


How do I use the HTML5 <progress> element to display the completion progress of a task?
Using the HTML5 <progress></progress>
Element to Display Task Completion Progress
The HTML5 <progress></progress>
element is designed to visually represent the progress of a task. It's a simple yet effective way to give users feedback on ongoing processes. The core attribute is value
, which represents the current progress, and max
, which defines the total value representing 100% completion. The browser automatically calculates and displays the progress bar based on these two values. For example:
<progress value="25" max="100"></progress>
This code snippet creates a progress bar that is 25% complete. The value
attribute is set to 25, indicating the current progress, while max
is set to 100, representing the total value. The browser will render a progress bar visually reflecting this 25% completion. You can also add labels for clarity:
<progress value="25" max="100">25% complete</progress>
The text "25% complete" will be displayed if the browser doesn't support visual progress bars, or as an accessibility aid. Remember that the value
attribute should always be less than or equal to the max
attribute. Attempting to set value
higher than max
will result in the progress bar showing 100% completion.
Customizing the Appearance of the HTML5 <progress>
Element
Unfortunately, the styling options for the <progress>
element are very limited with standard CSS. You cannot directly style the progress bar's appearance using properties like background-color
, border-radius
, or height
. Browsers render the progress bar with their own default styles. This is a significant limitation.
To overcome this, you can employ a workaround using JavaScript and a custom element or a library. This involves creating a visual representation of a progress bar using elements like div
and span
, then manipulating their styles with JavaScript to mimic the functionality of the <progress>
element. This approach gives you full control over the appearance, but it requires more code and effort.
Dynamically Updating the HTML5 <progress>
Element with JavaScript
Updating the <progress>
element dynamically with JavaScript is straightforward. You simply modify the value
attribute using the setAttribute()
method or directly accessing the value
property. For example:
const progressBar = document.querySelector('progress'); let currentValue = 0; function updateProgress(newValue) { currentValue = newValue; if (currentValue > 100) { currentValue = 100; } progressBar.value = currentValue; } // Example usage: update progress every second setInterval(() => { updateProgress(10); // Increment progress by 10 }, 1000);
This code snippet selects the <progress></progress>
element, then uses setInterval
to increment the value
attribute every second. The updateProgress
function ensures that the value
never exceeds the max
value (implicitly 100 unless otherwise specified). You can adapt this code to update the progress based on any event or calculation relevant to your application. Remember to include error handling to prevent unexpected behavior.
Browser Compatibility Issues with the HTML5 <progress></progress>
Element
The <progress></progress>
element enjoys relatively good browser support. Most modern browsers (Chrome, Firefox, Safari, Edge) render it correctly. However, older browsers might not support it or render it inconsistently. Furthermore, the lack of CSS styling options means that the visual appearance can vary significantly across different browsers, even among modern ones, due to default browser styling.
For optimal compatibility, you should always include a fallback mechanism. This could involve displaying an alternative text representation of the progress or using a JavaScript-based solution that provides a consistent cross-browser experience. Testing your application across different browsers and versions is crucial to ensure consistent behavior and appearance. Consider using a polyfill or a custom progress bar implementation if you need to support older browsers that lack native <progress></progress>
support.
The above is the detailed content of How do I use the HTML5 <progress> element to display the completion progress of a task?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


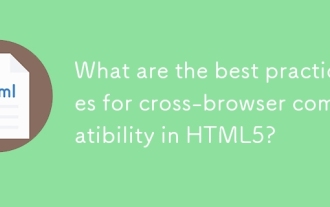
Article discusses best practices for ensuring HTML5 cross-browser compatibility, focusing on feature detection, progressive enhancement, and testing methods.
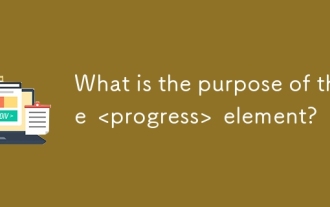
The article discusses the HTML <progress> element, its purpose, styling, and differences from the <meter> element. The main focus is on using <progress> for task completion and <meter> for stati
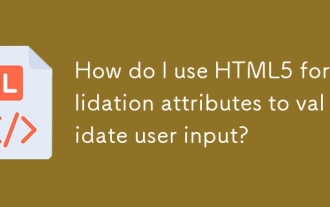
The article discusses using HTML5 form validation attributes like required, pattern, min, max, and length limits to validate user input directly in the browser.
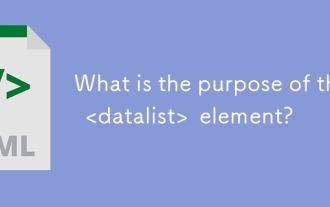
The article discusses the HTML <datalist> element, which enhances forms by providing autocomplete suggestions, improving user experience and reducing errors.Character count: 159
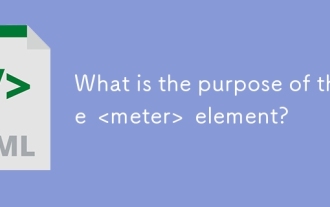
The article discusses the HTML <meter> element, used for displaying scalar or fractional values within a range, and its common applications in web development. It differentiates <meter> from <progress> and ex
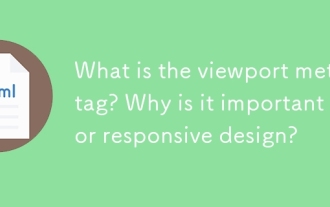
The article discusses the viewport meta tag, essential for responsive web design on mobile devices. It explains how proper use ensures optimal content scaling and user interaction, while misuse can lead to design and accessibility issues.
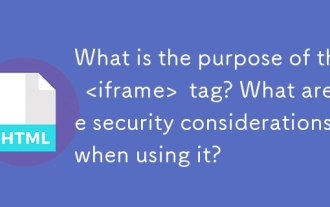
The article discusses the <iframe> tag's purpose in embedding external content into webpages, its common uses, security risks, and alternatives like object tags and APIs.

HTML is suitable for beginners because it is simple and easy to learn and can quickly see results. 1) The learning curve of HTML is smooth and easy to get started. 2) Just master the basic tags to start creating web pages. 3) High flexibility and can be used in combination with CSS and JavaScript. 4) Rich learning resources and modern tools support the learning process.
