How do I perform basic operations in Redis (SET, GET, DEL, INCR, DECR)?
This article explains basic Redis commands (SET, GET, DEL, INCR, DECR), optimizing their use via pipelining and efficient data structures. It also covers error handling, transaction management, and more efficient alternatives like MGET and MSET f
Performing Basic Operations in Redis (SET, GET, DEL, INCR, DECR)
Redis provides a straightforward API for basic operations. Let's explore SET
, GET
, DEL
, INCR
, and DECR
.
-
SET: This command sets the value of a key. The syntax is
SET key value
. For example,SET mykey "Hello, world!"
stores the string "Hello, world!" at the keymykey
. Redis overwrites the value if the key already exists. You can useSETNX
(SET if Not eXists) to only set the key if it doesn't already exist. -
GET: This command retrieves the value associated with a key. The syntax is
GET key
. For example,GET mykey
would return "Hello, world!". If the key doesn't exist, it returnsnil
. -
DEL: This command deletes a key. The syntax is
DEL key [key ...]
. You can delete multiple keys at once by providing them as arguments. For example,DEL mykey anotherkey
deletes both keys. If a key doesn't exist, it's silently ignored. -
INCR: This command increments the value of a key by 1. The key must hold an integer value. The syntax is
INCR key
. If the key doesn't exist, it's initialized to 0 before incrementing. -
DECR: This command decrements the value of a key by 1. The key must hold an integer value. The syntax is
DECR key
. If the key doesn't exist, it's initialized to 0 before decrementing.
Best Practices for Using Redis Basic Commands
Optimizing the use of SET
, GET
, DEL
, INCR
, and DECR
involves several strategies:
- Pipeline Commands: For multiple operations, use pipelining to reduce network round trips. Send multiple commands to the server at once, and receive all the responses together. This significantly improves performance.
- Use Appropriate Data Structures: While these commands work with strings, consider using other Redis data structures like lists, sets, or sorted sets for more complex scenarios. For instance, if you need to maintain an ordered list of items, a list is far more efficient than using multiple keys and managing ordering yourself.
- Key Naming Conventions: Use descriptive and consistent key naming conventions to improve code readability and maintainability. This helps in debugging and understanding the data stored in Redis.
-
Avoid Unnecessary Operations: Minimize the number of
GET
andSET
calls by carefully designing your application logic. If possible, batch operations to reduce the overhead of individual requests. - Efficient Data Serialization: If storing complex data structures, use efficient serialization methods like JSON or Protocol Buffers to minimize the size of data stored and improve performance.
Handling Errors When Using Redis Basic Commands
Error handling is crucial for robust applications. Redis commands typically return specific responses to indicate success or failure.
- Connection Errors: Handle potential connection errors (network issues, server down) gracefully. Implement retry mechanisms with exponential backoff to avoid overwhelming the server.
-
Key Not Found: Check for
nil
responses fromGET
to handle cases where the key doesn't exist. This avoids exceptions or unexpected behavior in your application. -
Type Mismatches: Ensure that keys hold the expected data types (e.g., integers for
INCR
andDECR
). Handle type mismatch errors appropriately, perhaps by logging an error or taking corrective action. -
Transaction Management: For operations that must be atomic, use Redis transactions (
MULTI
,EXEC
,DISCARD
). This ensures that either all operations succeed or none do. - Exception Handling: Use appropriate exception handling mechanisms (try-catch blocks) in your code to handle potential errors gracefully and prevent application crashes.
Alternative and More Efficient Commands
While SET
, GET
, DEL
, INCR
, and DECR
are fundamental, more efficient alternatives exist for specific use cases:
-
MGET
: Retrieves the values of multiple keys in a single command, improving efficiency compared to multiple individualGET
calls. -
MSET
: Sets the values of multiple keys simultaneously, more efficient than multipleSET
commands. -
INCRBY
andDECRBY
: Increment or decrement by an arbitrary value, not just 1. -
APPEND
: Appends a value to the end of an existing string value, avoiding a fullGET
andSET
. -
BITOP
: Perform bitwise operations on strings, useful for specific scenarios like setting flags or managing bitmaps.
Choosing the right command depends heavily on the specific use case. Analyzing your application's requirements and selecting the most appropriate commands can lead to substantial performance gains.
The above is the detailed content of How do I perform basic operations in Redis (SET, GET, DEL, INCR, DECR)?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics



The article discusses choosing shard keys in Redis Cluster, emphasizing their impact on performance, scalability, and data distribution. Key issues include ensuring even data distribution, aligning with access patterns, and avoiding common mistakes l
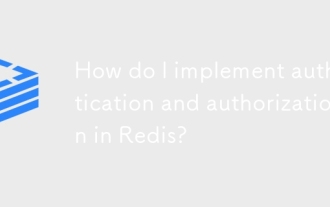
The article discusses implementing authentication and authorization in Redis, focusing on enabling authentication, using ACLs, and best practices for securing Redis. It also covers managing user permissions and tools to enhance Redis security.
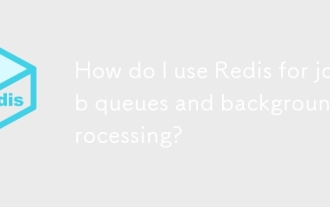
The article discusses using Redis for job queues and background processing, detailing setup, job definition, and execution. It covers best practices like atomic operations and job prioritization, and explains how Redis enhances processing efficiency.
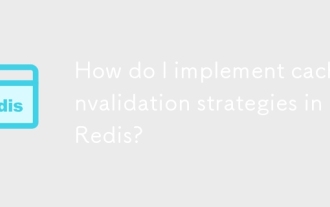
The article discusses strategies for implementing and managing cache invalidation in Redis, including time-based expiration, event-driven methods, and versioning. It also covers best practices for cache expiration and tools for monitoring and automat
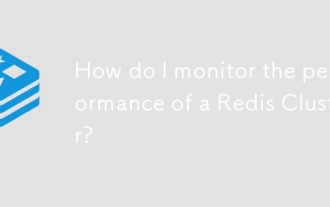
Article discusses monitoring Redis Cluster performance and health using tools like Redis CLI, Redis Insight, and third-party solutions like Datadog and Prometheus.
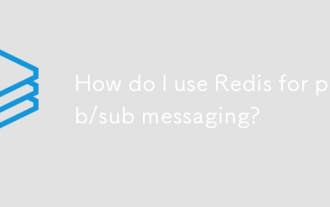
The article explains how to use Redis for pub/sub messaging, covering setup, best practices, ensuring message reliability, and monitoring performance.
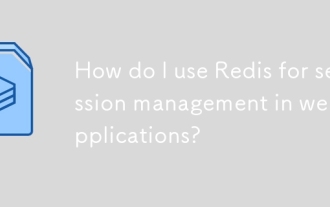
The article discusses using Redis for session management in web applications, detailing setup, benefits like scalability and performance, and security measures.
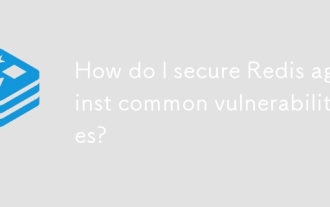
Article discusses securing Redis against vulnerabilities, focusing on strong passwords, network binding, command disabling, authentication, encryption, updates, and monitoring.
