How do I use Redis lists for queuing and pub/sub?
This article explores using Redis lists for queuing and pub/sub. While lists effectively implement FIFO/LIFO queues using LPUSH/RPOP, they are inefficient for pub/sub compared to Redis's native mechanism. The article also discusses performance tr
How to Use Redis Lists for Queuing and Pub/Sub?
Redis lists provide a straightforward way to implement both queuing and publish/subscribe (pub/sub) systems, although they are better suited for queuing. Let's break down each use case:
Queuing: Redis lists utilize the LPUSH
(left push) and RPOP
(right pop) commands for implementing a First-In, First-Out (FIFO) queue. LPUSH
adds elements to the head of the list, while RPOP
removes and returns the element at the tail. This creates a classic queue where items are processed in the order they are added. For a Last-In, First-Out (LIFO) stack, you would use RPUSH
(right push) and LPOP
(left pop).
Example (FIFO Queue):
Imagine a task queue. Workers consume tasks from a list named "tasks":
-
Producer: Uses
LPUSH tasks "task1"
to add tasks to the queue. -
Consumer: Uses
BRPOP tasks 0
(blocking pop) to wait for a task.BRPOP
blocks until a task is available or the timeout (0 means indefinite wait) is reached. Once a task is available, it's removed and processed.
Pub/Sub: While Redis lists can be adapted for pub/sub, it's not their primary strength. Redis's built-in pub/sub mechanism using PUBLISH
and SUBSCRIBE
commands is far more efficient and designed specifically for this purpose. Using lists for pub/sub would involve pushing messages to a list and having subscribers repeatedly polling the list for new messages, which is inefficient and scales poorly compared to the native pub/sub. Therefore, for pub/sub, use Redis's native pub/sub functionality.
What are the Performance Trade-offs Between Using Redis Lists and Other Data Structures for Queuing?
Redis offers several data structures suitable for queuing, each with performance trade-offs:
-
Lists: Excellent for simple FIFO or LIFO queues. Performance is good for moderate-sized queues, but
BRPOP
can become a bottleneck under heavy contention with many consumers waiting for tasks. Memory usage scales linearly with queue size. - Streams: Introduced in Redis 5.0, streams are purpose-built for message queuing. They offer features like message persistence, consumer groups, and efficient message delivery, significantly improving reliability and scalability compared to lists. Streams handle high throughput and concurrency better than lists. However, they have a slightly steeper learning curve.
- Sorted Sets: Useful for priority queues, where tasks have associated priorities. Sorted sets allow efficient retrieval of the highest-priority task. However, maintaining sorted order adds overhead compared to simple lists.
In summary: Lists are suitable for simple, low-concurrency queues. For high-throughput, reliable, and scalable queues, Redis Streams are the preferred choice. Sorted sets are ideal when task prioritization is crucial.
How Can I Implement a Reliable Message Queue with Redis Lists, Handling Potential Failures?
Implementing a truly reliable message queue with just Redis lists is challenging. Redis lists themselves don't offer features like message persistence beyond the server's memory. To improve reliability, consider these strategies:
- Persistence: Use Redis persistence mechanisms (RDB or AOF) to ensure data survives server restarts. However, this doesn't guarantee zero data loss during a very short failure window.
-
Transactions: Wrap
LPUSH
andRPOP
operations within transactions (MULTI
,EXEC
) to ensure atomicity. This prevents partial operations in case of failures. - Message Acknowledgements: Implement a mechanism where consumers acknowledge successful processing of a message. If a consumer fails before acknowledgement, the message remains in the queue. This requires a separate mechanism (e.g., a separate Redis key or an external database) to track acknowledgements.
- Dead-Letter Queues: Create a separate queue ("dead-letter-queue") to store messages that fail processing multiple times. This prevents messages from being lost and allows for later investigation.
- Monitoring: Monitor queue lengths and processing times to identify potential bottlenecks and failures.
These techniques enhance reliability but don't eliminate the possibility of data loss in extreme scenarios. For mission-critical applications, a more robust message queue system (e.g., Kafka, RabbitMQ) is recommended.
What Are Some Best Practices for Using Redis Lists for Pub/Sub Messaging, Ensuring Scalability and Efficiency?
