


How do I profile Java applications to identify performance bottlenecks?
How to Profile Java Applications to Identify Performance Bottlenecks
Profiling a Java application involves systematically measuring its performance characteristics to identify bottlenecks. This process typically involves instrumenting the application to track various metrics, such as CPU usage, memory allocation, garbage collection pauses, and I/O operations. The goal is to pinpoint specific code sections or operations that consume excessive resources, leading to slowdowns or performance degradation. Here's a step-by-step approach:
- Define Your Objectives: Before starting, clearly define what aspects of performance you want to analyze. Are you concerned about overall response time, CPU utilization, memory consumption, or specific operations? This will guide your choice of profiling tools and metrics.
- Choose a Profiling Tool: Select a suitable profiling tool (discussed in the next section). Consider factors like the type of profiling (sampling vs. instrumentation), the level of detail required, and your familiarity with the tool.
- Instrument Your Application: Most profiling tools require some level of integration with your application. This might involve adding agents, configuring JVM options, or using annotations. Follow the instructions provided by your chosen tool.
- Run Your Application Under Profile: Execute your application under the control of the profiling tool, ensuring you reproduce the performance issue you're investigating. The duration of the profiling session should be long enough to capture representative performance data.
- Analyze the Results: Once the profiling session is complete, review the generated reports. Look for areas with high CPU consumption, frequent garbage collection pauses, excessive memory allocation, or slow I/O operations. These are potential bottlenecks.
- Iterate and Optimize: Based on the profiling results, identify and address the bottlenecks. This might involve code optimization, algorithm improvements, database query tuning, or using more efficient data structures. Re-profile your application after each optimization to verify the improvements.
Best Tools for Profiling Java Applications
Several excellent tools are available for profiling Java applications, each with its strengths and weaknesses:
- Java VisualVM: This is a built-in tool in the JDK, making it readily accessible. It offers basic profiling capabilities, including CPU profiling, memory profiling, and thread monitoring. Its strength is its ease of use and accessibility; however, its capabilities are relatively limited compared to more advanced tools.
- JProfiler: A commercial tool offering comprehensive profiling features. It excels in its detailed analysis of CPU, memory, and thread activity. Its strength lies in its powerful visualization and analysis capabilities, but it comes with a cost.
- YourKit Java Profiler: Another commercial profiler known for its excellent performance and detailed analysis. It supports various profiling methods and provides insightful visualizations. Similar to JProfiler, its strength is its depth of analysis but it also comes with a price tag.
- Eclipse Memory Analyzer (MAT): Specialized for heap memory analysis. It's particularly useful for diagnosing memory leaks and identifying large objects consuming excessive memory. Its strength is its focus on memory analysis, but it doesn't provide comprehensive CPU or thread profiling.
- Async Profiler: A sampling profiler that's very low-overhead and ideal for production environments. It can provide insights into CPU usage, contention points, and other performance characteristics without significantly impacting the application's performance. Its strength is its low overhead and suitability for production.
Interpreting Java Application Profiling Results
Interpreting profiling results requires careful analysis. Focus on these key areas:
- High CPU Usage: Identify methods or code sections consuming a disproportionately large percentage of CPU time. These are prime candidates for optimization. Look for algorithmic inefficiencies or excessive computations.
- High Memory Consumption: Analyze memory allocation patterns. Look for memory leaks (objects that are no longer needed but are not garbage collected) or excessive object creation. Use tools like MAT to pinpoint the sources of memory leaks.
- Long Garbage Collection Pauses: Frequent or lengthy garbage collection pauses indicate inefficiencies in memory management. This might be due to excessive object creation, large objects, or inefficient garbage collection settings.
- I/O Bottlenecks: Identify slow I/O operations, such as database queries or network requests. Optimize database queries, use connection pooling, and consider asynchronous I/O techniques.
- Thread Contention: Analyze thread activity to identify areas with excessive contention (threads waiting for resources). This can lead to performance degradation. Consider using thread pools or other concurrency control mechanisms.
Use the profiler's visualization tools to identify patterns and relationships between different metrics. Correlate high CPU usage with specific methods, memory allocation with object creation, and I/O operations with network or database activity.
Common Performance Bottlenecks and Proactive Avoidance
Several common performance bottlenecks plague Java applications:
- Inefficient Algorithms: Using inefficient algorithms can lead to significant performance issues. Choose appropriate algorithms based on the problem's complexity and data size. Consider using optimized data structures like HashMaps or Trees instead of less efficient ones.
- Poor Database Design and Queries: Inefficient database queries can significantly impact performance. Optimize database queries, use appropriate indexes, and avoid unnecessary data retrieval.
- Unnecessary Object Creation: Excessive object creation can lead to increased garbage collection overhead. Reuse objects whenever possible and avoid creating unnecessary temporary objects.
