How do I test Vuex stores?
How to Test Vuex Stores
Testing your Vuex stores is crucial for ensuring the reliability and predictability of your Vue.js application. A well-tested store guarantees that your application's data layer behaves as expected, preventing unexpected behavior and simplifying debugging. There are several approaches to testing Vuex stores, primarily focusing on unit testing individual components of the store (actions, mutations, getters) and potentially integration tests covering the interactions between them. The most common approach involves using a testing framework like Jest alongside a mocking library such as jest-mock
.
You'll typically test your actions, mutations, and getters separately. For actions, you'll verify that they correctly dispatch mutations and handle asynchronous operations (using promises or async/await). For mutations, you'll assert that they correctly modify the application state. Getters are tested by verifying that they return the expected derived data based on the current state. Each test should be concise, focusing on a single aspect of the store's functionality. This allows for easy identification and resolution of issues if a test fails.
What are the Best Practices for Testing Vuex Actions and Mutations?
Best practices for testing Vuex actions and mutations revolve around clear, concise, and isolated tests.
For Actions:
- Focus on the outcome: Don't test the internal implementation details of an action, but rather the final result. Does the action correctly dispatch the expected mutations and handle potential errors?
- Mock asynchronous operations: When testing actions involving API calls or other asynchronous operations, use mocking to simulate the responses, ensuring predictable test results regardless of external factors.
- Test error handling: Actions should gracefully handle errors. Write tests that verify the error handling mechanisms are working correctly.
- Use clear assertions: Employ clear and specific assertions to verify the expected state changes or returned values.
- Keep tests independent: Each test should be independent and not rely on the state or outcome of other tests. Consider using a fresh store instance for each test.
For Mutations:
- Test state changes directly: Mutations should directly modify the state. Test each mutation by verifying that it correctly updates the state based on the provided payload.
- Keep mutations simple and focused: Mutations should perform a single, specific operation. This makes testing easier and more manageable.
- Avoid side effects: Mutations should ideally only modify the state and avoid any external interactions like API calls.
- Use snapshot testing cautiously: Snapshot testing can be helpful for verifying complex state changes, but rely on it cautiously, ensuring you understand and maintain the snapshots. Changes to snapshots should be carefully reviewed.
How Can I Effectively Use Mocking in My Vuex Store Tests?
Mocking is essential when testing Vuex stores, particularly when dealing with asynchronous operations or external dependencies. Mocking allows you to isolate the component under test, preventing unexpected behavior caused by external factors. This ensures consistent and reliable test results.
Mocking in Jest:
Jest's built-in mocking capabilities are ideal for this. You can mock API calls, database interactions, or any other external dependencies.
// Example mocking an API call within an action jest.mock('./api', () => ({ fetchData: jest.fn(() => Promise.resolve({ data: 'mocked data' })), })); // In your test: it('should fetch data successfully', async () => { const action = actions.fetchData; await action({ commit }, { someParam: 'value' }); expect(api.fetchData).toHaveBeenCalledWith({ someParam: 'value' }); expect(commit).toHaveBeenCalledWith('setData', { data: 'mocked data' }); });
This example mocks the fetchData
function from the ./api
module. The jest.fn()
creates a mock function that allows you to control its behavior and verify its calls. You can customize the mock's return value to simulate various scenarios. This keeps the test isolated from the actual API call and makes it faster and more reliable.
What Tools and Libraries Are Recommended for Unit Testing Vuex Stores?
Several tools and libraries are highly recommended for unit testing Vuex stores. The most common combination is:
- Jest: A powerful and widely used JavaScript testing framework. It provides excellent features for mocking, asynchronous testing, and snapshot testing.
- Vue Test Utils: A utility library from the Vue ecosystem specifically designed for testing Vue components. While primarily for component testing, it integrates well with testing Vuex stores.
-
jest-mock: Jest's built-in mocking capabilities are sufficient for most cases, eliminating the need for external mocking libraries. However, for more complex mocking scenarios, libraries like
sinon
can be helpful.
These tools work well together to provide a comprehensive testing environment for your Vuex stores. Jest handles the test runner and assertion capabilities, while Vue Test Utils offers helpful utilities for interacting with Vue components and their associated stores. This combination allows for thorough and efficient testing of all aspects of your Vuex implementation. The choice to include additional libraries like sinon
depends on your specific needs and complexity of mocking requirements.
The above is the detailed content of How do I test Vuex stores?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
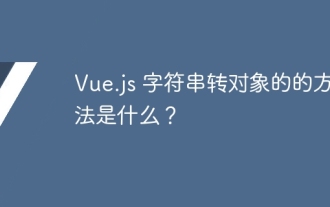
Using JSON.parse() string to object is the safest and most efficient: make sure that strings comply with JSON specifications and avoid common errors. Use try...catch to handle exceptions to improve code robustness. Avoid using the eval() method, which has security risks. For huge JSON strings, chunked parsing or asynchronous parsing can be considered for optimizing performance.