As previously stated, Redis lists are not the ideal choice for pub/sub. However, if you must use them, follow these practices (keeping in mind that these are workarounds and less efficient than native pub/sub):
-
Avoid polling: Continuously polling the list using
LRANGE
with a small timeout is highly inefficient. It wastes resources and increases latency. -
Use
BLPOP
orBRPOP
: Blocking pops (BLPOP
for left pop,BRPOP
for right pop) are more efficient than polling. They only consume resources when a message is available. - Multiple lists: For multiple subscribers, consider using separate lists for each subscriber to avoid contention. This increases memory usage but improves performance under high concurrency.
- Consider message acknowledgment: Although this adds complexity, it prevents message loss if a subscriber crashes after receiving but before processing a message.
Crucially, remember that Redis's native pub/sub system is far superior for pub/sub scenarios. These "best practices" are merely mitigation strategies for using a tool not designed for the task. Use Redis lists for queuing, and use Redis's built-in pub/sub for publish/subscribe operations for optimal performance and scalability.
The above is the detailed content of How do I use Redis lists for queuing and pub/sub?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics



The article discusses choosing shard keys in Redis Cluster, emphasizing their impact on performance, scalability, and data distribution. Key issues include ensuring even data distribution, aligning with access patterns, and avoiding common mistakes l
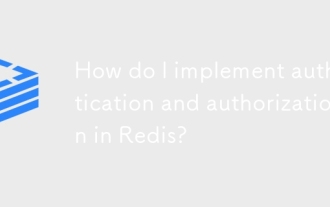
The article discusses implementing authentication and authorization in Redis, focusing on enabling authentication, using ACLs, and best practices for securing Redis. It also covers managing user permissions and tools to enhance Redis security.
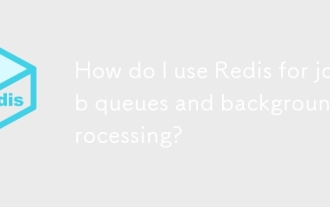
The article discusses using Redis for job queues and background processing, detailing setup, job definition, and execution. It covers best practices like atomic operations and job prioritization, and explains how Redis enhances processing efficiency.
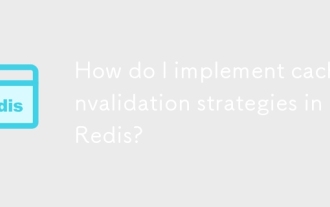
The article discusses strategies for implementing and managing cache invalidation in Redis, including time-based expiration, event-driven methods, and versioning. It also covers best practices for cache expiration and tools for monitoring and automat
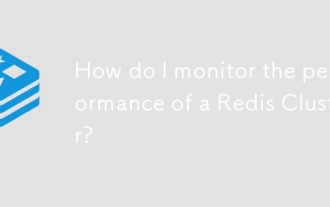
Article discusses monitoring Redis Cluster performance and health using tools like Redis CLI, Redis Insight, and third-party solutions like Datadog and Prometheus.
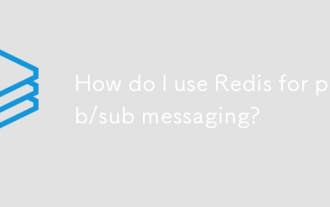
The article explains how to use Redis for pub/sub messaging, covering setup, best practices, ensuring message reliability, and monitoring performance.
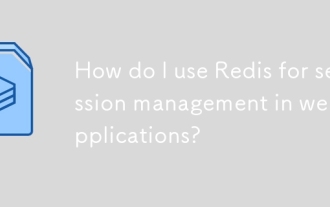
The article discusses using Redis for session management in web applications, detailing setup, benefits like scalability and performance, and security measures.
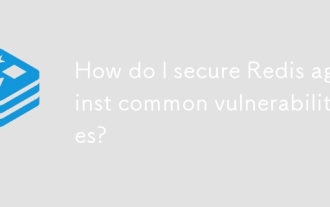
Article discusses securing Redis against vulnerabilities, focusing on strong passwords, network binding, command disabling, authentication, encryption, updates, and monitoring.
