How do I use recursive CTEs in SQL to query hierarchical data?
This article explains SQL's Recursive Common Table Expressions (CTEs) for querying hierarchical data. It details their structure, using an organizational chart example, and addresses common pitfalls like infinite recursion and incorrect joins. Opti
Using Recursive CTEs for Hierarchical Data
Recursive Common Table Expressions (CTEs) are a powerful tool in SQL for querying hierarchical data, such as organizational charts, file systems, or bill-of-materials. They allow you to traverse a tree-like structure by repeatedly referencing the CTE itself within its definition. The basic structure involves an anchor member (the initial query) and a recursive member (the self-referencing part).
Let's illustrate with a simple example of an organizational chart represented in a table named employees
:
CREATE TABLE employees ( employee_id INT PRIMARY KEY, employee_name VARCHAR(255), manager_id INT ); INSERT INTO employees (employee_id, employee_name, manager_id) VALUES (1, 'CEO', NULL), (2, 'VP Sales', 1), (3, 'Sales Rep 1', 2), (4, 'Sales Rep 2', 2), (5, 'VP Marketing', 1), (6, 'Marketing Manager', 5);
To retrieve the entire hierarchy under the CEO (employee_id 1), we use a recursive CTE:
WITH RECURSIVE EmployeeHierarchy AS ( -- Anchor member: Selects the CEO SELECT employee_id, employee_name, manager_id, 0 as level FROM employees WHERE employee_id = 1 UNION ALL -- Recursive member: Joins with itself to find subordinates SELECT e.employee_id, e.employee_name, e.manager_id, eh.level 1 FROM employees e INNER JOIN EmployeeHierarchy eh ON e.manager_id = eh.employee_id ) SELECT * FROM EmployeeHierarchy;
This query starts with the CEO and recursively adds subordinates until no more employees report to those already included. The level
column indicates the depth in the hierarchy. The UNION ALL
combines the results of the anchor and recursive members. The key is the self-join between employees
and EmployeeHierarchy
in the recursive member, linking each employee to their manager.
Common Pitfalls to Avoid When Using Recursive CTEs
Several pitfalls can lead to incorrect results or performance issues when working with recursive CTEs:
- Infinite Recursion: The most common mistake is creating a cycle in your data or a recursive query that doesn't have a proper termination condition. This will cause the query to run indefinitely. Ensure your data is acyclic (no employee reports to themselves, directly or indirectly) and that the recursive member eventually terminates (e.g., by reaching a leaf node in the hierarchy).
- Incorrect Join Conditions: Using incorrect join conditions in the recursive member will lead to missing or extra data. Carefully check your join condition to ensure it accurately reflects the hierarchical relationship in your data.
-
Lack of Termination Condition: A recursive CTE must have a clear termination condition to prevent infinite loops. This is usually done by checking for a specific value (e.g.,
NULL
in a parent ID column) or by limiting the recursion depth. -
Ignoring Data Duplicates: Using
UNION ALL
instead ofUNION
will include duplicate rows if they exist in the hierarchy. UseUNION
if you need to eliminate duplicates. However,UNION ALL
is generally faster.
Optimizing Recursive CTE Queries for Large Datasets
Recursive CTEs can be slow on very large hierarchical datasets. Several optimization strategies can improve performance:
- Indexing: Ensure appropriate indexes exist on the columns used in the join conditions (typically the parent-child relationship columns). Indexes significantly speed up the joins within the recursive CTE.
-
Filtering: Limit the scope of the recursion by adding
WHERE
clauses to the anchor and/or recursive members to filter out unnecessary branches of the hierarchy. This reduces the amount of data processed. - Materialized Views: For frequently executed recursive queries, consider creating a materialized view that pre-computes the hierarchical data. This can significantly improve query performance at the cost of storage space and some data staleness.
- Alternative Approaches: For exceptionally large datasets, consider alternative approaches like using adjacency lists or nested sets, which can offer better performance for certain hierarchical queries. Recursive CTEs are not always the optimal solution for all scenarios.
- Batch Processing: Instead of processing the entire hierarchy in a single query, consider breaking it down into smaller batches.
Recursive CTEs in Different Database Systems
Recursive CTEs are supported by most major database systems, but the syntax might vary slightly:
-
SQL Server: Uses
WITH RECURSIVE
(although theRECURSIVE
keyword is optional). -
PostgreSQL: Uses
WITH RECURSIVE
. - MySQL: Supports recursive CTEs starting from version 8.0. The syntax is similar to PostgreSQL.
