How do I implement dependency injection in ThinkPHP applications?
How to Implement Dependency Injection in ThinkPHP Applications?
ThinkPHP, while not inherently built with a built-in dependency injection (DI) container like Laravel, allows for the implementation of DI through several approaches. The most common and straightforward method involves using constructor injection. This means passing dependencies as arguments to a class's constructor.
Let's say you have a UserService
class that depends on a UserRepository
class:
// UserRepository.php class UserRepository { public function getUserById($id) { // ... database logic to retrieve user ... return ['id' => $id, 'name' => 'John Doe']; } } // UserService.php class UserService { private $userRepository; public function __construct(UserRepository $userRepository) { $this->userRepository = $userRepository; } public function getUserProfile($id) { $user = $this->userRepository->getUserById($id); // ... additional logic to process user data ... return $user; } }
In your controller or other part of your application, you would then instantiate UserService
and explicitly pass the UserRepository
instance:
// UserController.php class UserController extends Controller { public function profile($id) { $userRepository = new UserRepository(); // Or retrieve from a service container if you're using one. $userService = new UserService($userRepository); $profile = $userService->getUserProfile($id); $this->assign('profile', $profile); $this->display(); } }
This manual instantiation works well for smaller projects. For larger applications, a more robust approach using a service container (discussed in the next section) is recommended.
What are the Best Practices for Using Dependency Injection in ThinkPHP?
Following best practices when implementing DI in ThinkPHP ensures maintainability, testability, and scalability. Key best practices include:
- Favor Constructor Injection: Always prioritize constructor injection over setter injection or interface injection. This makes dependencies explicit and ensures that an object is properly initialized before use.
- Interface-Based Dependencies: Whenever possible, define interfaces for your dependencies rather than directly injecting concrete classes. This allows for easier swapping of implementations (e.g., for testing or using different data sources).
- Use a Service Container (for larger projects): For larger applications, a service container significantly improves the management of dependencies. It centralizes the creation and configuration of objects, simplifying dependency resolution and reducing boilerplate code.
- Keep Dependencies Concise: Avoid creating classes with an excessive number of dependencies. This indicates potential design issues and makes the class harder to test and maintain. Consider refactoring into smaller, more focused classes if necessary.
- Test Thoroughly: Unit testing becomes significantly easier with DI. You can easily mock or stub dependencies during testing, isolating the unit under test.
Can I Use a Specific Dependency Injection Container with ThinkPHP, and If So, How?
Yes, you can integrate a third-party dependency injection container with ThinkPHP. Popular choices include Pimple, Symfony's DependencyInjection component, or a more full-featured container like Aura.Di.
The integration typically involves:
- Installation: Install the chosen container via Composer.
- Configuration: Configure the container to register your services (classes and their dependencies).
- Dependency Resolution: Use the container to resolve dependencies when creating your objects.
Example using Pimple (a lightweight container):
// config/container.php $container = new Pimple\Container(); $container['userRepository'] = function ($c) { return new UserRepository(); }; $container['userService'] = function ($c) { return new UserService($c['userRepository']); }; // In your controller: $userService = $container['userService']; $profile = $userService->getUserProfile($id);
This example shows how to register UserRepository
and UserService
with Pimple, and then retrieve an instance of UserService
which automatically receives the properly injected UserRepository
instance.
What are the Benefits of Using Dependency Injection in My ThinkPHP Project?
Implementing DI in your ThinkPHP project offers several significant advantages:
- Loose Coupling: DI reduces the coupling between different parts of your application, making the code more modular, flexible, and easier to maintain. Changes in one part of the application are less likely to have cascading effects on other parts.
- Improved Testability: DI makes unit testing significantly easier. You can easily mock or stub dependencies during testing, isolating the unit under test and ensuring more reliable tests.
- Enhanced Reusability: Components designed with DI are more reusable in different contexts. They can be easily integrated into other projects or used with different implementations of their dependencies.
- Better Code Organization: DI promotes better code organization and structure, making the codebase easier to understand and navigate.
- Simplified Development: While there's an initial learning curve, DI ultimately simplifies development by making the code more manageable and less prone to errors as your project grows. It makes refactoring and extending functionalities easier in the long run.
The above is the detailed content of How do I implement dependency injection in ThinkPHP applications?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


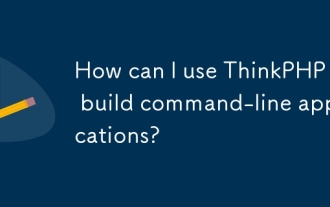
This article demonstrates building command-line applications (CLIs) using ThinkPHP's CLI capabilities. It emphasizes best practices like modular design, dependency injection, and robust error handling, while highlighting common pitfalls such as insu
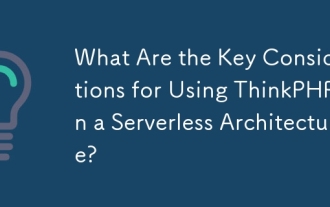
The article discusses key considerations for using ThinkPHP in serverless architectures, focusing on performance optimization, stateless design, and security. It highlights benefits like cost efficiency and scalability, but also addresses challenges
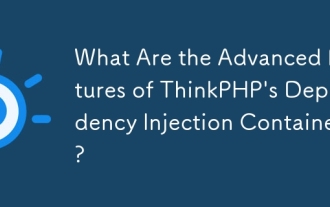
ThinkPHP's IoC container offers advanced features like lazy loading, contextual binding, and method injection for efficient dependency management in PHP apps.Character count: 159
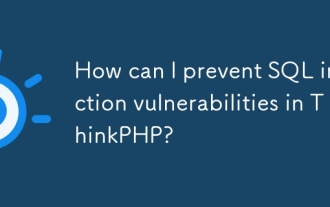
The article discusses preventing SQL injection vulnerabilities in ThinkPHP through parameterized queries, avoiding raw SQL, using ORM, regular updates, and proper error handling. It also covers best practices for securing database queries and validat
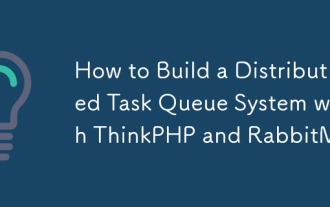
The article outlines building a distributed task queue system using ThinkPHP and RabbitMQ, focusing on installation, configuration, task management, and scalability. Key issues include ensuring high availability, avoiding common pitfalls like imprope
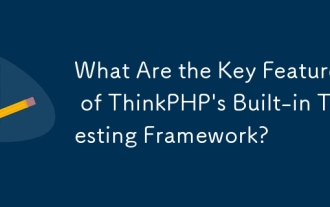
The article discusses ThinkPHP's built-in testing framework, highlighting its key features like unit and integration testing, and how it enhances application reliability through early bug detection and improved code quality.
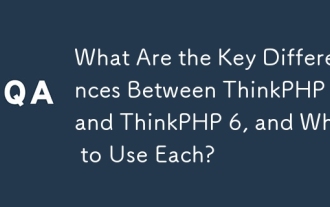
The article discusses key differences between ThinkPHP 5 and 6, focusing on architecture, features, performance, and suitability for legacy upgrades. ThinkPHP 5 is recommended for traditional projects and legacy systems, while ThinkPHP 6 suits new pr
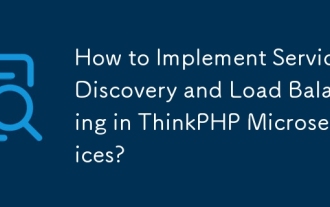
The article discusses implementing service discovery and load balancing in ThinkPHP microservices, focusing on setup, best practices, integration methods, and recommended tools.[159 characters]
