How does multiple inheritance work in C and what are its challenges?
How Does Multiple Inheritance Work in C and What Are Its Challenges?
Multiple inheritance in C allows a class to inherit from multiple base classes. This means a derived class can acquire the members (data and functions) of all its parent classes. The compiler combines the member declarations from all base classes into the derived class. However, this seemingly straightforward mechanism introduces several complexities.
How it Works: When a derived class inherits from multiple base classes, it inherits all the public and protected members (excluding constructors and destructors, which are not inherited but invoked during object creation and destruction). If there's a name conflict (two base classes have members with the same name), the compiler needs to resolve this ambiguity. This resolution can be done explicitly by using the scope resolution operator (::) to specify which base class's member is intended. For example, if both BaseA
and BaseB
have a function foo()
, and Derived
inherits from both, Derived
would need to use BaseA::foo()
or BaseB::foo()
to disambiguate.
Challenges:
- Ambiguity: The most significant challenge is ambiguity. If multiple base classes have members with the same name, the compiler cannot determine which member to use without explicit disambiguation. This leads to compilation errors.
- Complexity: Managing multiple inheritance can be complex, especially when dealing with virtual functions and virtual inheritance. Understanding the inheritance hierarchy and resolving conflicts can be difficult, making the code harder to maintain and debug.
- Fragile Base Class Problem: Changes in a base class can unintentionally break derived classes, especially if multiple derived classes rely on the same base class in different ways.
- Increased Coupling: Multiple inheritance can increase coupling between classes, making the system less flexible and harder to modify.
What Are the Best Practices to Avoid the Ambiguity Problem in Multiple Inheritance in C ?
Avoiding ambiguity in multiple inheritance requires careful planning and coding practices:
-
Careful Naming: Choose distinct names for members across all base classes. This is the simplest and most effective way to avoid ambiguity. Avoid generic names like
process()
ordata()
. Instead, use descriptive names likeprocessOrder()
orcustomerData()
. -
Explicit Disambiguation: If name conflicts are unavoidable, use the scope resolution operator (::) to explicitly specify which base class's member you are referring to. For instance,
BaseA::foo()
. - Using Namespaces: Encapsulate members within namespaces to avoid name collisions between different base classes or even external libraries.
- Interface Inheritance over Implementation Inheritance: Favor composition over multiple inheritance when possible. This means designing base classes as interfaces defining what functionality should be provided, rather than implementing specific functionality. Derived classes then compose those interfaces, avoiding the complexities of inheritance.
- Virtual Functions (Careful Consideration): While virtual functions can be helpful, they don't automatically resolve ambiguities. Overriding virtual functions from multiple base classes can still create ambiguity if the signatures aren't distinct.
How Can I Effectively Manage Virtual Inheritance to Resolve the Diamond Problem in C Multiple Inheritance?
The "diamond problem" arises when a class inherits from two classes that share a common ancestor. Without virtual inheritance, the common ancestor is duplicated in the derived class's object, leading to data inconsistency and potential errors.
Virtual inheritance solves this by ensuring that only one instance of the common ancestor exists in the derived class's object. This is achieved by declaring the common ancestor as a virtual base class in the intermediate classes.
Example:
class Animal { public: int legs; }; class Mammal : virtual public Animal {}; class Bird : virtual public Animal {}; class Platypus : public Mammal, public Bird {};
In this example, Animal
is a virtual base class for both Mammal
and Bird
. Platypus
will only have one instance of Animal
's legs
member, preventing the diamond problem. Without the virtual
keyword, Platypus
would have two copies of Animal
's members.
Are There Any Alternative Design Patterns to Multiple Inheritance in C That Offer Similar Functionality Without the Complexities?
Yes, several design patterns offer alternatives to multiple inheritance:
- Composition: This involves creating a class that contains instances of other classes as members. This allows a class to utilize the functionality of multiple classes without the complexities of inheritance. This often leads to more flexible and maintainable code.
- Interface Inheritance: This focuses on defining interfaces (pure abstract classes) that specify the behavior a class should implement. Multiple interfaces can be implemented by a single class, achieving similar functionality to multiple inheritance without the ambiguity problems.
- Mixin Classes: These are classes that provide a specific set of functionalities which can be "mixed in" to other classes through composition. They don't represent a complete object on their own but rather extend the capabilities of other classes.
- Strategy Pattern: This pattern defines a family of algorithms, encapsulates each one, and makes them interchangeable. This allows selecting algorithms at runtime without altering the client code.
These alternatives generally lead to more modular, flexible, and maintainable code than multiple inheritance, often making them preferable in C . They promote better code organization and reduce the risk of introducing subtle bugs associated with multiple inheritance.