- Inadequate Thread Management: Poor thread management can lead to contention and deadlocks. Use thread pools to manage threads effectively and implement proper synchronization mechanisms to avoid deadlocks.
- Memory Leaks: Memory leaks occur when objects are no longer needed but are not garbage collected. This leads to increased memory consumption and eventually to OutOfMemoryErrors. Use memory profilers to identify and fix memory leaks.
- Unoptimized I/O Operations: Slow I/O operations, especially network or database requests, can significantly impact performance. Use connection pooling, asynchronous I/O, and optimize network communication.
Proactive avoidance involves careful design and coding practices, regular performance testing, and using appropriate profiling tools to identify and address potential bottlenecks early in the development cycle. Regular code reviews and the use of static analysis tools can also help prevent common performance pitfalls.
The above is the detailed content of How do I profile Java applications to identify performance bottlenecks?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics




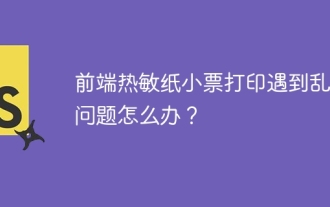
Frequently Asked Questions and Solutions for Front-end Thermal Paper Ticket Printing In Front-end Development, Ticket Printing is a common requirement. However, many developers are implementing...
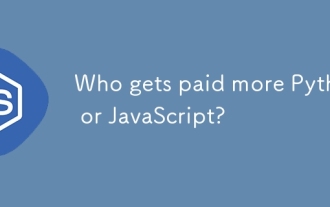
There is no absolute salary for Python and JavaScript developers, depending on skills and industry needs. 1. Python may be paid more in data science and machine learning. 2. JavaScript has great demand in front-end and full-stack development, and its salary is also considerable. 3. Influencing factors include experience, geographical location, company size and specific skills.
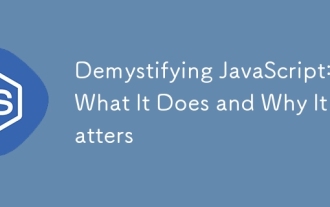
JavaScript is the cornerstone of modern web development, and its main functions include event-driven programming, dynamic content generation and asynchronous programming. 1) Event-driven programming allows web pages to change dynamically according to user operations. 2) Dynamic content generation allows page content to be adjusted according to conditions. 3) Asynchronous programming ensures that the user interface is not blocked. JavaScript is widely used in web interaction, single-page application and server-side development, greatly improving the flexibility of user experience and cross-platform development.
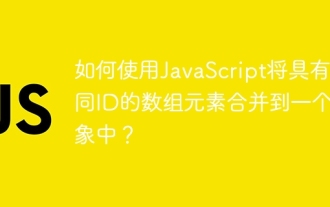
How to merge array elements with the same ID into one object in JavaScript? When processing data, we often encounter the need to have the same ID...
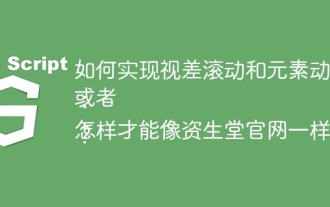
Discussion on the realization of parallax scrolling and element animation effects in this article will explore how to achieve similar to Shiseido official website (https://www.shiseido.co.jp/sb/wonderland/)...
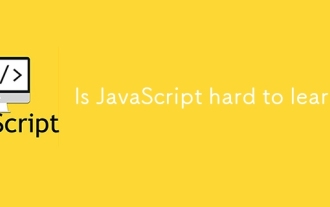
Learning JavaScript is not difficult, but it is challenging. 1) Understand basic concepts such as variables, data types, functions, etc. 2) Master asynchronous programming and implement it through event loops. 3) Use DOM operations and Promise to handle asynchronous requests. 4) Avoid common mistakes and use debugging techniques. 5) Optimize performance and follow best practices.
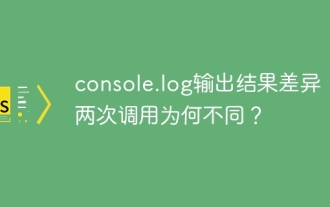
In-depth discussion of the root causes of the difference in console.log output. This article will analyze the differences in the output results of console.log function in a piece of code and explain the reasons behind it. �...
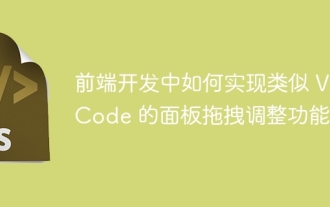
Explore the implementation of panel drag and drop adjustment function similar to VSCode in the front-end. In front-end development, how to implement VSCode similar to VSCode...
