


How can I connect to NoSQL databases like MongoDB or Redis with ThinkPHP?
Connecting to NoSQL Databases (MongoDB & Redis) with ThinkPHP
ThinkPHP, a popular PHP framework, doesn't offer built-in support for NoSQL databases like MongoDB or Redis directly. However, you can connect to them using their respective PHP drivers. For MongoDB, you'll use the mongodb
driver (often a part of the mongodb
PECL extension or a Composer package). For Redis, you'll need the predis
or phpredis
extension.
First, you need to install the necessary drivers. If using Composer, add the appropriate package to your composer.json
file:
{ "require": { "mongodb/mongodb": "^1.11", "predis/predis": "^2.0" } }
Then run composer update
. After installation, you can create a connection within your ThinkPHP application. This typically involves creating a model or service class to handle database interactions. For example, a MongoDB connection might look like this:
<?php namespace app\model; use MongoDB\Client; class MongoModel { private $client; private $collection; public function __construct() { $this->client = new Client("mongodb://localhost:27017"); // Replace with your connection string $this->collection = $this->client->selectDatabase('your_database')->selectCollection('your_collection'); } public function insertData($data) { return $this->collection->insertOne($data); } // ... other methods for finding, updating, deleting data ... }
And for Redis:
<?php namespace app\service; use Predis\Client; class RedisService { private $client; public function __construct() { $this->client = new Client([ 'scheme' => 'tcp', 'host' => '127.0.0.1', 'port' => 6379, ]); } public function setData($key, $value) { return $this->client->set($key, $value); } // ... other methods for getting, deleting, etc. data ... }
Remember to replace placeholders like database names, collection names, and connection strings with your actual values. You would then inject these classes into your controllers or other parts of your ThinkPHP application using dependency injection.
Best Practices for Using NoSQL Databases with ThinkPHP
- Schema Design: Carefully plan your NoSQL database schema. Unlike relational databases, NoSQL databases are schema-less, but a well-defined structure is crucial for efficient querying and data management. Consider data modeling and how your application will interact with the data.
- Data Modeling: Choose the appropriate NoSQL database type (document, key-value, graph) based on your data structure and access patterns. MongoDB is suitable for document-oriented data, while Redis excels as a key-value store.
- Transactions: NoSQL databases generally don't support ACID transactions in the same way as relational databases. Consider using alternative strategies for data consistency, such as optimistic locking or implementing your own transaction logic within your ThinkPHP application.
- Error Handling: Implement robust error handling to gracefully manage connection failures, data inconsistencies, and other potential issues.
- Data Validation: Validate data before inserting it into the NoSQL database to prevent inconsistencies and errors. ThinkPHP's validation capabilities can be used for this purpose.
- Caching: Utilize caching mechanisms (e.g., Redis) to improve application performance by storing frequently accessed data in memory.
ThinkPHP Extensions and Libraries for NoSQL Integration
There aren't widely used, officially supported ThinkPHP extensions specifically designed for seamless NoSQL integration. The approach described in the first section (using the native PHP drivers) is the most common and reliable method. While some community-contributed packages might exist, they often lack comprehensive support and regular updates. Therefore, relying on the official PHP drivers is generally recommended for stability and maintainability.
Performance Considerations when Connecting ThinkPHP to NoSQL Databases
- Connection Pooling: For improved performance, use connection pooling to reuse database connections instead of creating a new connection for each request. The PHP drivers often provide mechanisms for connection pooling.
- Query Optimization: Optimize your queries to minimize database load. Use appropriate indexes (where applicable, such as in MongoDB) and avoid inefficient query patterns.
- Data Serialization: Choose efficient data serialization formats (e.g., JSON) when interacting with NoSQL databases.
- Caching: Implement aggressive caching strategies to reduce the number of database queries. Redis is an excellent choice for this purpose.
- Asynchronous Operations: Consider using asynchronous operations (if supported by the chosen driver and database) to avoid blocking the main application thread during long-running database operations.
- Database Choice: Select the appropriate NoSQL database based on your application's specific needs and performance requirements. For example, Redis is extremely fast for caching and key-value operations, while MongoDB is better suited for flexible document storage. Choosing the wrong database can significantly impact performance.
The above is the detailed content of How can I connect to NoSQL databases like MongoDB or Redis with ThinkPHP?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


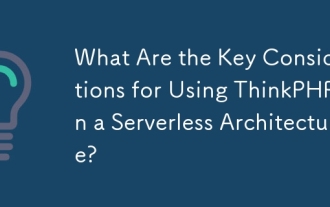
The article discusses key considerations for using ThinkPHP in serverless architectures, focusing on performance optimization, stateless design, and security. It highlights benefits like cost efficiency and scalability, but also addresses challenges
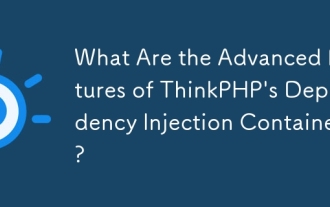
ThinkPHP's IoC container offers advanced features like lazy loading, contextual binding, and method injection for efficient dependency management in PHP apps.Character count: 159
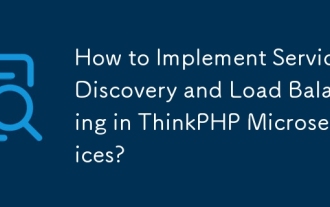
The article discusses implementing service discovery and load balancing in ThinkPHP microservices, focusing on setup, best practices, integration methods, and recommended tools.[159 characters]
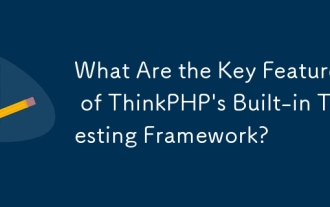
The article discusses ThinkPHP's built-in testing framework, highlighting its key features like unit and integration testing, and how it enhances application reliability through early bug detection and improved code quality.
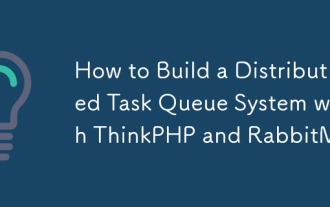
The article outlines building a distributed task queue system using ThinkPHP and RabbitMQ, focusing on installation, configuration, task management, and scalability. Key issues include ensuring high availability, avoiding common pitfalls like imprope
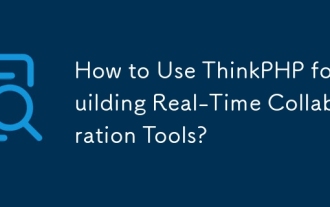
The article discusses using ThinkPHP to build real-time collaboration tools, focusing on setup, WebSocket integration, and security best practices.
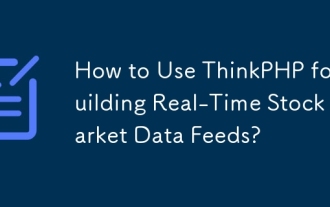
Article discusses using ThinkPHP for real-time stock market data feeds, focusing on setup, data accuracy, optimization, and security measures.

ThinkPHP benefits SaaS apps with its lightweight design, MVC architecture, and extensibility. It enhances scalability, speeds development, and improves security through various features.
