


How do I use algorithms from the STL (sort, find, transform, etc.) efficiently?
How do I use algorithms from the STL (sort, find, transform, etc.) efficiently?
Efficiently using STL algorithms hinges on understanding their underlying mechanics and applying best practices. Firstly, ensure your data is appropriately organized. For algorithms like sort
, using a vector (dynamic array) is generally more efficient than a list (doubly linked list) because vectors provide contiguous memory access, crucial for many sorting algorithms. Lists require pointer traversal, making sorting significantly slower.
Secondly, understand the algorithm's complexity. sort
typically uses an introspective sort (a hybrid of quicksort, heapsort, and insertion sort) with O(n log n) average-case complexity. However, if you know your data is nearly sorted, std::partial_sort
or even a simple insertion sort might be faster. Similarly, find
has linear O(n) complexity; if you need frequent searches, consider using a std::set
or std::unordered_set
(for unsorted and sorted data respectively) which offer logarithmic or constant time complexity for lookups.
Thirdly, use iterators effectively. STL algorithms operate on iterators, not containers directly. Passing iterators to the beginning and end of a range avoids unnecessary copying of data, improving performance, especially for large datasets. For example, instead of std::sort(myVector)
, use std::sort(myVector.begin(), myVector.end())
. Use the correct iterator type (e.g., const_iterator
if you don't need to modify the data).
Finally, consider using execution policies. For algorithms supporting parallel execution (like std::sort
), using execution policies like std::execution::par
or std::execution::par_unseq
can significantly speed up processing on multi-core machines, especially for large datasets. However, remember that the overhead of parallelization might outweigh the benefits for small datasets.
What are the common pitfalls to avoid when using STL algorithms?
Several common pitfalls can hinder the efficiency and correctness of STL algorithm usage:
- Incorrect iterator ranges: Providing incorrect start or end iterators is a frequent error, leading to undefined behavior or incorrect results. Always double-check your iterator ranges.
- Modifying containers during algorithm execution: Modifying the container being processed by an algorithm (e.g., adding or removing elements) while the algorithm is running can lead to unpredictable results, crashes, or data corruption.
- Ignoring algorithm preconditions: Many STL algorithms have preconditions (e.g., sorted input for certain algorithms). Failing to meet these preconditions can result in incorrect output or undefined behavior.
-
Inefficient data structures: Choosing the wrong data structure for the task can significantly impact performance. For example, using a
std::list
when astd::vector
would be more appropriate for frequent random access. - Unnecessary copies: Avoid unnecessary copying of data. Use iterators to process data in-place whenever possible.
- Overuse of algorithms: For simple operations, a custom loop might be more efficient than using a general-purpose STL algorithm. Profiling your code can help determine if an STL algorithm is truly necessary.
How can I choose the most efficient STL algorithm for a specific task?
Selecting the most efficient STL algorithm requires understanding the task's requirements and the algorithms' characteristics:
- Identify the operation: Determine what needs to be done (sorting, searching, transforming, etc.).
- Analyze the data: Consider the data's size, organization (sorted, unsorted), and properties.
-
Choose the appropriate algorithm: Based on the operation and data characteristics, select the algorithm with the best time and space complexity. For example, for searching in a sorted range,
std::lower_bound
orstd::binary_search
are more efficient thanstd::find
. For transforming data, considerstd::transform
orstd::for_each
. - Consider parallelization: If the dataset is large and the algorithm supports parallel execution, explore using execution policies for potential performance gains.
- Profile and benchmark: After choosing an algorithm, measure its performance using profiling tools to ensure it meets your requirements. Compare different algorithms to validate your choice.
Are there performance differences between different STL algorithms for the same task, and how can I measure them?
Yes, significant performance differences can exist between different STL algorithms designed for similar tasks. For instance, std::sort
might outperform a custom insertion sort for large, unsorted datasets, but the custom sort might be faster for small, nearly-sorted datasets. Similarly, std::find
is linear, while searching a std::set
is logarithmic.
To measure these differences, use profiling tools and benchmarking techniques:
- Profiling tools: Tools like gprof (for Linux) or Visual Studio Profiler (for Windows) can help identify performance bottlenecks in your code, showing the time spent in different functions, including STL algorithms.
