How do I create and use custom view helpers in ThinkPHP?
Creating and Using Custom View Helpers in ThinkPHP
ThinkPHP's flexibility allows for the creation of custom view helpers to streamline repetitive tasks and enhance code readability within your templates. To create a custom view helper, you need to define a class that extends the Think\Template\TagLib
class. This class will contain methods that represent your custom helper functions. Let's create a simple example: a helper to format dates.
First, create a file named DateHelper.php
(you can choose any name, but follow a consistent naming convention) within your application's Library/Think/Template/TagLib
directory (or create this directory if it doesn't exist). Inside this file, add the following code:
<?php namespace Think\Template\TagLib; class DateHelper extends \Think\Template\TagLib { public function formatDate($date, $format = 'Y-m-d') { return date($format, strtotime($date)); } }
This formatDate
method takes a date string and an optional format string as parameters. It then uses PHP's date()
function to format the date accordingly.
To use this helper in your template, you would call it like this:
{$Think.template.DateHelper->formatDate($myDate, 'F j, Y')}
Replacing $myDate
with your date variable. This will output the date formatted according to the specified format. Remember that you need to ensure your $myDate
variable is correctly defined within your template's context.
Best Practices for Organizing Custom View Helpers in a ThinkPHP Project
Organizing your custom view helpers effectively is crucial for maintainability and scalability. Here's a recommended approach:
- Directory Structure: Create a dedicated directory within your
Library/Think/Template/TagLib
directory to house your custom helpers. You might structure it based on functionality (e.g.,Library/Think/Template/TagLib/Helpers/Date
,Library/Think/Template/TagLib/Helpers/String
,Library/Think/Template/TagLib/Helpers/Form
). This keeps related helpers grouped together. - Naming Conventions: Use a consistent naming convention for your helper classes (e.g.,
CamelCase
orsnake_case
). This improves readability and makes it easier to find specific helpers. The helper method names should also be descriptive and follow a consistent style. - Modular Design: Break down complex tasks into smaller, more manageable helper methods. This promotes reusability and reduces code duplication.
- Documentation: Document your helpers clearly, including parameters, return values, and usage examples. This is essential for other developers (and your future self) to understand how to use them. Use PHPDoc style comments for best practice.
Passing Parameters to Custom ThinkPHP View Helpers
You can pass parameters to your custom view helpers just like in the formatDate
example above. The parameters are passed as arguments to the helper method. For example, let's extend the DateHelper
to include a helper for calculating the difference between two dates:
<?php namespace Think\Template\TagLib; class DateHelper extends \Think\Template\TagLib { // ... (formatDate method from previous example) ... public function dateDiff($date1, $date2, $unit = 'day') { $diff = abs(strtotime($date2) - strtotime($date1)); switch ($unit) { case 'day': return floor($diff / (60 * 60 * 24)); case 'hour': return floor($diff / (60 * 60)); case 'minute': return floor($diff / 60); case 'second': return $diff; default: return 0; // Or handle invalid unit appropriately } } }
This dateDiff
method accepts two dates and an optional unit ('day', 'hour', 'minute', 'second') as parameters. You can then call it in your template like this:
{$Think.template.DateHelper->dateDiff($startDate, $endDate, 'day')}
Debugging Issues with Custom ThinkPHP View Helpers
Debugging custom view helpers can be straightforward using standard PHP debugging techniques.
-
Error Reporting: Ensure that your PHP error reporting is enabled (e.g., using
error_reporting(E_ALL);
in your application's bootstrap file) to catch any syntax errors or runtime exceptions. -
var_dump()
andprint_r()
: Use these functions within your helper methods to inspect the values of variables and ensure they are as expected. Remember to remove or comment out these debugging statements once you've identified the issue. - Logging: Implement logging within your helpers to track the execution flow and values of variables. This is particularly helpful when dealing with complex logic or asynchronous operations.
- IDE Debugging: Utilize your IDE's debugging capabilities to step through the code line by line, inspect variables, and identify the source of errors. Set breakpoints within your helper methods to pause execution at specific points.
-
Check Template Context: Ensure that the variables you are passing to your helper methods are correctly defined and accessible within the template's context. Incorrect variable names or missing variables are common sources of errors. Use
var_dump($this->vars)
inside your helper to check available variables.
By following these guidelines, you can create, organize, and debug your custom view helpers effectively, leading to cleaner, more maintainable ThinkPHP applications.
The above is the detailed content of How do I create and use custom view helpers in ThinkPHP?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


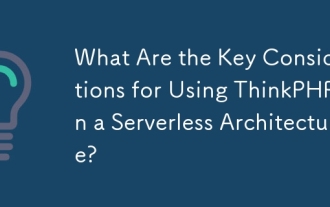
The article discusses key considerations for using ThinkPHP in serverless architectures, focusing on performance optimization, stateless design, and security. It highlights benefits like cost efficiency and scalability, but also addresses challenges
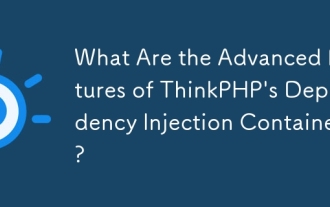
ThinkPHP's IoC container offers advanced features like lazy loading, contextual binding, and method injection for efficient dependency management in PHP apps.Character count: 159
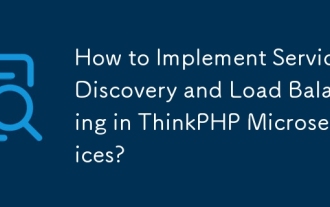
The article discusses implementing service discovery and load balancing in ThinkPHP microservices, focusing on setup, best practices, integration methods, and recommended tools.[159 characters]
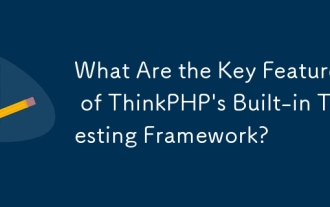
The article discusses ThinkPHP's built-in testing framework, highlighting its key features like unit and integration testing, and how it enhances application reliability through early bug detection and improved code quality.
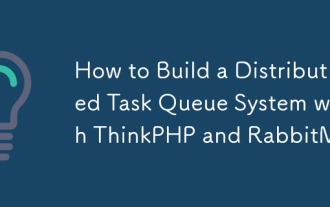
The article outlines building a distributed task queue system using ThinkPHP and RabbitMQ, focusing on installation, configuration, task management, and scalability. Key issues include ensuring high availability, avoiding common pitfalls like imprope
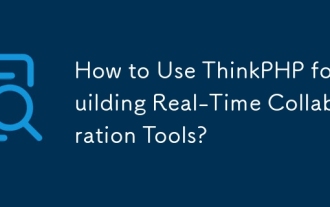
The article discusses using ThinkPHP to build real-time collaboration tools, focusing on setup, WebSocket integration, and security best practices.
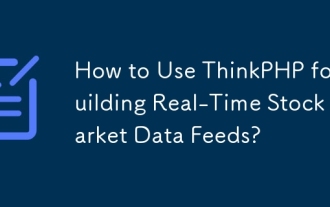
Article discusses using ThinkPHP for real-time stock market data feeds, focusing on setup, data accuracy, optimization, and security measures.

ThinkPHP benefits SaaS apps with its lightweight design, MVC architecture, and extensibility. It enhances scalability, speeds development, and improves security through various features.
