


What Are the Best Ways to Handle File Uploads and Downloads with Nginx?
What Are the Best Ways to Handle File Uploads and Downloads with Nginx?
Nginx, by itself, isn't designed for handling file uploads and downloads directly in the way a dedicated application server like Apache might be. It excels at acting as a reverse proxy and load balancer, making it ideal for serving static files efficiently but less so for managing the complex process of file uploads. The best way to handle file uploads and downloads with Nginx is to use it in conjunction with a backend application server (e.g., Node.js, Python with Flask or Django, Java with Spring, etc.).
This approach leverages Nginx's strengths:
- Efficient Static File Serving: Nginx serves static files (like downloaded files) incredibly fast, handling many concurrent connections with minimal resource consumption. Your backend application only needs to handle the actual upload/download process and then instruct Nginx where the files reside.
- Reverse Proxy: Nginx acts as a reverse proxy, forwarding upload requests to the application server and then relaying the response back to the client. This adds a layer of security and abstraction.
- Load Balancing: For high traffic, multiple application servers can be load balanced behind Nginx, ensuring high availability and scalability.
The workflow typically looks like this:
- Client initiates upload: The client sends the file upload request to Nginx.
- Nginx forwards request: Nginx forwards the request to the backend application server.
- Application server handles upload: The application server receives the file, processes it (e.g., validation, storage), and returns a success or failure response.
- Application server informs Nginx (if necessary): If Nginx needs to directly serve the uploaded file, the application server informs Nginx of the file's location.
- Client initiates download: The client requests the downloaded file from Nginx.
- Nginx serves file: Nginx efficiently serves the file directly from its storage location.
This architecture separates concerns, resulting in a robust and performant system.
How can I optimize Nginx for efficient large file uploads and downloads?
Optimizing Nginx for large file uploads and downloads involves several strategies:
-
sendfile
andaio
: Enablingsendfile
allows Nginx to efficiently transfer files directly from the kernel's buffer to the client, bypassing user space copying.aio
(Asynchronous I/O) enables asynchronous operations, improving concurrency. These are typically enabled by default but should be verified in your configuration. -
tcp_nopush
: This directive can improve performance, especially on slower connections, by reducing the number of packets sent. Experiment to see if it benefits your specific setup. -
client_max_body_size
: This directive sets the maximum size of the client request body (the uploaded file). Set it appropriately to prevent excessively large files from overwhelming the server. - Caching: While not directly related to the upload/download process itself, caching static files (e.g., frequently accessed downloaded files) significantly improves performance. Nginx offers powerful caching mechanisms.
-
Multiple worker processes: Increase the number of worker processes (
worker_processes
) in your Nginx configuration to handle more concurrent uploads and downloads. The optimal number depends on your server's resources (CPU cores, RAM). - Hardware considerations: Sufficient disk I/O performance is crucial. Using SSDs instead of HDDs significantly speeds up file access. Network bandwidth is also a limiting factor for large file transfers.
What security considerations should I address when implementing file uploads and downloads with Nginx?
Security is paramount when handling file uploads and downloads. Consider these aspects:
- Input Validation: Thoroughly validate all uploaded files on the application server side. Check file types, sizes, and content to prevent malicious uploads (e.g., executable files, scripts).
- File Storage Location: Store uploaded files in a location inaccessible to the web server's user. This prevents direct access to the files without going through the application server.
-
Content-Type Checking: Verify the
Content-Type
header in upload requests to ensure it matches the actual file type. - Protection against Directory Traversal Attacks: Carefully sanitize file paths to prevent attackers from accessing files outside the intended directory. Never directly use user-supplied input in file paths.
- HTTPS: Always use HTTPS to encrypt communication between clients and the server, protecting data in transit.
- Regular Security Updates: Keep Nginx and all related software up-to-date with the latest security patches.
- Rate Limiting: Implement rate limiting to prevent denial-of-service attacks (DoS) where a large number of requests overwhelm the server.
- Authentication and Authorization: Ensure that only authorized users can upload and download files. Use appropriate authentication and authorization mechanisms (e.g., OAuth, JWT).
What are the common Nginx configuration settings for managing file uploads and downloads, and how do I troubleshoot common issues?
Common Nginx configuration settings for file uploads and downloads are primarily related to the reverse proxy setup and handling large requests. They're not directly managing the upload/download process itself, as that's handled by the backend application. Here are some examples:
-
client_max_body_size
: (already mentioned above) Defines the maximum allowed size for client request bodies. -
location
block: This block defines how Nginx handles requests to specific paths. You'd use alocation
block to route upload requests to your application server usingproxy_pass
. Example:
location /upload { proxy_pass http://backend-app-server:3000/upload; proxy_set_header Host $host; proxy_set_header X-Real-IP $remote_addr; } location /downloads { alias /path/to/downloads; # Path to your downloads directory }
Troubleshooting:
- Upload failures: Check server logs for errors. Common issues include insufficient disk space, incorrect file permissions, or problems with the backend application server.
-
Slow downloads: Check network connectivity, disk I/O performance, and Nginx configuration (e.g.,
sendfile
,aio
). Analyze Nginx logs for slow requests. -
413 Request Entity Too Large: This error indicates that the uploaded file exceeds
client_max_body_size
. Increase this value if necessary. - 502 Bad Gateway: This often indicates a problem with the backend application server. Check its logs for errors.
Remember to always test your configuration thoroughly and monitor your server's performance to identify and address potential bottlenecks. Proper logging is essential for effective troubleshooting.
The above is the detailed content of What Are the Best Ways to Handle File Uploads and Downloads with Nginx?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