-
Benchmarking: Create test cases with varying data sizes and characteristics. Time the execution of different algorithms using high-resolution timers (e.g.,
std::chrono
in C ). Repeat the measurements multiple times and average the results to minimize noise. - Statistical analysis: Use statistical methods to compare the performance results and determine if the differences are statistically significant.
By combining profiling and benchmarking, you can accurately assess the performance of different STL algorithms and make informed decisions for your specific needs. Remember to test with representative datasets to get meaningful results.
The above is the detailed content of How do I use algorithms from the STL (sort, find, transform, etc.) efficiently?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


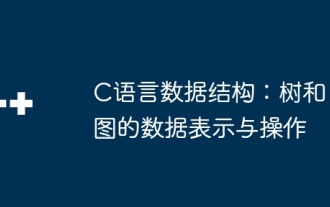
C language data structure: The data representation of the tree and graph is a hierarchical data structure consisting of nodes. Each node contains a data element and a pointer to its child nodes. The binary tree is a special type of tree. Each node has at most two child nodes. The data represents structTreeNode{intdata;structTreeNode*left;structTreeNode*right;}; Operation creates a tree traversal tree (predecision, in-order, and later order) search tree insertion node deletes node graph is a collection of data structures, where elements are vertices, and they can be connected together through edges with right or unrighted data representing neighbors.
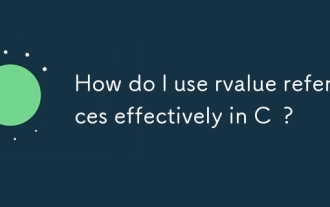
Article discusses effective use of rvalue references in C for move semantics, perfect forwarding, and resource management, highlighting best practices and performance improvements.(159 characters)
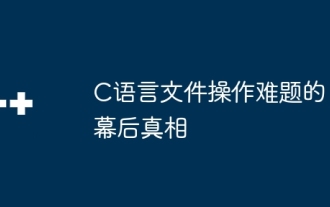
The truth about file operation problems: file opening failed: insufficient permissions, wrong paths, and file occupied. Data writing failed: the buffer is full, the file is not writable, and the disk space is insufficient. Other FAQs: slow file traversal, incorrect text file encoding, and binary file reading errors.
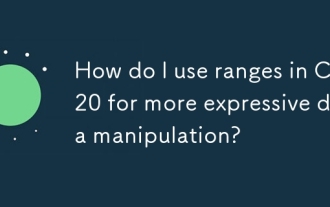
C 20 ranges enhance data manipulation with expressiveness, composability, and efficiency. They simplify complex transformations and integrate into existing codebases for better performance and maintainability.
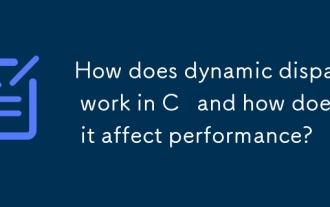
The article discusses dynamic dispatch in C , its performance costs, and optimization strategies. It highlights scenarios where dynamic dispatch impacts performance and compares it with static dispatch, emphasizing trade-offs between performance and
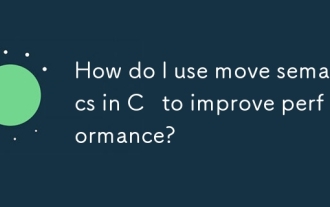
The article discusses using move semantics in C to enhance performance by avoiding unnecessary copying. It covers implementing move constructors and assignment operators, using std::move, and identifies key scenarios and pitfalls for effective appl
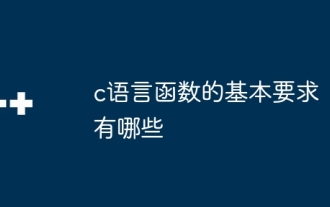
C language functions are the basis for code modularization and program building. They consist of declarations (function headers) and definitions (function bodies). C language uses values to pass parameters by default, but external variables can also be modified using address pass. Functions can have or have no return value, and the return value type must be consistent with the declaration. Function naming should be clear and easy to understand, using camel or underscore nomenclature. Follow the single responsibility principle and keep the function simplicity to improve maintainability and readability.
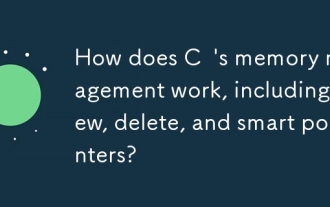
C memory management uses new, delete, and smart pointers. The article discusses manual vs. automated management and how smart pointers prevent memory leaks.
