How do I validate Bootstrap forms using JavaScript?
How to Validate Bootstrap Forms Using JavaScript
Validating Bootstrap forms with JavaScript involves leveraging JavaScript's capabilities to check user input before submission. This ensures data integrity and a better user experience. You can achieve this through various methods, primarily using event listeners and regular expressions. Here's a breakdown:
1. Event Listeners: Attach an event listener (typically onsubmit
for the form or oninput
for individual fields) to trigger the validation function. This function will perform the checks.
2. Validation Logic: Within your validation function, you'll use JavaScript to check the values of form fields. This might involve:
-
Required Fields: Checking if fields marked as required actually contain data. You can access field values using
document.getElementById("fieldName").value
. -
Data Types: Ensuring fields are of the correct type (e.g., numbers, emails, dates). Regular expressions are incredibly useful here. For example,
/^[^\s@] @[^\s@] \.[^\s@] $/
checks for a valid email format. -
Length Restrictions: Verifying that fields meet minimum or maximum length requirements.
value.length
provides the length of the string. - Custom Validation: Implementing any project-specific validation rules (e.g., password complexity).
3. Providing Feedback: After validation, provide clear feedback to the user. This can be done by:
-
Displaying error messages: Use Bootstrap's alert classes (e.g.,
alert-danger
) to display error messages near the respective fields. You can dynamically add or remove these messages based on validation results. You might useinnerHTML
to update the content of a designated error message element. -
Styling invalid fields: Add Bootstrap classes (e.g.,
is-invalid
) to visually highlight invalid fields. Bootstrap automatically styles these classes. -
Preventing submission: If validation fails, prevent the form from submitting using
event.preventDefault()
.
Example (Illustrative):
document.getElementById("myForm").addEventListener("submit", function(event) { event.preventDefault(); // Prevent default submission let isValid = true; //Check required fields if (document.getElementById("name").value === "") { document.getElementById("nameError").innerHTML = "Name is required"; document.getElementById("name").classList.add("is-invalid"); isValid = false; } else { document.getElementById("nameError").innerHTML = ""; document.getElementById("name").classList.remove("is-invalid"); } //Check email format if (!/^[^\s@] @[^\s@] \.[^\s@] $/.test(document.getElementById("email").value)) { document.getElementById("emailError").innerHTML = "Invalid email format"; document.getElementById("email").classList.add("is-invalid"); isValid = false; } else { document.getElementById("emailError").innerHTML = ""; document.getElementById("email").classList.remove("is-invalid"); } if (isValid) { //Submit the form if valid this.submit(); } });
This example demonstrates basic validation; more complex scenarios might require more elaborate logic.
Can I Use JavaScript Validation with Bootstrap's Form Styling?
Absolutely! Bootstrap's form styling works seamlessly with JavaScript validation. Bootstrap provides CSS classes (is-valid
, is-invalid
, was-validated
) specifically designed to visually indicate the validity of form fields. Your JavaScript validation logic can dynamically add or remove these classes based on the validation results. This ensures that the visual feedback provided by Bootstrap aligns perfectly with your JavaScript validation. The example above already showcases this integration.
What Are the Best Practices for Validating Bootstrap Forms with JavaScript?
Several best practices enhance the effectiveness and user experience of JavaScript form validation within a Bootstrap context:
- Client-side and Server-side Validation: While client-side validation (using JavaScript) provides immediate feedback, always perform server-side validation as well. Client-side validation can be bypassed, so server-side validation is crucial for security and data integrity.
- Clear and Concise Error Messages: Error messages should be clear, specific, and easy to understand. Avoid technical jargon. Position error messages close to the respective fields.
- Progressive Enhancement: Ensure your forms work correctly even if JavaScript is disabled. Provide fallback validation mechanisms (e.g., server-side validation alone).
- Accessibility: Make your validation accessible to users with disabilities. Use ARIA attributes to convey validation status to assistive technologies (e.g., screen readers).
- Maintainability: Keep your validation code organized, well-commented, and easy to maintain. Consider using a validation library (like a form validation plugin) for complex scenarios.
- User Experience: Provide helpful suggestions and guidance to users as they fill out the form. For instance, use placeholder text to indicate expected input formats.
How Can I Integrate JavaScript Form Validation into My Existing Bootstrap Project?
Integrating JavaScript validation into your existing Bootstrap project is straightforward:
-
Include JavaScript: Ensure you have a
<script></script>
tag in your HTML file (preferably at the end of theor in a separate
.js
file) to include your JavaScript validation code. -
Identify Form Elements: Use JavaScript's
document.getElementById()
orquerySelector()
methods to access your Bootstrap form elements (fields, buttons, etc.). -
Add Event Listeners: Attach event listeners (
onsubmit
,oninput
, etc.) to the form or individual fields to trigger your validation function when appropriate. - Implement Validation Logic: Write your validation logic, using JavaScript's built-in functions, regular expressions, or external libraries.
