What are virtual functions in C and how do they enable polymorphism?
What are virtual functions in C and how do they enable polymorphism?
Understanding Virtual Functions and Polymorphism
In C , virtual functions are member functions declared within a class using the virtual
keyword. Their primary purpose is to enable polymorphism, a powerful object-oriented programming (OOP) concept that allows you to treat objects of different classes in a uniform way. This is achieved through runtime dispatch.
When a virtual function is called on an object, the actual function to be executed isn't determined at compile time (static binding). Instead, it's determined at runtime (dynamic binding) based on the object's dynamic type (the type of the object at runtime). This means that if you have a base class pointer pointing to a derived class object, and the pointer calls a virtual function, the derived class's version of that function will be executed.
Let's illustrate with an example:
class Animal { public: virtual void makeSound() { // Virtual function std::cout << "Generic animal sound" << std::endl; } }; class Dog : public Animal { public: void makeSound() override { // Overriding the virtual function std::cout << "Woof!" << std::endl; } }; class Cat : public Animal { public: void makeSound() override { // Overriding the virtual function std::cout << "Meow!" << std::endl; } }; int main() { Animal* animal = new Dog(); animal->makeSound(); // Output: Woof! (Runtime polymorphism) animal = new Cat(); animal->makeSound(); // Output: Meow! (Runtime polymorphism) delete animal; return 0; }
In this example, makeSound
is a virtual function. Even though animal
is declared as an Animal
pointer, the correct makeSound
function (either Dog
's or Cat
's) is called at runtime depending on the actual object type. This is the essence of polymorphism enabled by virtual functions. Without the virtual
keyword, the Animal
's version of makeSound
would always be called, regardless of the actual object type (static dispatch).
Why are virtual functions important for object-oriented programming in C ?
The Importance of Virtual Functions in OOP
Virtual functions are crucial for achieving several key OOP principles:
- Polymorphism: As discussed above, they are the foundation of runtime polymorphism, allowing you to write flexible and extensible code that can handle objects of different classes uniformly. This avoids the need for extensive conditional logic based on object types.
- Extensibility: You can easily add new derived classes without modifying the existing base class code. The virtual function mechanism automatically handles calls to the appropriate overridden function in the derived class.
- Code Reusability: Virtual functions promote code reusability by allowing derived classes to inherit and extend the functionality of the base class without needing to rewrite the entire function. They allow for specialization of behavior.
- Abstraction: Virtual functions contribute to abstraction by hiding implementation details. The client code interacts with the base class interface, unaware of the specific implementation details of the derived classes.
How do virtual functions differ from regular member functions in C ?
Virtual vs. Regular Member Functions
The key difference lies in how they are bound:
- Virtual Functions: Bound at runtime (dynamic dispatch). The appropriate function is determined based on the object's dynamic type at the time of the function call. They require a virtual function table (vtable) to achieve this runtime binding.
- Regular Member Functions: Bound at compile time (static dispatch). The compiler determines which function to call based on the static type of the object (the type declared in the code). No vtable is involved.
Another difference is the virtual
keyword. Virtual functions are declared using the virtual
keyword in the base class. Derived classes can override them using the override
keyword (C 11 and later). Regular member functions don't use the virtual
keyword. Overriding a non-virtual function in a derived class simply creates a new, separate function; it doesn't replace the base class function in the way that overriding a virtual function does.
What are the performance implications of using virtual functions in C ?
Performance Implications of Virtual Functions
While virtual functions provide significant advantages in terms of code flexibility and maintainability, they do introduce some performance overhead:
- Vtable Overhead: Each class with virtual functions has an associated vtable, which is a table of function pointers. This adds a small amount of memory overhead.
- Indirect Function Call: Calling a virtual function involves an indirect function call through the vtable. This indirect call is generally slower than a direct function call to a regular member function. The compiler cannot optimize away the indirect call as it doesn't know at compile time which function will be executed.
However, the performance impact is usually negligible in most applications. The overhead of a single virtual function call is small, and the benefits of polymorphism and code maintainability often outweigh the minor performance cost. Only in extremely performance-critical sections of code might the performance impact become significant. Modern compilers also employ various optimization techniques to minimize the overhead of virtual function calls. Profiling is recommended to identify actual performance bottlenecks in real-world scenarios. Premature optimization based solely on the use of virtual functions is often unnecessary.
The above is the detailed content of What are virtual functions in C and how do they enable polymorphism?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
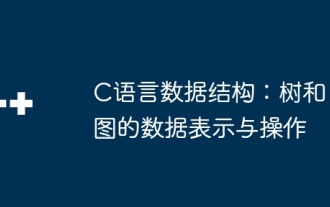
C language data structure: The data representation of the tree and graph is a hierarchical data structure consisting of nodes. Each node contains a data element and a pointer to its child nodes. The binary tree is a special type of tree. Each node has at most two child nodes. The data represents structTreeNode{intdata;structTreeNode*left;structTreeNode*right;}; Operation creates a tree traversal tree (predecision, in-order, and later order) search tree insertion node deletes node graph is a collection of data structures, where elements are vertices, and they can be connected together through edges with right or unrighted data representing neighbors.
