impact performance.
- Debuggers: Use a debugger (e.g., IntelliJ IDEA debugger, Eclipse debugger) to step through your code, observing the state of variables and threads. This can be particularly helpful for identifying race conditions. However, debugging concurrent code can be tricky due to the unpredictable nature of thread scheduling.
-
Thread Dumps: Generate thread dumps (using tools like
jstack
or IDE features) to get a snapshot of the state of all threads in your application. This can help identify blocked or waiting threads, which may indicate deadlocks. - Profilers: Profilers (e.g., JProfiler, YourKit) can help identify performance bottlenecks related to concurrency, such as excessive contention on shared resources.
- Concurrency Testing Frameworks: Frameworks like JUnit and dedicated concurrency testing libraries can help automate the process of testing your concurrent code under various load conditions and concurrency patterns. These frameworks help reveal subtle concurrency bugs that may not be easily reproducible manually.
- Instrumentation: Add instrumentation code to your application to track resource access patterns and thread execution timelines. This can help visualize the flow of execution and identify potential points of contention.
- Memory Analysis Tools: Tools like MAT (Memory Analyzer Tool) can help identify memory leaks related to concurrency, which can lead to performance issues and even application crashes.
Remember that a combination of careful design, proper synchronization, and thorough testing is key to building robust and reliable concurrent Java applications.
The above is the detailed content of How can I prevent deadlocks, race conditions, and other concurrency issues in Java?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
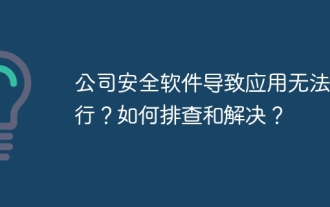
Troubleshooting and solutions to the company's security software that causes some applications to not function properly. Many companies will deploy security software in order to ensure internal network security. ...
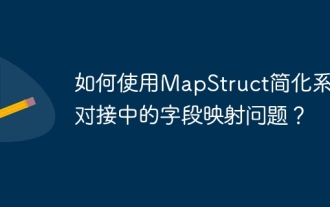
Field mapping processing in system docking often encounters a difficult problem when performing system docking: how to effectively map the interface fields of system A...
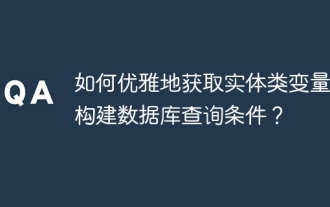
When using MyBatis-Plus or other ORM frameworks for database operations, it is often necessary to construct query conditions based on the attribute name of the entity class. If you manually every time...
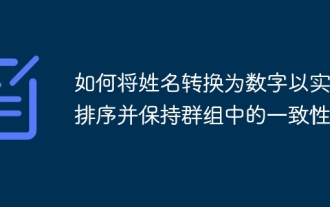
Solutions to convert names to numbers to implement sorting In many application scenarios, users may need to sort in groups, especially in one...
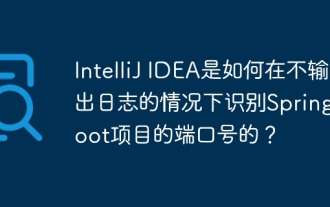
Start Spring using IntelliJIDEAUltimate version...
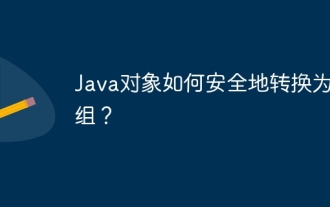
Conversion of Java Objects and Arrays: In-depth discussion of the risks and correct methods of cast type conversion Many Java beginners will encounter the conversion of an object into an array...
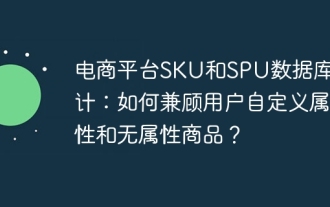
Detailed explanation of the design of SKU and SPU tables on e-commerce platforms This article will discuss the database design issues of SKU and SPU in e-commerce platforms, especially how to deal with user-defined sales...
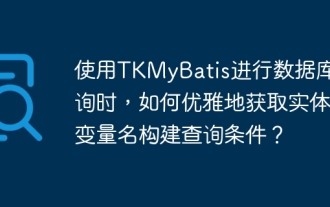
When using TKMyBatis for database queries, how to gracefully get entity class variable names to build query conditions is a common problem. This article will pin...