Vue.js is suitable for small and medium-sized projects and fast iterations, while React is suitable for large and complex applications. 1) Vue.js is easy to use and is suitable for situations where the team is insufficient or the project scale is small. 2) React has a richer ecosystem and is suitable for projects with high performance and complex functional needs.
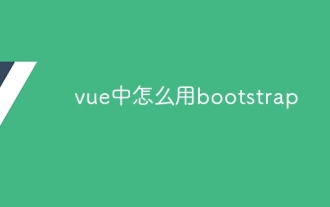
Using Bootstrap in Vue.js is divided into five steps: Install Bootstrap. Import Bootstrap in main.js. Use the Bootstrap component directly in the template. Optional: Custom style. Optional: Use plug-ins.

Vue.js is not difficult to learn, especially for developers with a JavaScript foundation. 1) Its progressive design and responsive system simplify the development process. 2) Component-based development makes code management more efficient. 3) The usage examples show basic and advanced usage. 4) Common errors can be debugged through VueDevtools. 5) Performance optimization and best practices, such as using v-if/v-show and key attributes, can improve application efficiency.
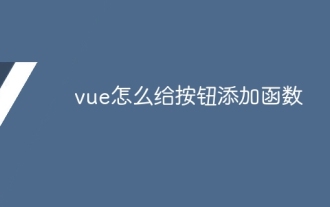
You can add a function to the Vue button by binding the button in the HTML template to a method. Define the method and write function logic in the Vue instance.

Vue.js is mainly used for front-end development. 1) It is a lightweight and flexible JavaScript framework focused on building user interfaces and single-page applications. 2) The core of Vue.js is its responsive data system, and the view is automatically updated when the data changes. 3) It supports component development, and the UI can be split into independent and reusable components.
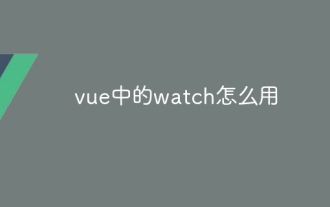
The watch option in Vue.js allows developers to listen for changes in specific data. When the data changes, watch triggers a callback function to perform update views or other tasks. Its configuration options include immediate, which specifies whether to execute a callback immediately, and deep, which specifies whether to recursively listen to changes to objects or arrays.
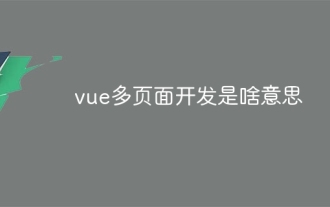
Vue multi-page development is a way to build applications using the Vue.js framework, where the application is divided into separate pages: Code Maintenance: Splitting the application into multiple pages can make the code easier to manage and maintain. Modularity: Each page can be used as a separate module for easy reuse and replacement. Simple routing: Navigation between pages can be managed through simple routing configuration. SEO Optimization: Each page has its own URL, which helps SEO.
