How can I use Yii for building single-page applications (SPAs)?
How to Use Yii for Building Single-Page Applications (SPAs)
Yii, a high-performance PHP framework, isn't traditionally designed for building SPAs in the same way as JavaScript frameworks like React or Vue. SPAs rely heavily on client-side JavaScript to handle most of the application logic and dynamically update the UI without full page reloads. Yii, on the other hand, excels at building server-side applications. Therefore, using Yii for SPAs involves a different approach: Yii acts as a powerful RESTful API backend, providing data and functionality to the SPA frontend, which is built using a JavaScript framework.
The process typically involves:
- Designing a RESTful API: You'll define API endpoints in Yii using controllers and actions that return data in formats like JSON. This data will be consumed by your SPA frontend. Yii's built-in support for RESTful APIs makes this process relatively straightforward. You can leverage Yii's ActiveRecord to easily map database models to your API responses.
- Creating the SPA Frontend: You'll use a JavaScript framework (like React, Angular, or Vue.js – discussed later) to build the user interface of your SPA. This frontend will make AJAX requests to the Yii backend API to fetch and manipulate data.
-
Connecting Frontend and Backend: The frontend uses techniques like
fetch
or libraries like Axios to communicate with the Yii backend's API endpoints. The Yii backend processes these requests, retrieves or manipulates data, and returns JSON responses that the frontend can interpret and display. - Authentication and Authorization: Implement robust authentication and authorization mechanisms on both the frontend and backend. Common approaches include using JSON Web Tokens (JWTs) for authentication. Yii provides tools to manage user authentication and authorization, and you can integrate these with your chosen JavaScript framework's authentication methods.
- State Management: For complex SPAs, consider using a state management library in your JavaScript framework (e.g., Redux, Vuex) to handle data flow and maintain application state efficiently.
Best Practices for Integrating a Yii Backend with a SPA Frontend
Several best practices ensure a smooth and efficient integration:
-
API Versioning: Implement API versioning (e.g., using URL prefixes like
/v1/users
or HTTP headers) to allow for future changes to your API without breaking existing frontend code. - Clear API Documentation: Use tools like Swagger or OpenAPI to generate comprehensive documentation for your Yii API. This is crucial for both development and maintenance.
- Consistent Data Formats: Maintain consistent data formats (JSON) across all API responses for easy parsing by the frontend.
- Error Handling: Implement proper error handling on both the backend (Yii) and frontend (JavaScript framework). Return meaningful error messages in JSON format to help the frontend gracefully handle errors.
- Security: Prioritize security best practices, including input validation, output encoding, and secure authentication mechanisms (e.g., JWTs, OAuth 2.0). Use HTTPS for all communication between the frontend and backend.
- Rate Limiting: Implement rate limiting on your Yii backend to protect against abuse and denial-of-service attacks.
- Caching: Employ caching strategies (e.g., browser caching, server-side caching using Yii's caching components) to improve performance.
Popular JavaScript Frameworks that Work Well with Yii for Building SPAs
Several popular JavaScript frameworks integrate well with a Yii backend:
- React: A component-based library that allows for building complex and reusable UI components. Its virtual DOM makes updates efficient. React's popularity and vast ecosystem make it a strong choice.
- Angular: A comprehensive framework offering a complete solution for building SPAs, including routing, state management, and dependency injection. Angular's structure can be beneficial for large projects.
- Vue.js: A progressive framework known for its ease of use and gentle learning curve. Vue.js is highly flexible and can be easily integrated with existing projects.
The choice depends on project requirements, team expertise, and personal preference. All three frameworks provide robust tools for handling AJAX requests and managing data fetched from a Yii backend.
Common Challenges Encountered When Developing SPAs with Yii, and How to Overcome Them
Developing SPAs with a Yii backend presents some unique challenges:
- Cross-Origin Resource Sharing (CORS): If your Yii backend and SPA frontend are hosted on different domains, you'll need to configure CORS headers on your Yii backend to allow the frontend to make requests. Yii provides mechanisms for setting these headers.