-
Oracle: Supports recursive CTEs with the
START WITH
andCONNECT BY
clauses, which have a slightly different syntax but achieve the same functionality.
While the core concept remains the same across different systems, always consult the documentation of your specific database system for the correct syntax and any system-specific limitations or optimizations. Remember to test your queries thoroughly and profile their performance to identify and address bottlenecks.
The above is the detailed content of How do I use recursive CTEs in SQL to query hierarchical data?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


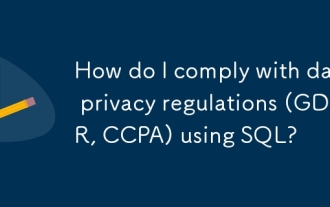
Article discusses using SQL for GDPR and CCPA compliance, focusing on data anonymization, access requests, and automatic deletion of outdated data.(159 characters)
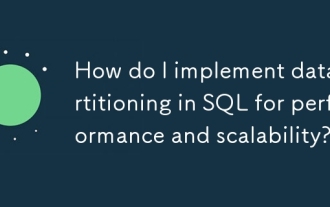
Article discusses implementing data partitioning in SQL for better performance and scalability, detailing methods, best practices, and monitoring tools.
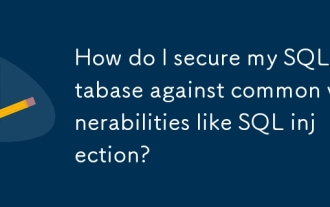
The article discusses securing SQL databases against vulnerabilities like SQL injection, emphasizing prepared statements, input validation, and regular updates.
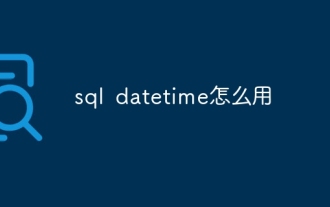
The DATETIME data type is used to store high-precision date and time information, ranging from 0001-01-01 00:00:00 to 9999-12-31 23:59:59.99999999, and the syntax is DATETIME(precision), where precision specifies the accuracy after the decimal point (0-7), and the default is 3. It supports sorting, calculation, and time zone conversion functions, but needs to be aware of potential issues when converting precision, range and time zones.
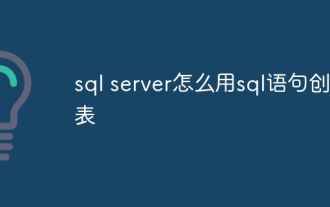
How to create tables using SQL statements in SQL Server: Open SQL Server Management Studio and connect to the database server. Select the database to create the table. Enter the CREATE TABLE statement to specify the table name, column name, data type, and constraints. Click the Execute button to create the table.
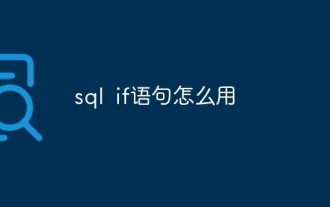
SQL IF statements are used to conditionally execute SQL statements, with the syntax as: IF (condition) THEN {statement} ELSE {statement} END IF;. The condition can be any valid SQL expression, and if the condition is true, execute the THEN clause; if the condition is false, execute the ELSE clause. IF statements can be nested, allowing for more complex conditional checks.
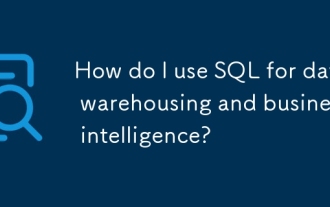
The article discusses using SQL for data warehousing and business intelligence, focusing on ETL processes, data modeling, and query optimization. It also covers BI report creation and tool integration.
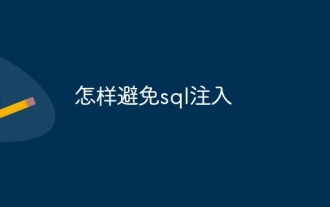
To avoid SQL injection attacks, you can take the following steps: Use parameterized queries to prevent malicious code injection. Escape special characters to avoid them breaking SQL query syntax. Verify user input against the whitelist for security. Implement input verification to check the format of user input. Use the security framework to simplify the implementation of protection measures. Keep software and databases updated to patch security vulnerabilities. Restrict database access to protect sensitive data. Encrypt sensitive data to prevent unauthorized access. Regularly scan and monitor to detect security vulnerabilities and abnormal activity.