The above is the detailed content of How does multiple inheritance work in C and what are its challenges?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
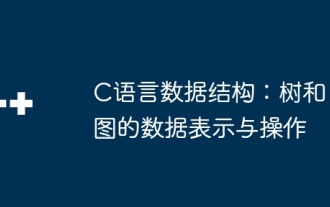
C language data structure: The data representation of the tree and graph is a hierarchical data structure consisting of nodes. Each node contains a data element and a pointer to its child nodes. The binary tree is a special type of tree. Each node has at most two child nodes. The data represents structTreeNode{intdata;structTreeNode*left;structTreeNode*right;}; Operation creates a tree traversal tree (predecision, in-order, and later order) search tree insertion node deletes node graph is a collection of data structures, where elements are vertices, and they can be connected together through edges with right or unrighted data representing neighbors.
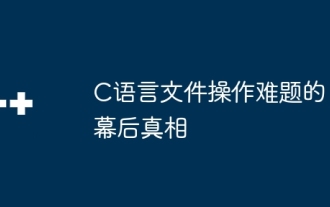
The truth about file operation problems: file opening failed: insufficient permissions, wrong paths, and file occupied. Data writing failed: the buffer is full, the file is not writable, and the disk space is insufficient. Other FAQs: slow file traversal, incorrect text file encoding, and binary file reading errors.
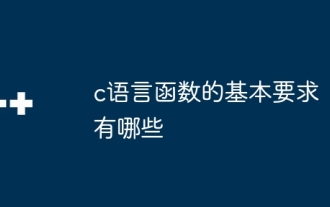
C language functions are the basis for code modularization and program building. They consist of declarations (function headers) and definitions (function bodies). C language uses values to pass parameters by default, but external variables can also be modified using address pass. Functions can have or have no return value, and the return value type must be consistent with the declaration. Function naming should be clear and easy to understand, using camel or underscore nomenclature. Follow the single responsibility principle and keep the function simplicity to improve maintainability and readability.
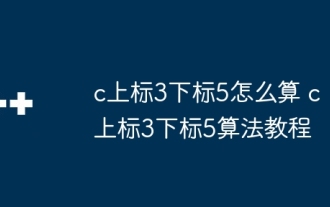
The calculation of C35 is essentially combinatorial mathematics, representing the number of combinations selected from 3 of 5 elements. The calculation formula is C53 = 5! / (3! * 2!), which can be directly calculated by loops to improve efficiency and avoid overflow. In addition, understanding the nature of combinations and mastering efficient calculation methods is crucial to solving many problems in the fields of probability statistics, cryptography, algorithm design, etc.
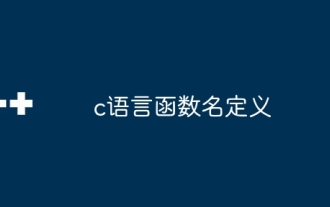
The C language function name definition includes: return value type, function name, parameter list and function body. Function names should be clear, concise and unified in style to avoid conflicts with keywords. Function names have scopes and can be used after declaration. Function pointers allow functions to be passed or assigned as arguments. Common errors include naming conflicts, mismatch of parameter types, and undeclared functions. Performance optimization focuses on function design and implementation, while clear and easy-to-read code is crucial.
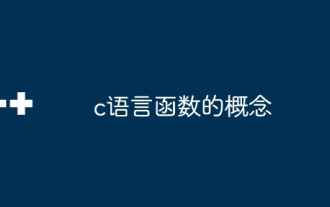
C language functions are reusable code blocks. They receive input, perform operations, and return results, which modularly improves reusability and reduces complexity. The internal mechanism of the function includes parameter passing, function execution, and return values. The entire process involves optimization such as function inline. A good function is written following the principle of single responsibility, small number of parameters, naming specifications, and error handling. Pointers combined with functions can achieve more powerful functions, such as modifying external variable values. Function pointers pass functions as parameters or store addresses, and are used to implement dynamic calls to functions. Understanding function features and techniques is the key to writing efficient, maintainable, and easy to understand C programs.
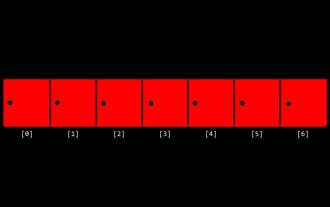
Algorithms are the set of instructions to solve problems, and their execution speed and memory usage vary. In programming, many algorithms are based on data search and sorting. This article will introduce several data retrieval and sorting algorithms. Linear search assumes that there is an array [20,500,10,5,100,1,50] and needs to find the number 50. The linear search algorithm checks each element in the array one by one until the target value is found or the complete array is traversed. The algorithm flowchart is as follows: The pseudo-code for linear search is as follows: Check each element: If the target value is found: Return true Return false C language implementation: #include#includeintmain(void){i
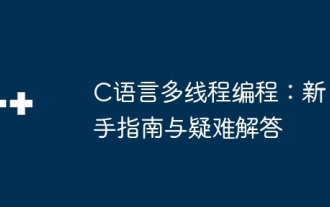
C language multithreading programming guide: Creating threads: Use the pthread_create() function to specify thread ID, properties, and thread functions. Thread synchronization: Prevent data competition through mutexes, semaphores, and conditional variables. Practical case: Use multi-threading to calculate the Fibonacci number, assign tasks to multiple threads and synchronize the results. Troubleshooting: Solve problems such as program crashes, thread stop responses, and performance bottlenecks.