- Data Synchronization: Maintaining data consistency between the frontend and backend can be challenging. Using optimistic or pessimistic locking strategies can help prevent data conflicts.
- Debugging: Debugging across both frontend and backend can be more complex. Use browser developer tools to debug the frontend and Yii's debugging tools (or Xdebug) for the backend.
- Performance Optimization: Large SPAs can become slow if not optimized. Use techniques like code splitting, lazy loading, and caching to improve performance. Optimizing your Yii backend queries is also crucial.
- State Management: Managing application state in complex SPAs requires careful planning. Employing a state management library in your chosen JavaScript framework (like Redux, Vuex, or Pinia) is highly recommended.
Overcoming these challenges requires careful planning, well-defined API design, robust error handling, and the use of appropriate tools and libraries. Thorough testing is also essential to ensure a smooth and reliable SPA.
The above is the detailed content of How can I use Yii for building single-page applications (SPAs)?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


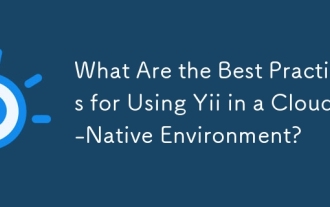
The article discusses best practices for deploying Yii applications in cloud-native environments, focusing on scalability, reliability, and efficiency through containerization, orchestration, and security measures.
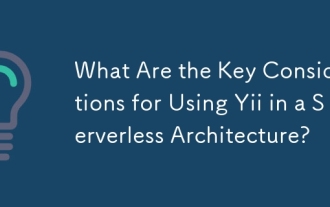
The article discusses key considerations for using Yii in serverless architectures, focusing on statelessness, cold starts, function size, database interactions, security, and monitoring. It also covers optimization strategies and potential integrati
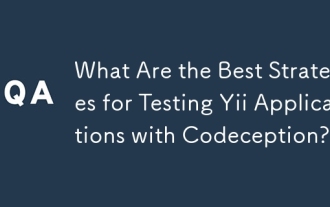
The article discusses strategies for testing Yii applications using Codeception, focusing on using built-in modules, BDD, different test types, mocking, CI integration, and code coverage.
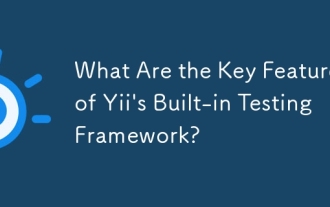
Yii's built-in testing framework enhances application testing with features like PHPUnit integration, fixture management, and support for various test types, improving code quality and development practices.
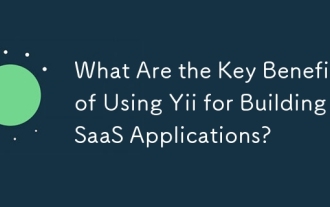
The article discusses Yii's benefits for SaaS development, focusing on performance, security, and rapid development features to enhance scalability and reduce time-to-market.
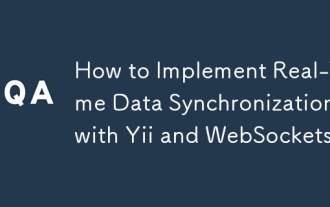
The article discusses implementing real-time data synchronization using Yii and WebSockets, covering setup, integration, and best practices for performance and security.
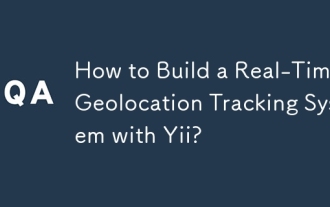
Article discusses building real-time geolocation tracking with Yii, covering setup, database design, and security. Main focus is on integration and best practices for data privacy and security.

The article discusses implementing service discovery and load balancing in Yii microservices, detailing steps and best practices for efficient communication and workload distribution.