AI Hentai Generator
Generate AI Hentai for free.

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics


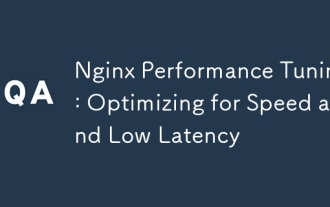
Nginx performance tuning can be achieved by adjusting the number of worker processes, connection pool size, enabling Gzip compression and HTTP/2 protocols, and using cache and load balancing. 1. Adjust the number of worker processes and connection pool size: worker_processesauto; events{worker_connections1024;}. 2. Enable Gzip compression and HTTP/2 protocol: http{gzipon;server{listen443sslhttp2;}}. 3. Use cache optimization: http{proxy_cache_path/path/to/cachelevels=1:2k
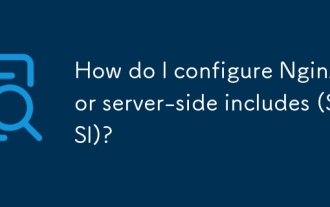
The article discusses configuring Nginx for server-side includes (SSI), performance implications, using SSI for dynamic content, and troubleshooting common SSI issues in Nginx.Word count: 159
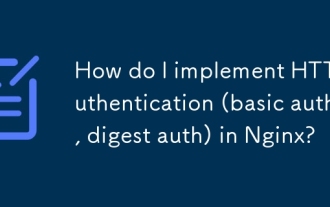
The article discusses implementing HTTP authentication in Nginx using basic and digest methods, detailing setup steps and security implications. It also covers using authentication realms for user management and suggests combining authentication meth
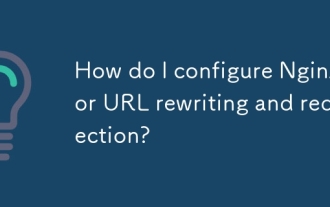
The article discusses configuring Nginx for URL rewriting and redirection, detailing steps and best practices. It addresses common mistakes and testing methods to ensure effective URL management.
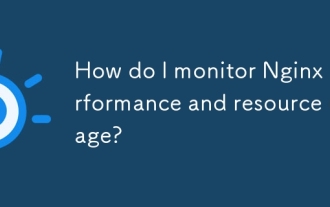
The article discusses monitoring and optimizing Nginx performance, focusing on using tools like Nginx's status page, system-level monitoring, and third-party solutions like Prometheus and Grafana. It emphasizes best practices for performance optimiza
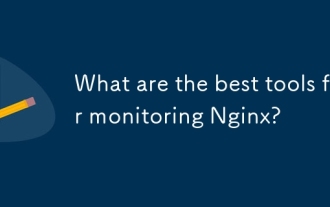
The article discusses top Nginx monitoring tools like Datadog, New Relic, and NGINX Amplify, focusing on their features for real-time monitoring, alerting, and detailed metrics to enhance server performance.
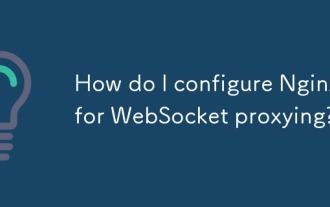
Article discusses configuring Nginx for WebSocket proxying, detailing necessary settings and troubleshooting steps for successful WebSocket connections.(159 characters)

The article details how to configure Gzip compression in Nginx, its performance benefits, and verification methods. Main issue: optimizing web server performance through compression.[159 characters]