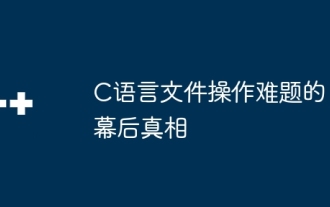
The truth about file operation problems: file opening failed: insufficient permissions, wrong paths, and file occupied. Data writing failed: the buffer is full, the file is not writable, and the disk space is insufficient. Other FAQs: slow file traversal, incorrect text file encoding, and binary file reading errors.
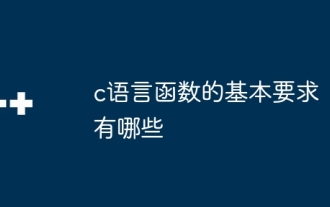
C language functions are the basis for code modularization and program building. They consist of declarations (function headers) and definitions (function bodies). C language uses values to pass parameters by default, but external variables can also be modified using address pass. Functions can have or have no return value, and the return value type must be consistent with the declaration. Function naming should be clear and easy to understand, using camel or underscore nomenclature. Follow the single responsibility principle and keep the function simplicity to improve maintainability and readability.
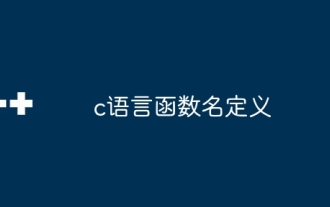
The C language function name definition includes: return value type, function name, parameter list and function body. Function names should be clear, concise and unified in style to avoid conflicts with keywords. Function names have scopes and can be used after declaration. Function pointers allow functions to be passed or assigned as arguments. Common errors include naming conflicts, mismatch of parameter types, and undeclared functions. Performance optimization focuses on function design and implementation, while clear and easy-to-read code is crucial.
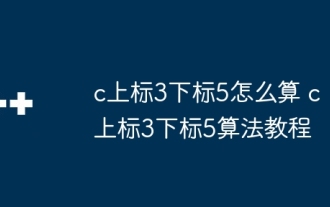
The calculation of C35 is essentially combinatorial mathematics, representing the number of combinations selected from 3 of 5 elements. The calculation formula is C53 = 5! / (3! * 2!), which can be directly calculated by loops to improve efficiency and avoid overflow. In addition, understanding the nature of combinations and mastering efficient calculation methods is crucial to solving many problems in the fields of probability statistics, cryptography, algorithm design, etc.
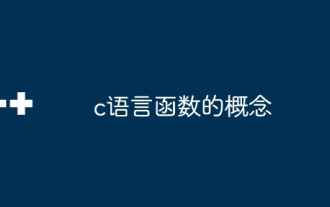
C language functions are reusable code blocks. They receive input, perform operations, and return results, which modularly improves reusability and reduces complexity. The internal mechanism of the function includes parameter passing, function execution, and return values. The entire process involves optimization such as function inline. A good function is written following the principle of single responsibility, small number of parameters, naming specifications, and error handling. Pointers combined with functions can achieve more powerful functions, such as modifying external variable values. Function pointers pass functions as parameters or store addresses, and are used to implement dynamic calls to functions. Understanding function features and techniques is the key to writing efficient, maintainable, and easy to understand C programs.
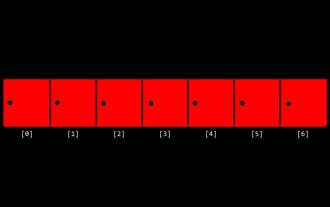
Algorithms are the set of instructions to solve problems, and their execution speed and memory usage vary. In programming, many algorithms are based on data search and sorting. This article will introduce several data retrieval and sorting algorithms. Linear search assumes that there is an array [20,500,10,5,100,1,50] and needs to find the number 50. The linear search algorithm checks each element in the array one by one until the target value is found or the complete array is traversed. The algorithm flowchart is as follows: The pseudo-code for linear search is as follows: Check each element: If the target value is found: Return true Return false C language implementation: #include#includeintmain(void){i
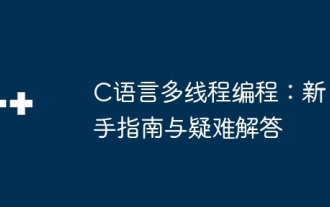
C language multithreading programming guide: Creating threads: Use the pthread_create() function to specify thread ID, properties, and thread functions. Thread synchronization: Prevent data competition through mutexes, semaphores, and conditional variables. Practical case: Use multi-threading to calculate the Fibonacci number, assign tasks to multiple threads and synchronize the results. Troubleshooting: Solve problems such as program crashes, thread stop responses, and performance bottlenecks.