-
Provide Feedback: Use Bootstrap's CSS classes (
is-valid
,is-invalid
) to visually indicate the validity of fields. Display clear and concise error messages near the respective fields. -
Prevent Submission (if needed): Use
event.preventDefault()
to prevent form submission if validation fails. - Test Thoroughly: Test your validation thoroughly in different browsers and scenarios to ensure it functions correctly.
Remember to place your JavaScript code within <script></script>
tags in your HTML file or link to an external JavaScript file. Ensure your CSS and JavaScript files are correctly linked and loaded before the form is rendered. This integration is essentially the same process described in the first answer, but within the context of an already established Bootstrap project.
The above is the detailed content of How do I validate Bootstrap forms using JavaScript?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
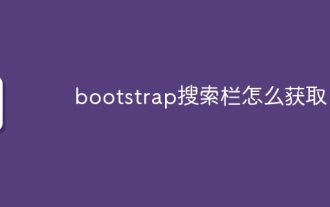
How to use Bootstrap to get the value of the search bar: Determines the ID or name of the search bar. Use JavaScript to get DOM elements. Gets the value of the element. Perform the required actions.
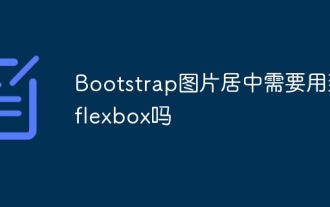
There are many ways to center Bootstrap pictures, and you don’t have to use Flexbox. If you only need to center horizontally, the text-center class is enough; if you need to center vertically or multiple elements, Flexbox or Grid is more suitable. Flexbox is less compatible and may increase complexity, while Grid is more powerful and has a higher learning cost. When choosing a method, you should weigh the pros and cons and choose the most suitable method according to your needs and preferences.
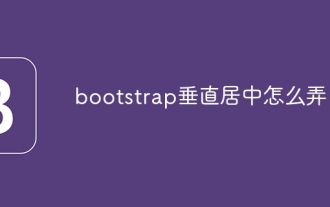
Use Bootstrap to implement vertical centering: flexbox method: Use the d-flex, justify-content-center, and align-items-center classes to place elements in the flexbox container. align-items-center class method: For browsers that do not support flexbox, use the align-items-center class, provided that the parent element has a defined height.
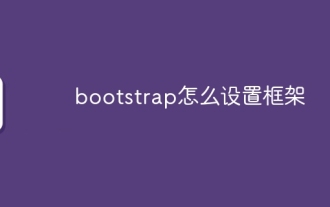
To set up the Bootstrap framework, you need to follow these steps: 1. Reference the Bootstrap file via CDN; 2. Download and host the file on your own server; 3. Include the Bootstrap file in HTML; 4. Compile Sass/Less as needed; 5. Import a custom file (optional). Once setup is complete, you can use Bootstrap's grid systems, components, and styles to create responsive websites and applications.
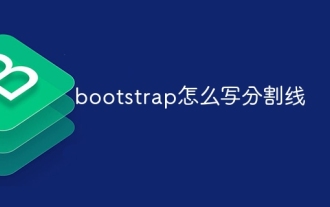
There are two ways to create a Bootstrap split line: using the tag, which creates a horizontal split line. Use the CSS border property to create custom style split lines.

Building an inclusive and user-friendly website with Bootstrap can be achieved through the following steps: 1. Enhance screen reader support with ARIA tags; 2. Adjust color contrast to comply with WCAG standards; 3. Ensure keyboard navigation is friendly. These measures ensure that the website is friendly and accessible to all users, including those with barriers.
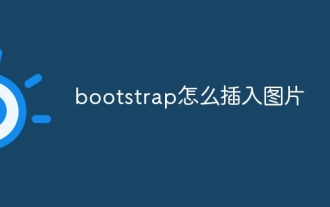
There are several ways to insert images in Bootstrap: insert images directly, using the HTML img tag. With the Bootstrap image component, you can provide responsive images and more styles. Set the image size, use the img-fluid class to make the image adaptable. Set the border, using the img-bordered class. Set the rounded corners and use the img-rounded class. Set the shadow, use the shadow class. Resize and position the image, using CSS style. Using the background image, use the background-image CSS property.
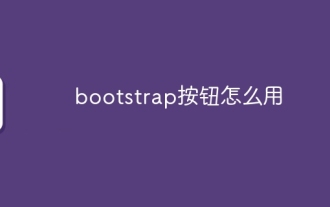
How to use the Bootstrap button? Introduce Bootstrap CSS to create button elements and add Bootstrap button class to add button text
